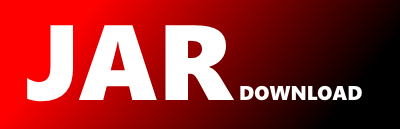
io.muserver.HttpConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mu-server Show documentation
Show all versions of mu-server Show documentation
A simple but powerful web server framework
The newest version!
package io.muserver;
import javax.net.ssl.TrustManager;
import java.net.InetSocketAddress;
import java.security.cert.Certificate;
import java.time.Instant;
import java.util.Optional;
import java.util.Set;
import java.util.concurrent.ExecutorService;
/**
* A connection between a server and a client.
*/
public interface HttpConnection {
/**
* The HTTP protocol for the request.
* @return A string such as HTTP/1.1
or HTTP/2
*/
String protocol();
/**
* @return true
if the connnection is secured over HTTPS, otherwise false
*/
boolean isHttps();
/**
* Gets the HTTPS protocol, for example "TLSv1.2" or "TLSv1.3"
* @return The HTTPS protocol being used, or null
if this connection is not over HTTPS.
*/
String httpsProtocol();
/**
* @return The HTTPS cipher used on this connection, or null
if this connection is not over HTTPS.
*/
String cipher();
/**
* @return The time that this connection was established.
*/
Instant startTime();
/**
* @return The socket address of the client.
*/
InetSocketAddress remoteAddress();
/**
* @return The number of completed requests on this connection.
*/
long completedRequests();
/**
* @return The number of requests received that were not valid HTTP messages.
*/
long invalidHttpRequests();
/**
* @return The number of requests rejected because the executor passed to {@link MuServerBuilder#withHandlerExecutor(ExecutorService)}
* rejected a new response.
*/
long rejectedDueToOverload();
/**
* @return A readonly connection of requests that are in progress on this connection
*/
Set activeRequests();
/**
* The websockets on this connection.
* Note that in Mu Server websockets are only on HTTP/1.1 connections and there is a 1:1 mapping between
* a websocket and an HTTP Connection. This means the returned set is either empty or has a size of 1.
* @return A readonly set of active websockets being used on this connection
*/
Set activeWebsockets();
/**
* @return The server that this connection belongs to
*/
MuServer server();
/**
* Gets the TLS certificate the client sent.
* The returned certificate will be {@link Optional#empty()} when:
*
* - The client did not send a certificate, or
* - The client sent a certificate that failed verification with the client trust manager, or
* - No client trust manager was set with {@link HttpsConfigBuilder#withClientCertificateTrustManager(TrustManager)}, or
* - The request was not sent over HTTPS
*
* @return The client certificate, or empty
if no certificate is available
*/
Optional clientCertificate();
/**
* Gets information from a proxy that uses the HA
* Proxy protocol.
* Note: this is only available if enabled via {@link MuServerBuilder#withHAProxyProtocolEnabled(boolean)}
* @return Information from a proxy about the source client, or {@link Optional#empty()} if none specified.
*/
Optional proxyInfo();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy