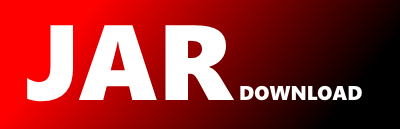
io.nem.symbol.catapult.builders.BlockHeaderBuilder Maven / Gradle / Ivy
/**
*** Copyright (c) 2016-present,
*** Jaguar0625, gimre, BloodyRookie, Tech Bureau, Corp. All rights reserved.
***
*** This file is part of Catapult.
***
*** Catapult is free software: you can redistribute it and/or modify
*** it under the terms of the GNU Lesser General Public License as published by
*** the Free Software Foundation, either version 3 of the License, or
*** (at your option) any later version.
***
*** Catapult is distributed in the hope that it will be useful,
*** but WITHOUT ANY WARRANTY; without even the implied warranty of
*** MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
*** GNU Lesser General Public License for more details.
***
*** You should have received a copy of the GNU Lesser General Public License
*** along with Catapult. If not, see .
**/
package io.nem.symbol.catapult.builders;
import java.io.DataInputStream;
import java.nio.ByteBuffer;
import java.util.EnumSet;
import java.util.List;
/**
* Binary layout for a block header
**/
public class BlockHeaderBuilder implements Serializer {
/** Entity size. **/
private int size;
/** Reserved padding to align Signature on 8-byte boundary. **/
private final int verifiableEntityHeader_Reserved1;
/** Entity signature. **/
private final SignatureDto signature;
/** Entity signer's public key. **/
private final KeyDto signerPublicKey;
/** Reserved padding to align end of EntityBody on 8-byte boundary. **/
private final int entityBody_Reserved1;
/** Entity version. **/
private final byte version;
/** Entity network. **/
private final NetworkTypeDto network;
/** Entity type. **/
private final EntityTypeDto type;
/** Block height. **/
private final HeightDto height;
/** Number of milliseconds elapsed since creation of nemesis block. **/
private final TimestampDto timestamp;
/** Block difficulty. **/
private final DifficultyDto difficulty;
/** Generation hash proof. **/
private final VrfProofBuilder generationHashProof;
/** Previous block hash. **/
private final Hash256Dto previousBlockHash;
/** Hash of the transactions in this block. **/
private final Hash256Dto transactionsHash;
/** Hash of the receipts generated by this block. **/
private final Hash256Dto receiptsHash;
/** Hash of the global chain state at this block. **/
private final Hash256Dto stateHash;
/** Beneficiary address designated by harvester. **/
private final AddressDto beneficiaryAddress;
/** Fee multiplier applied to block transactions. **/
private final BlockFeeMultiplierDto feeMultiplier;
/** Reserved padding to align end of BlockHeader on 8-byte boundary. **/
private final int blockHeader_Reserved1;
/**
* Constructor - Creates an object from stream.
*
* @param stream Byte stream to use to serialize the object.
*/
protected BlockHeaderBuilder(DataInputStream stream) {
try {
this.size = Integer.reverseBytes(stream.readInt());
this.verifiableEntityHeader_Reserved1 = Integer.reverseBytes(stream.readInt());
this.signature = SignatureDto.loadFromBinary(stream);
this.signerPublicKey = KeyDto.loadFromBinary(stream);
this.entityBody_Reserved1 = Integer.reverseBytes(stream.readInt());
this.version = stream.readByte();
this.network = NetworkTypeDto.loadFromBinary(stream);
this.type = EntityTypeDto.loadFromBinary(stream);
this.height = HeightDto.loadFromBinary(stream);
this.timestamp = TimestampDto.loadFromBinary(stream);
this.difficulty = DifficultyDto.loadFromBinary(stream);
this.generationHashProof = VrfProofBuilder.loadFromBinary(stream);
this.previousBlockHash = Hash256Dto.loadFromBinary(stream);
this.transactionsHash = Hash256Dto.loadFromBinary(stream);
this.receiptsHash = Hash256Dto.loadFromBinary(stream);
this.stateHash = Hash256Dto.loadFromBinary(stream);
this.beneficiaryAddress = AddressDto.loadFromBinary(stream);
this.feeMultiplier = BlockFeeMultiplierDto.loadFromBinary(stream);
this.blockHeader_Reserved1 = Integer.reverseBytes(stream.readInt());
} catch (Exception e) {
throw GeneratorUtils.getExceptionToPropagate(e);
}
}
/**
* Creates an instance of BlockHeaderBuilder from a stream.
*
* @param stream Byte stream to use to serialize the object.
* @return Instance of BlockHeaderBuilder.
*/
public static BlockHeaderBuilder loadFromBinary(DataInputStream stream) {
return new BlockHeaderBuilder(stream);
}
/**
* Constructor.
*
* @param signature Entity signature.
* @param signerPublicKey Entity signer's public key.
* @param version Entity version.
* @param network Entity network.
* @param type Entity type.
* @param height Block height.
* @param timestamp Number of milliseconds elapsed since creation of nemesis block.
* @param difficulty Block difficulty.
* @param generationHashProof Generation hash proof.
* @param previousBlockHash Previous block hash.
* @param transactionsHash Hash of the transactions in this block.
* @param receiptsHash Hash of the receipts generated by this block.
* @param stateHash Hash of the global chain state at this block.
* @param beneficiaryAddress Beneficiary address designated by harvester.
* @param feeMultiplier Fee multiplier applied to block transactions.
*/
protected BlockHeaderBuilder(SignatureDto signature, KeyDto signerPublicKey, byte version, NetworkTypeDto network, EntityTypeDto type, HeightDto height, TimestampDto timestamp, DifficultyDto difficulty, VrfProofBuilder generationHashProof, Hash256Dto previousBlockHash, Hash256Dto transactionsHash, Hash256Dto receiptsHash, Hash256Dto stateHash, AddressDto beneficiaryAddress, BlockFeeMultiplierDto feeMultiplier) {
GeneratorUtils.notNull(signature, "signature is null");
GeneratorUtils.notNull(signerPublicKey, "signerPublicKey is null");
GeneratorUtils.notNull(version, "version is null");
GeneratorUtils.notNull(network, "network is null");
GeneratorUtils.notNull(type, "type is null");
GeneratorUtils.notNull(height, "height is null");
GeneratorUtils.notNull(timestamp, "timestamp is null");
GeneratorUtils.notNull(difficulty, "difficulty is null");
GeneratorUtils.notNull(generationHashProof, "generationHashProof is null");
GeneratorUtils.notNull(previousBlockHash, "previousBlockHash is null");
GeneratorUtils.notNull(transactionsHash, "transactionsHash is null");
GeneratorUtils.notNull(receiptsHash, "receiptsHash is null");
GeneratorUtils.notNull(stateHash, "stateHash is null");
GeneratorUtils.notNull(beneficiaryAddress, "beneficiaryAddress is null");
GeneratorUtils.notNull(feeMultiplier, "feeMultiplier is null");
this.verifiableEntityHeader_Reserved1 = 0;
this.signature = signature;
this.signerPublicKey = signerPublicKey;
this.entityBody_Reserved1 = 0;
this.version = version;
this.network = network;
this.type = type;
this.height = height;
this.timestamp = timestamp;
this.difficulty = difficulty;
this.generationHashProof = generationHashProof;
this.previousBlockHash = previousBlockHash;
this.transactionsHash = transactionsHash;
this.receiptsHash = receiptsHash;
this.stateHash = stateHash;
this.beneficiaryAddress = beneficiaryAddress;
this.feeMultiplier = feeMultiplier;
this.blockHeader_Reserved1 = 0;
}
/**
* Creates an instance of BlockHeaderBuilder.
*
* @param signature Entity signature.
* @param signerPublicKey Entity signer's public key.
* @param version Entity version.
* @param network Entity network.
* @param type Entity type.
* @param height Block height.
* @param timestamp Number of milliseconds elapsed since creation of nemesis block.
* @param difficulty Block difficulty.
* @param generationHashProof Generation hash proof.
* @param previousBlockHash Previous block hash.
* @param transactionsHash Hash of the transactions in this block.
* @param receiptsHash Hash of the receipts generated by this block.
* @param stateHash Hash of the global chain state at this block.
* @param beneficiaryAddress Beneficiary address designated by harvester.
* @param feeMultiplier Fee multiplier applied to block transactions.
* @return Instance of BlockHeaderBuilder.
*/
public static BlockHeaderBuilder create(SignatureDto signature, KeyDto signerPublicKey, byte version, NetworkTypeDto network, EntityTypeDto type, HeightDto height, TimestampDto timestamp, DifficultyDto difficulty, VrfProofBuilder generationHashProof, Hash256Dto previousBlockHash, Hash256Dto transactionsHash, Hash256Dto receiptsHash, Hash256Dto stateHash, AddressDto beneficiaryAddress, BlockFeeMultiplierDto feeMultiplier) {
return new BlockHeaderBuilder(signature, signerPublicKey, version, network, type, height, timestamp, difficulty, generationHashProof, previousBlockHash, transactionsHash, receiptsHash, stateHash, beneficiaryAddress, feeMultiplier);
}
/**
* Gets entity size.
*
* @return Entity size.
*/
public int getStreamSize() {
return this.size;
}
/**
* Gets reserved padding to align Signature on 8-byte boundary.
*
* @return Reserved padding to align Signature on 8-byte boundary.
*/
private int getVerifiableEntityHeader_Reserved1() {
return this.verifiableEntityHeader_Reserved1;
}
/**
* Gets entity signature.
*
* @return Entity signature.
*/
public SignatureDto getSignature() {
return this.signature;
}
/**
* Gets entity signer's public key.
*
* @return Entity signer's public key.
*/
public KeyDto getSignerPublicKey() {
return this.signerPublicKey;
}
/**
* Gets reserved padding to align end of EntityBody on 8-byte boundary.
*
* @return Reserved padding to align end of EntityBody on 8-byte boundary.
*/
private int getEntityBody_Reserved1() {
return this.entityBody_Reserved1;
}
/**
* Gets entity version.
*
* @return Entity version.
*/
public byte getVersion() {
return this.version;
}
/**
* Gets entity network.
*
* @return Entity network.
*/
public NetworkTypeDto getNetwork() {
return this.network;
}
/**
* Gets entity type.
*
* @return Entity type.
*/
public EntityTypeDto getType() {
return this.type;
}
/**
* Gets block height.
*
* @return Block height.
*/
public HeightDto getHeight() {
return this.height;
}
/**
* Gets number of milliseconds elapsed since creation of nemesis block.
*
* @return Number of milliseconds elapsed since creation of nemesis block.
*/
public TimestampDto getTimestamp() {
return this.timestamp;
}
/**
* Gets block difficulty.
*
* @return Block difficulty.
*/
public DifficultyDto getDifficulty() {
return this.difficulty;
}
/**
* Gets generation hash proof.
*
* @return Generation hash proof.
*/
public VrfProofBuilder getGenerationHashProof() {
return this.generationHashProof;
}
/**
* Gets previous block hash.
*
* @return Previous block hash.
*/
public Hash256Dto getPreviousBlockHash() {
return this.previousBlockHash;
}
/**
* Gets hash of the transactions in this block.
*
* @return Hash of the transactions in this block.
*/
public Hash256Dto getTransactionsHash() {
return this.transactionsHash;
}
/**
* Gets hash of the receipts generated by this block.
*
* @return Hash of the receipts generated by this block.
*/
public Hash256Dto getReceiptsHash() {
return this.receiptsHash;
}
/**
* Gets hash of the global chain state at this block.
*
* @return Hash of the global chain state at this block.
*/
public Hash256Dto getStateHash() {
return this.stateHash;
}
/**
* Gets beneficiary address designated by harvester.
*
* @return Beneficiary address designated by harvester.
*/
public AddressDto getBeneficiaryAddress() {
return this.beneficiaryAddress;
}
/**
* Gets fee multiplier applied to block transactions.
*
* @return Fee multiplier applied to block transactions.
*/
public BlockFeeMultiplierDto getFeeMultiplier() {
return this.feeMultiplier;
}
/**
* Gets reserved padding to align end of BlockHeader on 8-byte boundary.
*
* @return Reserved padding to align end of BlockHeader on 8-byte boundary.
*/
private int getBlockHeader_Reserved1() {
return this.blockHeader_Reserved1;
}
/**
* Gets the size of the object.
*
* @return Size in bytes.
*/
public int getSize() {
int size = 0;
size += 4; // size
size += 4; // verifiableEntityHeader_Reserved1
size += this.signature.getSize();
size += this.signerPublicKey.getSize();
size += 4; // entityBody_Reserved1
size += 1; // version
size += this.network.getSize();
size += this.type.getSize();
size += this.height.getSize();
size += this.timestamp.getSize();
size += this.difficulty.getSize();
size += this.generationHashProof.getSize();
size += this.previousBlockHash.getSize();
size += this.transactionsHash.getSize();
size += this.receiptsHash.getSize();
size += this.stateHash.getSize();
size += this.beneficiaryAddress.getSize();
size += this.feeMultiplier.getSize();
size += 4; // blockHeader_Reserved1
return size;
}
/**
* Serializes an object to bytes.
*
* @return Serialized bytes.
*/
public byte[] serialize() {
return GeneratorUtils.serialize((dataOutputStream) -> {
dataOutputStream.writeInt(Integer.reverseBytes((int) this.getSize()));
dataOutputStream.writeInt(Integer.reverseBytes((int) this.getVerifiableEntityHeader_Reserved1()));
GeneratorUtils.writeEntity(dataOutputStream, this.signature);
GeneratorUtils.writeEntity(dataOutputStream, this.signerPublicKey);
dataOutputStream.writeInt(Integer.reverseBytes((int) this.getEntityBody_Reserved1()));
dataOutputStream.writeByte((byte) this.getVersion());
GeneratorUtils.writeEntity(dataOutputStream, this.network);
GeneratorUtils.writeEntity(dataOutputStream, this.type);
GeneratorUtils.writeEntity(dataOutputStream, this.height);
GeneratorUtils.writeEntity(dataOutputStream, this.timestamp);
GeneratorUtils.writeEntity(dataOutputStream, this.difficulty);
GeneratorUtils.writeEntity(dataOutputStream, this.generationHashProof);
GeneratorUtils.writeEntity(dataOutputStream, this.previousBlockHash);
GeneratorUtils.writeEntity(dataOutputStream, this.transactionsHash);
GeneratorUtils.writeEntity(dataOutputStream, this.receiptsHash);
GeneratorUtils.writeEntity(dataOutputStream, this.stateHash);
GeneratorUtils.writeEntity(dataOutputStream, this.beneficiaryAddress);
GeneratorUtils.writeEntity(dataOutputStream, this.feeMultiplier);
dataOutputStream.writeInt(Integer.reverseBytes((int) this.getBlockHeader_Reserved1()));
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy