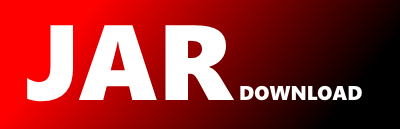
io.nem.symbol.catapult.builders.AccountMosaicRestrictionTransactionBodyBuilder Maven / Gradle / Ivy
/**
*** Copyright (c) 2016-present,
*** Jaguar0625, gimre, BloodyRookie, Tech Bureau, Corp. All rights reserved.
***
*** This file is part of Catapult.
***
*** Catapult is free software: you can redistribute it and/or modify
*** it under the terms of the GNU Lesser General Public License as published by
*** the Free Software Foundation, either version 3 of the License, or
*** (at your option) any later version.
***
*** Catapult is distributed in the hope that it will be useful,
*** but WITHOUT ANY WARRANTY; without even the implied warranty of
*** MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
*** GNU Lesser General Public License for more details.
***
*** You should have received a copy of the GNU Lesser General Public License
*** along with Catapult. If not, see .
**/
package io.nem.symbol.catapult.builders;
import java.io.DataInputStream;
import java.nio.ByteBuffer;
import java.util.EnumSet;
import java.util.List;
/**
* Binary layout for an account mosaic restriction transaction
**/
public class AccountMosaicRestrictionTransactionBodyBuilder implements Serializer {
/** Account restriction flags. **/
private final EnumSet restrictionFlags;
/** Reserved padding to align restrictionAdditions on 8-byte boundary. **/
private final int accountRestrictionTransactionBody_Reserved1;
/** Account restriction additions. **/
private final List restrictionAdditions;
/** Account restriction deletions. **/
private final List restrictionDeletions;
/**
* Constructor - Creates an object from stream.
*
* @param stream Byte stream to use to serialize the object.
*/
protected AccountMosaicRestrictionTransactionBodyBuilder(DataInputStream stream) {
try {
this.restrictionFlags = GeneratorUtils.toSet(AccountRestrictionFlagsDto.class, Short.reverseBytes(stream.readShort()));
final byte restrictionAdditionsCount = stream.readByte();
final byte restrictionDeletionsCount = stream.readByte();
this.accountRestrictionTransactionBody_Reserved1 = Integer.reverseBytes(stream.readInt());
this.restrictionAdditions = GeneratorUtils.loadFromBinaryArray(UnresolvedMosaicIdDto::loadFromBinary, stream, restrictionAdditionsCount);
this.restrictionDeletions = GeneratorUtils.loadFromBinaryArray(UnresolvedMosaicIdDto::loadFromBinary, stream, restrictionDeletionsCount);
} catch (Exception e) {
throw GeneratorUtils.getExceptionToPropagate(e);
}
}
/**
* Creates an instance of AccountMosaicRestrictionTransactionBodyBuilder from a stream.
*
* @param stream Byte stream to use to serialize the object.
* @return Instance of AccountMosaicRestrictionTransactionBodyBuilder.
*/
public static AccountMosaicRestrictionTransactionBodyBuilder loadFromBinary(DataInputStream stream) {
return new AccountMosaicRestrictionTransactionBodyBuilder(stream);
}
/**
* Constructor.
*
* @param restrictionFlags Account restriction flags.
* @param restrictionAdditions Account restriction additions.
* @param restrictionDeletions Account restriction deletions.
*/
protected AccountMosaicRestrictionTransactionBodyBuilder(EnumSet restrictionFlags, List restrictionAdditions, List restrictionDeletions) {
GeneratorUtils.notNull(restrictionFlags, "restrictionFlags is null");
GeneratorUtils.notNull(restrictionAdditions, "restrictionAdditions is null");
GeneratorUtils.notNull(restrictionDeletions, "restrictionDeletions is null");
this.restrictionFlags = restrictionFlags;
this.accountRestrictionTransactionBody_Reserved1 = 0;
this.restrictionAdditions = restrictionAdditions;
this.restrictionDeletions = restrictionDeletions;
}
/**
* Creates an instance of AccountMosaicRestrictionTransactionBodyBuilder.
*
* @param restrictionFlags Account restriction flags.
* @param restrictionAdditions Account restriction additions.
* @param restrictionDeletions Account restriction deletions.
* @return Instance of AccountMosaicRestrictionTransactionBodyBuilder.
*/
public static AccountMosaicRestrictionTransactionBodyBuilder create(EnumSet restrictionFlags, List restrictionAdditions, List restrictionDeletions) {
return new AccountMosaicRestrictionTransactionBodyBuilder(restrictionFlags, restrictionAdditions, restrictionDeletions);
}
/**
* Gets account restriction flags.
*
* @return Account restriction flags.
*/
public EnumSet getRestrictionFlags() {
return this.restrictionFlags;
}
/**
* Gets reserved padding to align restrictionAdditions on 8-byte boundary.
*
* @return Reserved padding to align restrictionAdditions on 8-byte boundary.
*/
private int getAccountRestrictionTransactionBody_Reserved1() {
return this.accountRestrictionTransactionBody_Reserved1;
}
/**
* Gets account restriction additions.
*
* @return Account restriction additions.
*/
public List getRestrictionAdditions() {
return this.restrictionAdditions;
}
/**
* Gets account restriction deletions.
*
* @return Account restriction deletions.
*/
public List getRestrictionDeletions() {
return this.restrictionDeletions;
}
/**
* Gets the size of the object.
*
* @return Size in bytes.
*/
public int getSize() {
int size = 0;
size += AccountRestrictionFlagsDto.values()[0].getSize();
size += 1; // restrictionAdditionsCount
size += 1; // restrictionDeletionsCount
size += 4; // accountRestrictionTransactionBody_Reserved1
size += this.restrictionAdditions.stream().mapToInt(o -> o.getSize()).sum();
size += this.restrictionDeletions.stream().mapToInt(o -> o.getSize()).sum();
return size;
}
/**
* Serializes an object to bytes.
*
* @return Serialized bytes.
*/
public byte[] serialize() {
return GeneratorUtils.serialize((dataOutputStream) -> {
dataOutputStream.writeShort(Short.reverseBytes((short) GeneratorUtils.toLong(AccountRestrictionFlagsDto.class, this.restrictionFlags)));
dataOutputStream.writeByte((byte) GeneratorUtils.getSize(this.getRestrictionAdditions()));
dataOutputStream.writeByte((byte) GeneratorUtils.getSize(this.getRestrictionDeletions()));
dataOutputStream.writeInt(Integer.reverseBytes((int) this.getAccountRestrictionTransactionBody_Reserved1()));
GeneratorUtils.writeList(dataOutputStream, this.restrictionAdditions);
GeneratorUtils.writeList(dataOutputStream, this.restrictionDeletions);
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy