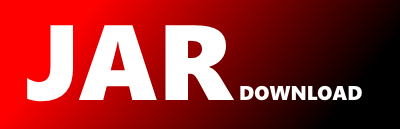
io.mosip.registration.dao.RegistrationDAO Maven / Gradle / Ivy
package io.mosip.registration.dao;
import java.sql.Timestamp;
import java.util.List;
import io.mosip.registration.dto.PacketStatusDTO;
import io.mosip.registration.dto.RegistrationDTO;
import io.mosip.registration.entity.Registration;
import io.mosip.registration.exception.RegBaseCheckedException;
import lombok.NonNull;
/**
* This class will be used to fetch/Add/Update details from the
* {@link Registration} and {@link RegistrationTransaction} while creating a
* registration packet the respective packet data will be stored tables. It will
* insert a record while creating a new packet. While performing EOD operation
* this will fetch the registration data and updates the respective operation
* related information to registration table. While performing the Sync and
* Upload operations it fetches the corresponding registrations and updates the
* registrations accordingly depending upon the status received.
*
* @author Balaji Sridharan
* @author Mahesh Kumar
* @author Saravanakumar Gnanaguru
* @since 1.0.0
*/
public interface RegistrationDAO {
/**
*
* Once the packet gets created and stored in the local this method will get
* called to save the record for that particular registration id.
*
*
*
* All the packet related details will be stored in the Registration entity and
* it will get saved
*
*
* @param zipFileName the name of the zip file with absolute path
* @param registrationDTO the {@link RegistrationDTO} of the individual
* @throws RegBaseCheckedException will be thrown if any exception occurs while
* saving {@link Registration}
*/
void save(String zipFileName, RegistrationDTO registrationDTO) throws RegBaseCheckedException;
/**
*
* Once the packet gets created the packet will gets approved by the supervisor
* and the same will be updated as either Approved or Rejected using this
* method.
*
*
*
* If Approved:
*
*
* The same will updated in the status comments
*
*
* If rejected:
*
*
* The rejection reason will be updated in the status comments
*
*
* @param registrationID the id of the {@link Registration} entity to be
* updated
* @param statusComments the status comments to be updated
* @param clientStatusCode the status to be updated
* @return the updated {@link Registration} entity
*/
Registration updateRegistration(String applicationId, String statusComments, String clientStatusCode);
/**
*
* It will retrieve registration records based on the status
*
*
* The records will be arranged in the ascending order of the created Date time
*
*
* @param status the status of the registration to be retrieved
* @return the list of {@link Registration} based on the given input status
*/
List getEnrollmentByStatus(String status);
/**
*
* This method is used to fetch the records in which the corresponding packets
* are ready to upload
*
*
* The records that are fetched here are based on the client status code and
* server status code
*
* Client Status Codes
*
* - Synced
* - Exported
*
* Server Status Code
*
* - Resend
*
*
* @param packetStatus the packet status
* @return the list of {@link Registration} based on status
*/
List getRegistrationByStatus(List packetStatus);
/**
*
* Once the Packet Upload is done to the Server the same needs to be updated in
* the DB and it will be done through this method.
*
*
* The packet upload count will get increased and updated in the Upload count
* column, incase if we tries to upload the same packet again.
*
*
* @param packetStatus - the {@link PacketStatusDTO} to be updated
* @return {@link Registration} entity
*/
Registration updateRegStatus(PacketStatusDTO packetStatus);
/**
*
* This method will fetch the records which are in
* Approved/Rejected/ReRegisterApproved
*
*
* The records will be fetched in the Ascending order of Update Timestamp
*
*
* @param statusCodes the status codes - Approved/Rejected/ReRegisterApproved
* @return List of {@link Registration} entities
*/
List getPacketsToBeSynched(List statusCodes);
/**
* This method is used to update the Packet sync status in the database.
*
* @param packet the packet
* @return the registration
*/
Registration updatePacketSyncStatus(PacketStatusDTO packet);
/**
* This method is used to get all the Re-Registration packets.
*
* @param status the status
* @return the all re registration packets
*/
List getAllReRegistrationPackets(String clientStatus, List serverStatus);
/**
* This method is used to get list of Registrations by passing list of registration id's.
*
* @param regIds id
* @return List of Registrations
*/
List get(List regIds);
/**
* This method is used to find list of registrations by CrDtimes and client status code.
*
* @param crDtimes the date upto packets to be deleted
* @param serverStatusCode status of resgistrationPacket
* @return list of registrations
*/
List get(Timestamp crDtimes, List serverStatusCodes);
/**
* This method is used to find list of registrations by server status code in.
*
* @param serveSstatusCodes the serve status codes
* @return the list
*/
List findByServerStatusCodeIn(List serveSstatusCodes);
/**
* This method is used to find list of registrations by server status code not in.
*
* @param serverStatusCodes the server status codes
* @return the list
*/
List findByServerStatusCodeNotIn(List serverStatusCodes);
/**
* This method is used to fetch the records for the packets to Upload.
*
* @param clientStatus the client status
* @param serverStatus the server status
* @return the list
*/
List fetchPacketsToUpload(List clientStatus, String serverStatus);
List fetchReRegisterPendingPackets();
List getAllRegistrations();
List