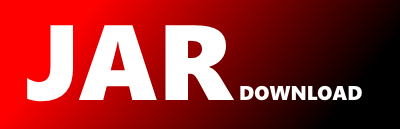
io.mosip.registration.controller.vo.PacketStatusVO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of registration-client Show documentation
Show all versions of registration-client Show documentation
Maven project of MOSIP Registration UI
package io.mosip.registration.controller.vo;
import javafx.beans.property.BooleanProperty;
import javafx.beans.property.SimpleBooleanProperty;
import javafx.beans.property.SimpleStringProperty;
public class PacketStatusVO {
private SimpleStringProperty fileName;
private SimpleStringProperty packetId;
private SimpleStringProperty packetClientStatus;
private SimpleStringProperty packetServerStatus;
private BooleanProperty status = new SimpleBooleanProperty(false);
private SimpleStringProperty packetPath;
private SimpleStringProperty uploadStatus;
private SimpleStringProperty clientStatusComments;
private SimpleStringProperty packetStatus;
private SimpleStringProperty supervisorStatus;
private SimpleStringProperty supervisorComments;
private SimpleStringProperty createdTime;
private SimpleStringProperty slno;
private SimpleStringProperty name;
private SimpleStringProperty phone;
private SimpleStringProperty email;
private SimpleStringProperty userId;
/**
* @return the supervisorStatus
*/
public String getSupervisorStatus() {
return supervisorStatus.get();
}
/**
* @param supervisorStatus the supervisorStatus to set
*/
public void setSupervisorStatus(String supervisorStatus) {
this.supervisorStatus = new SimpleStringProperty(supervisorStatus);
}
/**
* @return the supervisorComments
*/
public String getSupervisorComments() {
return supervisorComments.get();
}
/**
* @param supervisorComments the supervisorComments to set
*/
public void setSupervisorComments(String supervisorComments) {
this.supervisorComments = new SimpleStringProperty(supervisorComments);
}
public String getFileName() {
return fileName.get();
}
public void setFileName(String fileName) {
this.fileName = new SimpleStringProperty(fileName);
}
public String getPacketId() {
return packetId.get();
}
public void setPacketId(String packetId) {
this.packetId = new SimpleStringProperty(packetId);
}
public String getPacketClientStatus() {
return packetClientStatus.get();
}
public void setPacketClientStatus(String packetClientStatus) {
this.packetClientStatus = new SimpleStringProperty(packetClientStatus);
}
public String getPacketServerStatus() {
return packetServerStatus.get();
}
public void setPacketServerStatus(String packetServerStatus) {
this.packetServerStatus = new SimpleStringProperty(packetServerStatus);
}
public String getPacketPath() {
return packetPath.get();
}
public void setPacketPath(String packetPath) {
this.packetPath = new SimpleStringProperty(packetPath);
}
public String getUploadStatus() {
return uploadStatus.get();
}
public void setUploadStatus(String uploadStatus) {
this.uploadStatus = new SimpleStringProperty(uploadStatus);
}
public String getClientStatusComments() {
return clientStatusComments.get();
}
public void setClientStatusComments(String clientStatusComments) {
this.clientStatusComments = new SimpleStringProperty(clientStatusComments);
}
public Boolean getStatus() {
return status.get();
}
public BooleanProperty selectedProperty() {
return status;
}
public void setStatus(Boolean status) {
this.status.set(status);
}
public String getPacketStatus() {
return packetStatus.get();
}
public void setPacketStatus(String packetStatus) {
this.packetStatus = new SimpleStringProperty(packetStatus);
}
public String getCreatedTime() {
return createdTime.get();
}
public void setCreatedTime(String createdTime) {
this.createdTime = new SimpleStringProperty(createdTime);
}
public String getSlno() {
return slno.get();
}
public void setSlno(String slno) {
this.slno = new SimpleStringProperty(slno);
}
public String getName() {
return name == null ? null : name.get();
}
public void setName(String name) {
this.name = new SimpleStringProperty(name);
}
public String getPhone() {
return phone == null ? null : phone.get();
}
public void setPhone(String phone) {
this.phone = new SimpleStringProperty(phone);
}
public String getEmail() {
return email == null ? null : email.get();
}
public void setEmail(String email) {
this.email = new SimpleStringProperty(email);
}
public String getUserId() {
return userId == null ? null : userId.get();
}
public void setUserId(String userId) {
this.userId = new SimpleStringProperty(userId);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy