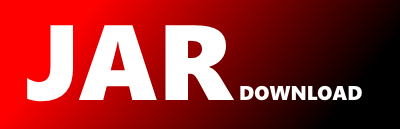
io.nem.symbol.sdk.openapi.jersey2.api.MetadataRoutesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-openapi-jersey2-client Show documentation
Show all versions of symbol-openapi-jersey2-client Show documentation
symbol-openapi-jersey2-client Generated Open API client for symbol-sdk-java
package io.nem.symbol.sdk.openapi.jersey2.api;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiException;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiClient;
import io.nem.symbol.sdk.openapi.jersey2.invoker.ApiResponse;
import io.nem.symbol.sdk.openapi.jersey2.invoker.Configuration;
import io.nem.symbol.sdk.openapi.jersey2.invoker.Pair;
import javax.ws.rs.core.GenericType;
import io.nem.symbol.sdk.openapi.jersey2.model.MerkleStateInfoDTO;
import io.nem.symbol.sdk.openapi.jersey2.model.MetadataInfoDTO;
import io.nem.symbol.sdk.openapi.jersey2.model.MetadataPage;
import io.nem.symbol.sdk.openapi.jersey2.model.MetadataTypeEnum;
import io.nem.symbol.sdk.openapi.jersey2.model.ModelError;
import io.nem.symbol.sdk.openapi.jersey2.model.Order;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2021-02-02T19:39:53.661Z[UTC]")
public class MetadataRoutesApi {
private ApiClient apiClient;
public MetadataRoutesApi() {
this(Configuration.getDefaultApiClient());
}
public MetadataRoutesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get metadata information
* Gets the metadata for a given composite hash.
* @param compositeHash Filter by composite hash. (required)
* @return MetadataInfoDTO
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public MetadataInfoDTO getMetadata(String compositeHash) throws ApiException {
return getMetadataWithHttpInfo(compositeHash).getData();
}
/**
* Get metadata information
* Gets the metadata for a given composite hash.
* @param compositeHash Filter by composite hash. (required)
* @return ApiResponse<MetadataInfoDTO>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse getMetadataWithHttpInfo(String compositeHash) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'compositeHash' is set
if (compositeHash == null) {
throw new ApiException(400, "Missing the required parameter 'compositeHash' when calling getMetadata");
}
// create path and map variables
String localVarPath = "/metadata/{compositeHash}"
.replaceAll("\\{" + "compositeHash" + "\\}", apiClient.escapeString(compositeHash.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("MetadataRoutesApi.getMetadata", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get metadata merkle information
* Gets the metadata merkle for a given composite hash.
* @param compositeHash Filter by composite hash. (required)
* @return MerkleStateInfoDTO
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public MerkleStateInfoDTO getMetadataMerkle(String compositeHash) throws ApiException {
return getMetadataMerkleWithHttpInfo(compositeHash).getData();
}
/**
* Get metadata merkle information
* Gets the metadata merkle for a given composite hash.
* @param compositeHash Filter by composite hash. (required)
* @return ApiResponse<MerkleStateInfoDTO>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse getMetadataMerkleWithHttpInfo(String compositeHash) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'compositeHash' is set
if (compositeHash == null) {
throw new ApiException(400, "Missing the required parameter 'compositeHash' when calling getMetadataMerkle");
}
// create path and map variables
String localVarPath = "/metadata/{compositeHash}/merkle"
.replaceAll("\\{" + "compositeHash" + "\\}", apiClient.escapeString(compositeHash.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("MetadataRoutesApi.getMetadataMerkle", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Search metadata entries
* Returns an array of metadata.
* @param sourceAddress Filter by address sending the metadata entry. (optional)
* @param targetAddress Filter by target address. (optional)
* @param scopedMetadataKey Filter by metadata key. (optional)
* @param targetId Filter by namespace or mosaic id. (optional)
* @param metadataType Filter by metadata type. (optional)
* @param pageSize Select the number of entries to return. (optional, default to 10)
* @param pageNumber Filter by page number. (optional, default to 1)
* @param offset Entry id at which to start pagination. If the ordering parameter is set to -id, the elements returned precede the identifier. Otherwise, newer elements with respect to the id are returned. (optional)
* @param order Sort responses in ascending or descending order based on the collection property set on the param ``orderBy``. If the request does not specify ``orderBy``, REST returns the collection ordered by id. (optional, default to desc)
* @return MetadataPage
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public MetadataPage searchMetadataEntries(String sourceAddress, String targetAddress, String scopedMetadataKey, String targetId, MetadataTypeEnum metadataType, Integer pageSize, Integer pageNumber, String offset, Order order) throws ApiException {
return searchMetadataEntriesWithHttpInfo(sourceAddress, targetAddress, scopedMetadataKey, targetId, metadataType, pageSize, pageNumber, offset, order).getData();
}
/**
* Search metadata entries
* Returns an array of metadata.
* @param sourceAddress Filter by address sending the metadata entry. (optional)
* @param targetAddress Filter by target address. (optional)
* @param scopedMetadataKey Filter by metadata key. (optional)
* @param targetId Filter by namespace or mosaic id. (optional)
* @param metadataType Filter by metadata type. (optional)
* @param pageSize Select the number of entries to return. (optional, default to 10)
* @param pageNumber Filter by page number. (optional, default to 1)
* @param offset Entry id at which to start pagination. If the ordering parameter is set to -id, the elements returned precede the identifier. Otherwise, newer elements with respect to the id are returned. (optional)
* @param order Sort responses in ascending or descending order based on the collection property set on the param ``orderBy``. If the request does not specify ``orderBy``, REST returns the collection ordered by id. (optional, default to desc)
* @return ApiResponse<MetadataPage>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 success -
409 InvalidArgument -
*/
public ApiResponse searchMetadataEntriesWithHttpInfo(String sourceAddress, String targetAddress, String scopedMetadataKey, String targetId, MetadataTypeEnum metadataType, Integer pageSize, Integer pageNumber, String offset, Order order) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/metadata";
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sourceAddress", sourceAddress));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "targetAddress", targetAddress));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "scopedMetadataKey", scopedMetadataKey));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "targetId", targetId));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "metadataType", metadataType));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pageSize", pageSize));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "pageNumber", pageNumber));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "offset", offset));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "order", order));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI("MetadataRoutesApi.searchMetadataEntries", localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy