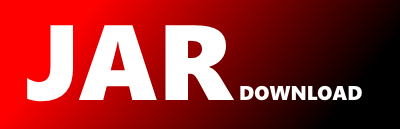
io.muserver.openapi.ResponseObjectBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mu-server Show documentation
Show all versions of mu-server Show documentation
A simple but powerful web server framework
The newest version!
package io.muserver.openapi;
import java.util.HashMap;
import java.util.Map;
import static io.muserver.openapi.OpenApiUtils.immutable;
/**
* Describes a single response from an API Operation, including design-time, static links
to operations
* based on the response.
*/
public class ResponseObjectBuilder {
private String description;
private Map headers;
private Map content;
private Map links;
/**
* @param description REQUIRED. A short description of the response.
* CommonMark syntax MAY be used for rich text representation.
* @return The current builder
*/
public ResponseObjectBuilder withDescription(String description) {
this.description = description;
return this;
}
/**
* @param headers Maps a header name to its definition. RFC7230
* states header names are case insensitive. If a response header is defined with the name
* "Content-Type"
, it SHALL be ignored.
* @return The current builder
*/
public ResponseObjectBuilder withHeaders(Map headers) {
this.headers = headers;
return this;
}
/**
* @param content A map containing descriptions of potential response payloads. The key is a media type or
* media type range and the value
* describes it. For responses that match multiple keys, only the most specific key is applicable.
* e.g. text/plain overrides text/*
* @return The current builder
*/
public ResponseObjectBuilder withContent(Map content) {
this.content = content;
return this;
}
/**
* @param links A map of operations links that can be followed from the response.
* @return The current builder
*/
public ResponseObjectBuilder withLinks(Map links) {
this.links = links;
return this;
}
/**
* @return A new object
*/
public ResponseObject build() {
return new ResponseObject(description, immutable(headers), immutable(content), immutable(links));
}
/**
* Creates a builder for a {@link ResponseObject}
*
* @return A new builder
*/
public static ResponseObjectBuilder responseObject() {
return new ResponseObjectBuilder();
}
/**
* Creates a new build by merging two existing response objects
* @param primary A responses object to use. This is the dominant response who's values will
* be preferred when values cannot be merged (such as {@link ResponseObject#description}
* @param secondary The other responses object
* @return A builder that is the merged value of the two given ones
*/
public static ResponseObjectBuilder mergeResponses(ResponseObject primary, ResponseObject secondary) {
Map mergedHeaders = new HashMap<>();
addHeaders(mergedHeaders, primary);
addHeaders(mergedHeaders, secondary);
Map mergedContent = new HashMap<>();
addContent(mergedContent, primary);
addContent(mergedContent, secondary);
Map mergedLinks = new HashMap<>();
addLinks(mergedLinks, primary);
addLinks(mergedLinks, secondary);
return responseObject()
.withDescription(primary != null ? primary.description() : secondary != null ? secondary.description() : null)
.withHeaders(mergedHeaders.isEmpty() ? null : mergedHeaders)
.withContent(mergedContent.isEmpty() ? null : mergedContent)
.withLinks(mergedLinks.isEmpty() ? null : mergedLinks);
}
private static void addLinks(Map dest, ResponseObject source) {
if (source != null && source.links() != null) {
for (Map.Entry entry : source.links().entrySet()) {
String name = entry.getKey();
if (!dest.containsKey(name)) {
dest.put(name, entry.getValue());
}
}
}
}
private static void addContent(Map dest, ResponseObject source) {
if (source != null && source.content() != null) {
for (Map.Entry entry : source.content().entrySet()) {
String name = entry.getKey();
if (!dest.containsKey(name)) {
dest.put(name, entry.getValue());
}
}
}
}
private static void addHeaders(Map dest, ResponseObject source) {
if (source != null && source.headers() != null) {
for (Map.Entry entry : source.headers().entrySet()) {
String name = entry.getKey();
if (!dest.containsKey(name)) {
dest.put(name, entry.getValue()); // merging headers is too complicated so just add missing headers
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy