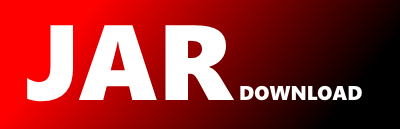
io.mosip.authentication.common.service.integration.MasterDataManager Maven / Gradle / Ivy
package io.mosip.authentication.common.service.integration;
import java.util.AbstractMap;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.jayway.jsonpath.JsonPath;
import io.mosip.authentication.common.service.cache.MasterDataCache;
import io.mosip.authentication.core.constant.IdAuthCommonConstants;
import io.mosip.authentication.core.constant.IdAuthenticationErrorConstants;
import io.mosip.authentication.core.constant.RestServicesConstants;
import io.mosip.authentication.core.exception.IDDataValidationException;
import io.mosip.authentication.core.exception.IdAuthenticationBusinessException;
import io.mosip.authentication.core.logger.IdaLogger;
import io.mosip.authentication.core.util.LanguageComparator;
import io.mosip.kernel.core.logger.spi.Logger;
/**
* MasterDataManager.
*
* @author Dinesh Karuppiah.T
* @author Manoj SP
* @author Nagarjuna
*/
@Component
public class MasterDataManager {
/** The Constant TITLE_NAME_JSON_PATH. */
private static final String TITLE_NAME_JSON_PATH = "$.response.titleList[?(@.langCode=='%s')].titleName";
/** The Constant LANG_CODE_JSON_PATH. */
private static final String LANG_CODE_JSON_PATH = "$.response.titleList.*.langCode";
/** The Constant LANG_CODE. */
private static final String LANG_CODE = "langCode";
/** The Constant TEMPLATE_TYPE_CODE. */
private static final String TEMPLATE_TYPE_CODE = "templateTypeCode";
/** The Constant RESPONSE. */
private static final String RESPONSE = "response";
/** The Constant IS_ACTIVE. */
private static final String IS_ACTIVE = "isActive";
/** The Constant FILE_TEXT. */
private static final String FILE_TEXT = "fileText";
/** The Constant TEMPLATES. */
private static final String TEMPLATES = "templates";
/** The Constant NAME_PLACEHOLDER. */
private static final String NAME_PLACEHOLDER = "$name";
/** IdTemplate Manager Logger. */
private static Logger logger = IdaLogger.getLogger(MasterDataManager.class);
/** The master data cache. */
@Autowired
private MasterDataCache masterDataCache;
/**
* Fetch master data for provided languages.
*
* @param type the type
* @param params the params
* @param masterDataListName the master data list name
* @param keyAttribute the key attribute
* @param valueAttribute the value attribute
* @return the map
* @throws IdAuthenticationBusinessException the id authentication business
* exception
*/
@SuppressWarnings("unchecked")
Map> fetchMasterData(RestServicesConstants type, Map params,
String masterDataListName, String keyAttribute, String valueAttribute)
throws IdAuthenticationBusinessException {
try {
Map response = masterDataCache.getMasterDataTemplate(params.get(TEMPLATE_TYPE_CODE));
Map>> fetchResponse;
if (response.get(RESPONSE) instanceof Map) {
fetchResponse = (Map>>) response.get(RESPONSE);
} else {
fetchResponse = Collections.emptyMap();
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy