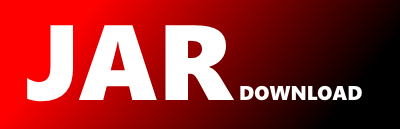
io.muserver.MuWebSocketSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mu-server Show documentation
Show all versions of mu-server Show documentation
A simple but powerful web server framework
The newest version!
package io.muserver;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
/**
* A web socket session used to send messages and events to a web socket client.
* The simplest way to get a reference to a session is to extend {@link BaseWebSocket} and use the {@link BaseWebSocket#session()} method.
*/
public interface MuWebSocketSession {
/**
* Sends a message to the client asynchronously
* @param message The message to be sent
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendText(String message, DoneCallback doneCallback);
/**
* Sends a full or partial message to the client asynchronously with an optional parameter allowing partial fragments to be sent
* @param message The message to be sent
* @param isLastFragment If false
then this message will be sent as a partial fragment
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendText(String message, boolean isLastFragment, DoneCallback doneCallback);
/**
* Sends a message to the client asynchronously
* @param message The message to be sent
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendBinary(ByteBuffer message, DoneCallback doneCallback);
/**
* Sends a full or partial message to the client asynchronously with an optional parameter allowing partial fragments to be sent
* @param message The message to be sent
* @param isLastFragment If false
then this message will be sent as a partial fragment
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendBinary(ByteBuffer message, boolean isLastFragment, DoneCallback doneCallback);
/**
* Sends a ping message to the client, which is used for keeping sockets alive.
* @param payload The message to send.
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendPing(ByteBuffer payload, DoneCallback doneCallback);
/**
* Sends a pong message to the client, generally in response to receiving a ping via {@link MuWebSocket#onPing(ByteBuffer, DoneCallback)}
* @param payload The payload to send back to the client.
* @param doneCallback The callback to call when the write succeeds or fails. To ignore the write result, you can
* use {@link DoneCallback#NoOp}. If using a buffer received from a {@link MuWebSocket} event,
* pass the onComplete
received to this parameter.
*/
void sendPong(ByteBuffer payload, DoneCallback doneCallback);
/**
* Initiates a graceful shutdown with the client.
* @throws IOException Thrown if there is an error writing to the client, for example if the user has closed their browser.
*/
void close() throws IOException;
/**
* Initiates a graceful shutdown with the client.
* @param statusCode The status code to send, such as 1000
. See https://tools.ietf.org/html/rfc6455#section-7.4
* @param reason An optional reason for closing.
* @throws IOException Thrown if there is an error writing to the client, for example if the user has closed their browser.
*/
void close(int statusCode, String reason) throws IOException;
/**
* @return The client's address
*/
InetSocketAddress remoteAddress();
/**
* @return The state of the current session
*/
WebsocketSessionState state();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy