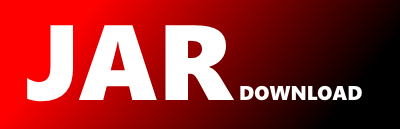
io.mosip.pms.common.util.RestUtil Maven / Gradle / Ivy
package io.mosip.pms.common.util;
import java.io.IOException;
import java.net.URI;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.cert.X509Certificate;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.net.ssl.SSLContext;
import org.apache.http.Header;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.TrustStrategy;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.client.HttpComponentsClientHttpRequestFactory;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.util.UriComponents;
import org.springframework.web.util.UriComponentsBuilder;
import com.google.gson.Gson;
import io.mosip.kernel.core.util.DateUtils;
import io.mosip.kernel.core.util.StringUtils;
import io.mosip.kernel.core.util.TokenHandlerUtil;
import io.mosip.pms.common.constant.ApiAccessibleExceptionConstant;
import io.mosip.pms.common.dto.Metadata;
import io.mosip.pms.common.dto.SecretKeyRequest;
import io.mosip.pms.common.dto.TokenRequestDTO;
import io.mosip.pms.common.exception.ApiAccessibleException;
@Component
public class RestUtil {
@Autowired
private Environment environment;
private static final String AUTHORIZATION = "Authorization=";
@SuppressWarnings("unchecked")
public T postApi(String apiUrl, List pathsegments, String queryParamName, String queryParamValue,
MediaType mediaType, Object requestType, Class> responseClass) {
T result = null;
UriComponentsBuilder builder = null;
if (apiUrl != null)
builder = UriComponentsBuilder.fromUriString(apiUrl);
if (builder != null) {
if (!((pathsegments == null) || (pathsegments.isEmpty()))) {
for (String segment : pathsegments) {
if (!((segment == null) || (("").equals(segment)))) {
builder.pathSegment(segment);
}
}
}
if (!((queryParamName == null) || (("").equals(queryParamName)))) {
String[] queryParamNameArr = queryParamName.split(",");
String[] queryParamValueArr = queryParamValue.split(",");
for (int i = 0; i < queryParamNameArr.length; i++) {
builder.queryParam(queryParamNameArr[i], queryParamValueArr[i]);
}
}
RestTemplate restTemplate;
try {
restTemplate = getRestTemplate();
result = (T) restTemplate.postForObject(builder.toUriString(), setRequestHeader(requestType, mediaType),
responseClass);
} catch (Exception e) {
throw new ApiAccessibleException(
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorCode(),
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorMessage() + apiUrl);
}
}
return result;
}
@SuppressWarnings("unchecked")
public T getApi(String apiName, List pathsegments, String queryParamName, String queryParamValue,
Class> responseType) {
String apiHostIpPort = environment.getProperty(apiName);
T result = null;
UriComponentsBuilder builder = null;
UriComponents uriComponents = null;
if (apiHostIpPort != null) {
builder = UriComponentsBuilder.fromUriString(apiHostIpPort);
if (!((pathsegments == null) || (pathsegments.isEmpty()))) {
for (String segment : pathsegments) {
if (!((segment == null) || (("").equals(segment)))) {
builder.pathSegment(segment);
}
}
}
if (!((queryParamName == null) || (("").equals(queryParamName)))) {
String[] queryParamNameArr = queryParamName.split(",");
String[] queryParamValueArr = queryParamValue.split(",");
for (int i = 0; i < queryParamNameArr.length; i++) {
builder.queryParam(queryParamNameArr[i], queryParamValueArr[i]);
}
}
uriComponents = builder.build(false).encode();
RestTemplate restTemplate;
try {
restTemplate = getRestTemplate();
result = (T) restTemplate
.exchange(uriComponents.toUri(), HttpMethod.GET, setRequestHeader(null, null), responseType)
.getBody();
} catch (Exception e) {
throw new ApiAccessibleException(
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorCode(),
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorMessage() + apiName);
}
}
return result;
}
@SuppressWarnings("unchecked")
public T getApi(String apiUrl, Map pathsegments, Class> responseType) {
T result = null;
UriComponentsBuilder builder = null;
if (apiUrl != null) {
builder = UriComponentsBuilder.fromUriString(apiUrl);
URI urlWithPath = builder.build(pathsegments);
RestTemplate restTemplate;
try {
restTemplate = getRestTemplate();
result = (T) restTemplate
.exchange(urlWithPath, HttpMethod.GET, setRequestHeader(null, null), responseType).getBody();
} catch (Exception e) {
throw new ApiAccessibleException(
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorCode(),
ApiAccessibleExceptionConstant.API_NOT_ACCESSIBLE_EXCEPTION.getErrorMessage() + apiUrl);
}
}
return result;
}
public RestTemplate getRestTemplate() throws KeyManagementException, NoSuchAlgorithmException, KeyStoreException {
TrustStrategy acceptingTrustStrategy = (X509Certificate[] chain, String authType) -> true;
SSLContext sslContext = org.apache.http.ssl.SSLContexts.custom().loadTrustMaterial(null, acceptingTrustStrategy)
.build();
SSLConnectionSocketFactory csf = new SSLConnectionSocketFactory(sslContext);
CloseableHttpClient httpClient = HttpClients.custom().setSSLSocketFactory(csf).build();
HttpComponentsClientHttpRequestFactory requestFactory = new HttpComponentsClientHttpRequestFactory();
requestFactory.setHttpClient(httpClient);
return new RestTemplate(requestFactory);
}
@SuppressWarnings("unchecked")
private HttpEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy