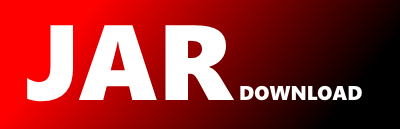
io.mosip.preregistration.batchjob.repository.AvailabilityRepository Maven / Gradle / Ivy
/*
* Copyright
*
*/
package io.mosip.preregistration.batchjob.repository;
import java.time.LocalDate;
import java.time.LocalTime;
import java.util.List;
import javax.transaction.Transactional;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.query.Param;
import org.springframework.stereotype.Repository;
import io.mosip.kernel.core.dataaccess.spi.repository.BaseRepository;
import io.mosip.preregistration.batchjob.entity.AvailibityEntity;
/**
* This repository interface is used to define the JPA methods for Booking application.
*
* @author Kishan Rathore
* @author Jagadishwari
* @author Ravi C. Balaji
* @since 1.0.0
*
*/
@Repository("availabilityRepository")
@Transactional
public interface AvailabilityRepository extends BaseRepository {
/**
* @param Registration center id
* @param Registration date
* @return List AvailibityEntity based registration id and registration date.
*/
public List findByRegcntrIdAndRegDateOrderByFromTimeAsc(String regcntrId, LocalDate regDate);
/**
* @param regcntrId
* @param fromDate
* @param toDate
* @return List of LocalDate based on date
*/
public List findDate(@Param("regcntrId") String regcntrId, @Param("fromDate") LocalDate fromDate,
@Param("toDate") LocalDate toDate);
/**
* @param slot_from_time
* @param slot_to_time
* @param reg_date
* @param regcntr_id
* @return Availability entity
*/
public AvailibityEntity findByFromTimeAndToTimeAndRegDateAndRegcntrId(
@Param("slot_from_time") LocalTime slotFromTime, @Param("slot_to_time") LocalTime slotToTime,
@Param("availability_date") LocalDate regDate, @Param("regcntr_id") String regcntrd);
/**
*
* @param regDate
* @return list of available date
*/
@Query("SELECT DISTINCT e.regDate FROM AvailibityEntity e WHERE e.regDate>= ?1")
public List findAvaialableDate(LocalDate regDate);
public List findByRegcntrId(String regCenterId);
/**
*
* @param regDate
* @return list of available date
*/
@Query("SELECT DISTINCT e.regcntrId FROM AvailibityEntity e WHERE e.regDate>= ?1")
public List findAvaialableRegCenter(LocalDate regDate);
/**
*
* @param regDate
* @param regcntrId
* @return list of available date
*/
@Query("SELECT DISTINCT e.regDate FROM AvailibityEntity e WHERE e.regDate>= ?1 and e.regcntrId=?2")
public List findAvaialableDate(LocalDate regDate, String regcntrId);
/**
*
* @param regDate
* @param regcntrId
* @return list of AvailibityEntity
*/
@Query("SELECT DISTINCT e FROM AvailibityEntity e WHERE e.regDate= ?1 and e.regcntrId=?2 order by e.fromTime ")
public List findAvaialableSlots(LocalDate regDate, String regcntrId);
/**
*
* @param regDate
* @param regcntrId
* @return deleted number of slots
*/
public int deleteByRegcntrIdAndRegDate( String regcntrId ,LocalDate regDate);
/**
*
* @param regDate
* @param regcntrId
* @return deleted number of slots
*/
public int deleteByRegcntrIdAndRegDateAndFromTimeBetween( String regcntrId ,LocalDate regDate, LocalTime startTime,LocalTime endTime);
/**
*
* @param regDate
* @return list of regcntrId
*/
public int deleteByRegcntrIdAndRegDateGreaterThanEqual(String regcntrId, LocalDate regDate);
/**
*
* @param regDate
* @param regcntrId
* @return deleted number of slots
*/
public int deleteByRegcntrIdAndRegDateAndFromTimeAndToTime( String regcntrId ,LocalDate regDate, LocalTime startTime,LocalTime endTime);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy