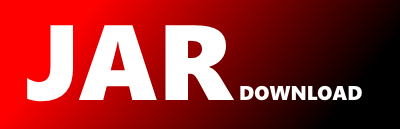
io.mosip.pms.common.util.NotificationUtil Maven / Gradle / Ivy
package io.mosip.pms.common.util;
import java.io.IOException;
import java.time.LocalDateTime;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.multipart.MultipartFile;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.mosip.kernel.core.logger.spi.Logger;
import io.mosip.kernel.core.util.DateUtils;
import io.mosip.pms.common.constant.ApiAccessibleExceptionConstant;
import io.mosip.pms.common.exception.ApiAccessibleException;
import io.mosip.pms.common.response.dto.NotificationDto;
import io.mosip.pms.common.response.dto.NotificationResponseDto;
import io.mosip.pms.common.response.dto.ResponseWrapper;
/**
*
* @author Nagarjuna
*
*/
@Component
public class NotificationUtil {
private Logger log = PMSLogger.getLogger(NotificationUtil.class);
@Value("${emailResourse.url}")
private String emailResourseUrl;
@Autowired
RestUtil restUtil;
@Autowired
private ObjectMapper mapper;
/**
* Calls the email notifier api to send email notifications
*
* @param notificationDto
* @param file
* @param subject
* @param body
* @return
* @throws IOException
*/
public ResponseWrapper emailNotification(NotificationDto notificationDto,
MultipartFile file, String subject, String body) throws IOException {
log.info("In emailNotification method of NotificationUtil service");
HttpEntity doc = null;
MultiValueMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy