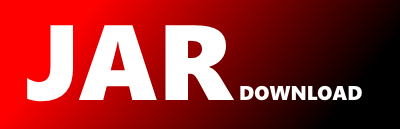
io.mosip.registration.util.control.impl.DropDownFxControl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of registration-client Show documentation
Show all versions of registration-client Show documentation
Maven project of MOSIP Registration UI
package io.mosip.registration.util.control.impl;
import java.util.*;
import java.util.Map.Entry;
import io.mosip.registration.controller.ClientApplication;
import io.mosip.registration.dao.MasterSyncDao;
import org.springframework.context.ApplicationContext;
import io.mosip.registration.dto.mastersync.GenericDto;
import io.mosip.commons.packet.dto.packet.SimpleDto;
import io.mosip.kernel.core.logger.spi.Logger;
import io.mosip.registration.config.AppConfig;
import io.mosip.registration.constants.RegistrationConstants;
import io.mosip.registration.controller.FXUtils;
import io.mosip.registration.controller.GenericController;
import io.mosip.registration.controller.Initialization;
import io.mosip.registration.controller.reg.Validations;
import io.mosip.registration.dto.schema.UiFieldDTO;
import io.mosip.registration.entity.Location;
import io.mosip.registration.service.sync.MasterSyncService;
import io.mosip.registration.util.common.ComboBoxAutoComplete;
import io.mosip.registration.util.common.DemographicChangeActionHandler;
import io.mosip.registration.util.control.FxControl;
import javafx.scene.Node;
import javafx.scene.control.ComboBox;
import javafx.scene.control.Label;
import javafx.scene.layout.Pane;
import javafx.scene.layout.VBox;
import javafx.util.StringConverter;
import org.springframework.util.Assert;
public class DropDownFxControl extends FxControl {
/**
* Instance of {@link Logger}
*/
private static final Logger LOGGER = AppConfig.getLogger(DropDownFxControl.class);
private static final String loggerClassName = "DropDownFxControl";
private int hierarchyLevel;
private Validations validation;
private DemographicChangeActionHandler demographicChangeActionHandler;
private MasterSyncService masterSyncService;
private MasterSyncDao masterSyncDao;
public DropDownFxControl() {
ApplicationContext applicationContext = ClientApplication.getApplicationContext();
validation = applicationContext.getBean(Validations.class);
demographicChangeActionHandler = applicationContext.getBean(DemographicChangeActionHandler.class);
masterSyncService = applicationContext.getBean(MasterSyncService.class);
masterSyncDao = applicationContext.getBean(MasterSyncDao.class);
}
@Override
public FxControl build(UiFieldDTO uiFieldDTO) {
this.uiFieldDTO = uiFieldDTO;
this.control = this;
this.node = create(uiFieldDTO, getRegistrationDTo().getSelectedLanguagesByApplicant().get(0));
//As subType in UI Spec is defined in any lang we find the langCode to fill initial dropdown
String subTypeLangCode = getSubTypeLangCode(uiFieldDTO.getSubType());
if(subTypeLangCode != null) {
TreeMap groupFields = GenericController.currentHierarchyMap.getOrDefault(uiFieldDTO.getGroup(), new TreeMap<>());
for (Entry entry : GenericController.hierarchyLevels.get(subTypeLangCode).entrySet()) {
if (entry.getValue().equals(uiFieldDTO.getSubType())) {
this.hierarchyLevel = entry.getKey();
groupFields.put(entry.getKey(), uiFieldDTO.getId());
GenericController.currentHierarchyMap.put(uiFieldDTO.getGroup(), groupFields);
break;
}
}
}
Map data = new LinkedHashMap<>();
data.put(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0),
getPossibleValues(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0)));
//clears & refills items
fillData(data);
return this.control;
}
private String getSubTypeLangCode(String subType) {
for( String langCode : GenericController.hierarchyLevels.keySet()) {
if(GenericController.hierarchyLevels.get(langCode).containsValue(subType))
return langCode;
}
return null;
}
private VBox create(UiFieldDTO uiFieldDTO, String langCode) {
String fieldName = uiFieldDTO.getId();
/** Container holds title, fields and validation message elements */
VBox simpleTypeVBox = new VBox();
//simpleTypeVBox.setPrefWidth(200);
//simpleTypeVBox.setPrefHeight(95);
simpleTypeVBox.setSpacing(5);
simpleTypeVBox.setId(fieldName + RegistrationConstants.VBOX);
/** Title label */
Label fieldTitle = getLabel(uiFieldDTO.getId() + RegistrationConstants.LABEL, "",
RegistrationConstants.DEMOGRAPHIC_FIELD_LABEL, true, simpleTypeVBox.getWidth());
simpleTypeVBox.getChildren().add(fieldTitle);
List labels = new ArrayList<>();
getRegistrationDTo().getSelectedLanguagesByApplicant().forEach(lCode -> {
labels.add(this.uiFieldDTO.getLabel().get(lCode));
});
String titleText = String.join(RegistrationConstants.SLASH, labels) + getMandatorySuffix(uiFieldDTO);
ComboBox comboBox = getComboBox(fieldName, titleText, RegistrationConstants.DOC_COMBO_BOX,
simpleTypeVBox.getPrefWidth(), false);
simpleTypeVBox.getChildren().add(comboBox);
comboBox.setOnMouseExited(event -> {
getField(uiFieldDTO.getId() + RegistrationConstants.MESSAGE).setVisible(false);
if(comboBox.getTooltip()!=null) {
comboBox.getTooltip().hide();
}
});
comboBox.setOnMouseEntered((event -> {
getField(uiFieldDTO.getId() + RegistrationConstants.MESSAGE).setVisible(true);
}));
setListener(comboBox);
fieldTitle.setText(titleText);
Label messageLabel = getLabel(uiFieldDTO.getId() + RegistrationConstants.MESSAGE, null,
RegistrationConstants.DEMOGRAPHIC_FIELD_LABEL, false, simpleTypeVBox.getPrefWidth());
messageLabel.setMaxWidth(200);
simpleTypeVBox.getChildren().add(messageLabel);
changeNodeOrientation(simpleTypeVBox, langCode);
return simpleTypeVBox;
}
public List getPossibleValues(String langCode) {
boolean isHierarchical = false;
String fieldSubType = uiFieldDTO.getSubType();
if(GenericController.currentHierarchyMap.containsKey(uiFieldDTO.getGroup())) {
isHierarchical = true;
Entry parentEntry = GenericController.currentHierarchyMap.get(uiFieldDTO.getGroup())
.lowerEntry(this.hierarchyLevel);
if(parentEntry == null) { //first parent
parentEntry = GenericController.hierarchyLevels.get(langCode).lowerEntry(this.hierarchyLevel);
Assert.notNull(parentEntry);
List locations = masterSyncDao.getLocationDetails(parentEntry.getValue(), langCode);
fieldSubType = locations != null && !locations.isEmpty() ? locations.get(0).getCode() : null;
}
else {
FxControl fxControl = GenericController.getFxControlMap().get(parentEntry.getValue());
Node comboBox = getField(fxControl.getNode(), parentEntry.getValue());
GenericDto selectedItem = comboBox != null ?
((ComboBox) comboBox).getSelectionModel().getSelectedItem() : null;
fieldSubType = selectedItem != null ? selectedItem.getCode() : null;
if(fieldSubType == null)
return Collections.EMPTY_LIST;
}
}
return masterSyncService.getFieldValues(fieldSubType, langCode, isHierarchical);
}
private ComboBox getComboBox(String id, String titleText, String stycleClass, double prefWidth,
boolean isDisable) {
ComboBox field = new ComboBox();
StringConverter uiRenderForComboBox = FXUtils.getInstance().getStringConverterForComboBox();
field.setId(id);
// field.setPrefWidth(prefWidth);
//field.setPromptText(titleText);
field.setDisable(isDisable);
field.getStyleClass().add(RegistrationConstants.DEMOGRAPHIC_COMBOBOX);
field.setConverter((StringConverter) uiRenderForComboBox);
return field;
}
@Override
public void setData(Object data) {
ComboBox appComboBox = (ComboBox) getField(uiFieldDTO.getId());
if(appComboBox.getSelectionModel().getSelectedItem() == null) {
return;
}
String selectedCode = appComboBox.getSelectionModel().getSelectedItem().getCode();
switch (this.uiFieldDTO.getType()) {
case RegistrationConstants.SIMPLE_TYPE:
List values = new ArrayList();
for (String langCode : getRegistrationDTo().getSelectedLanguagesByApplicant()) {
Optional result = getPossibleValues(langCode).stream()
.filter(b -> b.getCode().equals(selectedCode)).findFirst();
if (result.isPresent()) {
SimpleDto simpleDto = new SimpleDto(langCode, result.get().getName());
values.add(simpleDto);
}
}
getRegistrationDTo().addDemographicField(uiFieldDTO.getId(), values);
getRegistrationDTo().SELECTED_CODES.put(uiFieldDTO.getId()+"Code", selectedCode);
break;
default:
Optional result = getPossibleValues(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0)).stream()
.filter(b -> b.getCode().equals(selectedCode)).findFirst();
if (result.isPresent()) {
getRegistrationDTo().addDemographicField(uiFieldDTO.getId(), result.get().getName());
getRegistrationDTo().SELECTED_CODES.put(uiFieldDTO.getId()+"Code", selectedCode);
}
break;
}
}
@Override
public Object getData() {
return getRegistrationDTo().getDemographics().get(uiFieldDTO.getId());
}
@Override
public boolean isValid() {
ComboBox appComboBox = (ComboBox) getField(uiFieldDTO.getId());
boolean isValid = appComboBox != null && appComboBox.getSelectionModel().getSelectedItem() != null;
if (appComboBox != null) {
appComboBox.getStyleClass().removeIf((s) -> {
return s.equals("demographicComboboxFocused");
});
if(!isValid) {
appComboBox.getStyleClass().add("demographicComboboxFocused");
}
}
return isValid;
}
@Override
public boolean isEmpty() {
ComboBox appComboBox = (ComboBox) getField(uiFieldDTO.getId());
return appComboBox == null || appComboBox.getSelectionModel().getSelectedItem() == null;
}
@Override
public void setListener(Node node) {
ComboBox fieldComboBox = (ComboBox) node;
fieldComboBox.getSelectionModel().selectedItemProperty().addListener((options, oldValue, newValue) -> {
displayFieldLabel();
if (isValid()) {
List toolTipText = new ArrayList<>();
String selectedCode = fieldComboBox.getSelectionModel().getSelectedItem().getCode();
for (String langCode : getRegistrationDTo().getSelectedLanguagesByApplicant()) {
Optional result = getPossibleValues(langCode).stream()
.filter(b -> b.getCode().equals(selectedCode)).findFirst();
if (result.isPresent()) {
toolTipText.add(result.get().getName());
}
}
Label messageLabel = (Label) getField(uiFieldDTO.getId() + RegistrationConstants.MESSAGE);
messageLabel.setText(String.join(RegistrationConstants.SLASH, toolTipText));
setData(null);
refreshNextHierarchicalFxControls();
demographicChangeActionHandler.actionHandle((Pane) getNode(), node.getId(), uiFieldDTO.getChangeAction());
// Group level visibility listeners
refreshFields();
}
});
}
private void refreshNextHierarchicalFxControls() {
if(GenericController.currentHierarchyMap.containsKey(uiFieldDTO.getGroup())) {
Entry nextEntry = GenericController.currentHierarchyMap.get(uiFieldDTO.getGroup())
.higherEntry(this.hierarchyLevel);
while (nextEntry != null) {
FxControl fxControl = GenericController.getFxControlMap().get(nextEntry.getValue());
Map data = new LinkedHashMap<>();
data.put(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0),
fxControl.getPossibleValues(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0)));
//clears & refills items
fxControl.fillData(data);
nextEntry = GenericController.currentHierarchyMap.get(uiFieldDTO.getGroup())
.higherEntry(nextEntry.getKey());
}
}
}
private void displayFieldLabel() {
FXUtils.getInstance().toggleUIField((Pane) getNode(), uiFieldDTO.getId() + RegistrationConstants.LABEL,
true);
Label label = (Label) getField(uiFieldDTO.getId() + RegistrationConstants.LABEL);
label.getStyleClass().add("demoGraphicFieldLabelOnType");
label.getStyleClass().remove("demoGraphicFieldLabel");
FXUtils.getInstance().toggleUIField((Pane) getNode(), uiFieldDTO.getId() + RegistrationConstants.MESSAGE, false);
}
private Node getField(Node fieldParentNode, String id) {
return fieldParentNode.lookup(RegistrationConstants.HASH + id);
}
@Override
public void fillData(Object data) {
if (data != null) {
Map> val = (Map>) data;
List items = val.get(getRegistrationDTo().getSelectedLanguagesByApplicant().get(0));
if (items != null && !items.isEmpty()) {
setItems((ComboBox) getField(uiFieldDTO.getId()), items);
}
}
}
private void setItems(ComboBox comboBox, List val) {
if (comboBox != null && val != null && !val.isEmpty()) {
comboBox.getItems().clear();
comboBox.getItems().addAll(val);
new ComboBoxAutoComplete(comboBox);
comboBox.hide();
}
}
@Override
public void selectAndSet(Object data) {
ComboBox field = (ComboBox) getField(uiFieldDTO.getId());
if (data == null) {
field.getSelectionModel().clearSelection();
return;
}
if (data instanceof List) {
List list = (List) data;
selectItem(field, list.isEmpty() ? null : list.get(0).getValue());
} else if (data instanceof String) {
selectItem(field, (String) data);
}
}
private void selectItem(ComboBox field, String val) {
if (field != null && val != null && !val.isEmpty()) {
for (GenericDto genericDto : field.getItems()) {
if (genericDto.getCode().equals(val)) {
field.getSelectionModel().select(genericDto);
break;
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy