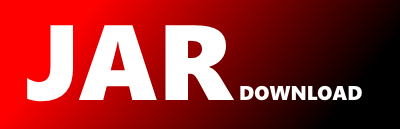
commonMain.io.nacular.doodle.utils.Interpolator.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of controls Show documentation
Show all versions of controls Show documentation
A pure Kotlin, UI framework for the Web and Desktop
package io.nacular.doodle.utils
import io.nacular.measured.units.Measure
import io.nacular.measured.units.Units
import io.nacular.measured.units.div
/**
* Allows interpolating between two start and end values of [T] given some progress.
*/
public interface Interpolator {
/**
* Interpolates between [start] and [end] (inclusive).
*
* @param start value
* @param end value
* @param progress between [start] and [end] in the range [0-1]
* @return the value
*/
public fun lerp(start: T, end: T, progress: Float): T
/**
* Returns the progress of [value] between [start] and [end] (inclusive).
*
* @param start value
* @param end value
* @param value being checked
* @return the progress of [value] between [start] and [end]
*/
public fun progress(start: T, end: T, value: T): Float
/**
* Returns a number to represent [value] to accessibility systems.
*
* @param start value
* @param end value
* @param value being checked
* @return the numeric representation of [value]
*/
public fun accessibleNumericValue(start: T, end: T, value: T): Double = progress(start, end, value).toDouble()
}
/**
* [Interpolator] for [Measure]s
*/
public val T.interpolator: Interpolator> get() = object: Interpolator> {
override fun lerp (start: Measure, end: Measure, progress: Float ) = io.nacular.doodle.utils.lerp(start, end, progress)
override fun progress(start: Measure, end: Measure, value : Measure) = ((value - start) / (end - start)).toFloat()
override fun accessibleNumericValue(start: Measure, end: Measure, value: Measure) = value.amount
}
/**
* [Interpolator] for [Char].
*/
internal object CharInterpolator: Interpolator {
override fun lerp (start: Char, end: Char, progress: Float) = (lerp(start.code.toDouble(), end.code.toDouble(), progress)).toInt().toChar()
override fun progress(start: Char, end: Char, value : Char) = ((value - start).toDouble() / (end - start)).toFloat()
override fun accessibleNumericValue(start: Char, end: Char, value: Char): Double = value.code.toDouble()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy