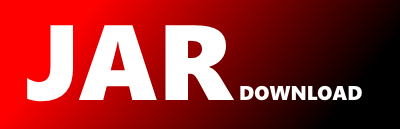
io.mosip.registration.repositories.RegistrationRepository Maven / Gradle / Ivy
package io.mosip.registration.repositories;
import java.sql.Timestamp;
import java.util.List;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Slice;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.query.Param;
import io.mosip.kernel.core.dataaccess.spi.repository.BaseRepository;
import io.mosip.registration.entity.Registration;
/**
* The repository interface for {@link Registration}
*
* @author Balaji Sridharan
* @since 1.0.0
*
*/
public interface RegistrationRepository extends BaseRepository {
/**
* This method returns the list of {@link Registration} based on provided id's.
*
* @param clientstatusCode
* the clientstatus code
* @param exportstatusCode
* the exportstatus code
* @param serverStatusCode
* the server status code
* @param fileUploadStatus
* the file upload status
* @return the list of {@link Registration}
*/
@Query("select reg from Registration reg where reg.clientStatusCode= :syncStatus or reg.clientStatusCode= :exportStatus and (reg.serverStatusCode=:resendStatus or reg.serverStatusCode IS NULL) or reg.fileUploadStatus=:fileUploadStatus")
List findByStatusCodes(@Param("syncStatus") String clientstatusCode, @Param("exportStatus") String exportstatusCode,
@Param("resendStatus") String serverStatusCode, @Param("fileUploadStatus") String fileUploadStatus);
/**
* This method returns the list of {@link Registration} based on status code
*
* @param statusCode
* the status code
* @return the list of {@link Registration}
*/
List findByclientStatusCodeOrderByCrDtime(String statusCode);
/**
* This method returns the list of {@link Registration} based on status code or status comments
*
* @param statusCode
* @param statusComments
* @return the list of {@link Registration}
*/
List findByClientStatusCodeOrClientStatusCommentsOrderByCrDtime(String statusCode, String statusComments);
/**
* This method fetches the registration packets based on given client status
* codes.
*
* @param statusCodes
* the status codes
* @return List of registration packets
*/
List findByClientStatusCodeInOrderByUpdDtimesDesc(List statusCodes);
List findByClientStatusCodeInOrderByCrDtimeAsc(List statusCodes);
/**
* To fetch the records for Packet Upload.
*
* @param statusCodes
* the status codes
* @param serverStatus
* the server status
* @return List of registration packets
*/
List findByClientStatusCodeInOrServerStatusCodeOrderByUpdDtimesDesc(List statusCodes,String serverStatus);
/**
* Fetching all the re registration records.
*
* @param clientStatus
* the client status
* @param serverStatus
* the server status
* @return List of registration packets
*/
List findByClientStatusCodeAndServerStatusCodeIn(String clientStatus, List serverStatus);
/**
* Find by CrDtimes and client status code.
*
* @param crDtimes
* the date upto packets to be deleted
* @return list of registrations
*/
List findByCrDtimeBefore(Timestamp crDtimes);
/**
* This method returns the list of {@link Registration} based on status code.
*
* @param statusCode
* the status code
* @return the list of {@link Registration}
*/
List findByclientStatusCodeOrderByCrDtimeAsc(String statusCode);
/**
* Find by CrDtimes and server status code.
*
* @param crDtimes
* the date upto packets to be deleted
* @param serverStatus
* status of resgistrationPacket
* @return list of registrations
*/
List findByCrDtimeBeforeAndServerStatusCodeIn(Timestamp crDtimes, List statusCodes);
/**
* fetches all the Registration records which is having the given server status
* codes.
*
* @param statusCodes
* the status codes
* @return the list of registrations
*/
List findByServerStatusCodeIn(List statusCodes);
/**
* fetches all the Registration records which is not having the given server
* status codes.
*
* @param statusCodes
* the status codes
* @return the list of registrations
*/
List findByServerStatusCodeNotInOrServerStatusCodeIsNull(List statusCodes);
/**
* Fetches all the re register pending records
* @param clientStatusCodes
* @param serverStatusCodes
* @return
*/
List findByClientStatusCodeNotInAndServerStatusCodeIn(List clientStatusCodes,
List serverStatusCodes);
@Query("select clientStatusCode, serverStatusCode, count(packetId) from Registration group by clientStatusCode, serverStatusCode")
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy