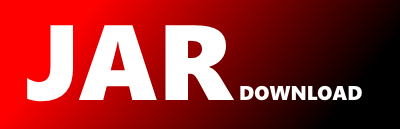
io.nem.symbol.sdk.openapi.jersey2.api.HashLockRoutesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-openapi-jersey2-client Show documentation
Show all versions of symbol-openapi-jersey2-client Show documentation
symbol-openapi-jersey2-client Generated Open API client for symbol-sdk-java
package io.nem.symbol.sdk.openapi.jersey2.api;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiException;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiClient;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiResponse;
import io.nem.symbol.sdk.openapi.jersey2.invoker.Configuration;
import io.nem.symbol.sdk.openapi.jersey2.invoker.Pair;
import javax.ws.rs.core.GenericType;
import io.nem.symbol.sdk.openapi.jersey2.model.HashLockInfoDTO;
import io.nem.symbol.sdk.openapi.jersey2.model.HashLockPage;
import io.nem.symbol.sdk.openapi.jersey2.model.MerkleStateInfoDTO;
import io.nem.symbol.sdk.openapi.jersey2.model.ModelError;
import io.nem.symbol.sdk.openapi.jersey2.model.Order;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2021-02-02T19:39:53.661Z[UTC]")
public class HashLockRoutesApi {
private ApiClient apiClient;
public HashLockRoutesApi() {
this(Configuration.getDefaultApiClient());
}
public HashLockRoutesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get hash lock information
* Gets the hash lock for a given hash.
* @param hash Filter by hash. (required)
* @return HashLockInfoDTO
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public HashLockInfoDTO getHashLock(String hash) throws ApiException {
return getHashLockWithHttpInfo(hash).getData();
}
/**
* Get hash lock information
* Gets the hash lock for a given hash.
* @param hash Filter by hash. (required)
* @return ApiResponse<HashLockInfoDTO>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse getHashLockWithHttpInfo(String hash) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'hash' is set
if (hash == null) {
throw new ApiException(400, "Missing the required parameter 'hash' when calling getHashLock");
}
// create path and map variables
String localVarPath = "/lock/hash/{hash}"
.replaceAll("\\{" + "hash" + "\\}", apiClient.escapeString(hash.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("HashLockRoutesApi.getHashLock", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get hash lock merkle information
* Gets the hash lock merkle for a given hash.
* @param hash Filter by hash. (required)
* @return MerkleStateInfoDTO
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public MerkleStateInfoDTO getHashLockMerkle(String hash) throws ApiException {
return getHashLockMerkleWithHttpInfo(hash).getData();
}
/**
* Get hash lock merkle information
* Gets the hash lock merkle for a given hash.
* @param hash Filter by hash. (required)
* @return ApiResponse<MerkleStateInfoDTO>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse getHashLockMerkleWithHttpInfo(String hash) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'hash' is set
if (hash == null) {
throw new ApiException(400, "Missing the required parameter 'hash' when calling getHashLockMerkle");
}
// create path and map variables
String localVarPath = "/lock/hash/{hash}/merkle"
.replaceAll("\\{" + "hash" + "\\}", apiClient.escapeString(hash.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("HashLockRoutesApi.getHashLockMerkle", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Search hash lock entries
* Returns an array of hash locks.
* @param address Filter by address involved in the transaction. An account's address is considered to be involved in the transaction when the account is the sender, recipient, or it is required to cosign the transaction. This filter cannot be combined with ``recipientAddress`` and ``signerPublicKey`` query params. (optional)
* @param pageSize Select the number of entries to return. (optional, default to 10)
* @param pageNumber Filter by page number. (optional, default to 1)
* @param offset Entry id at which to start pagination. If the ordering parameter is set to -id, the elements returned precede the identifier. Otherwise, newer elements with respect to the id are returned. (optional)
* @param order Sort responses in ascending or descending order based on the collection property set on the param ``orderBy``. If the request does not specify ``orderBy``, REST returns the collection ordered by id. (optional, default to desc)
* @return HashLockPage
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public HashLockPage searchHashLock(String address, Integer pageSize, Integer pageNumber, String offset, Order order) throws ApiException {
return searchHashLockWithHttpInfo(address, pageSize, pageNumber, offset, order).getData();
}
/**
* Search hash lock entries
* Returns an array of hash locks.
* @param address Filter by address involved in the transaction. An account's address is considered to be involved in the transaction when the account is the sender, recipient, or it is required to cosign the transaction. This filter cannot be combined with ``recipientAddress`` and ``signerPublicKey`` query params. (optional)
* @param pageSize Select the number of entries to return. (optional, default to 10)
* @param pageNumber Filter by page number. (optional, default to 1)
* @param offset Entry id at which to start pagination. If the ordering parameter is set to -id, the elements returned precede the identifier. Otherwise, newer elements with respect to the id are returned. (optional)
* @param order Sort responses in ascending or descending order based on the collection property set on the param ``orderBy``. If the request does not specify ``orderBy``, REST returns the collection ordered by id. (optional, default to desc)
* @return ApiResponse<HashLockPage>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse searchHashLockWithHttpInfo(String address, Integer pageSize, Integer pageNumber, String offset, Order order) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/lock/hash";
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "address", address));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pageSize", pageSize));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pageNumber", pageNumber));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "offset", offset));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "order", order));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("HashLockRoutesApi.searchHashLock", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy