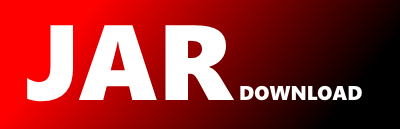
io.nem.symbol.sdk.openapi.jersey2.model.AggregateNetworkPropertiesDTO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-openapi-jersey2-client Show documentation
Show all versions of symbol-openapi-jersey2-client Show documentation
symbol-openapi-jersey2-client Generated Open API client for symbol-sdk-java
/*
* Catapult REST Endpoints
* OpenAPI Specification of catapult-rest 2.3.0
*
* The version of the OpenAPI document: 0.11.2
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.nem.symbol.sdk.openapi.jersey2.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* AggregateNetworkPropertiesDTO
*/
@JsonPropertyOrder({
AggregateNetworkPropertiesDTO.JSON_PROPERTY_MAX_TRANSACTIONS_PER_AGGREGATE,
AggregateNetworkPropertiesDTO.JSON_PROPERTY_MAX_COSIGNATURES_PER_AGGREGATE,
AggregateNetworkPropertiesDTO.JSON_PROPERTY_ENABLE_STRICT_COSIGNATURE_CHECK,
AggregateNetworkPropertiesDTO.JSON_PROPERTY_ENABLE_BONDED_AGGREGATE_SUPPORT,
AggregateNetworkPropertiesDTO.JSON_PROPERTY_MAX_BONDED_TRANSACTION_LIFETIME
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2021-02-02T19:39:53.661Z[UTC]")
public class AggregateNetworkPropertiesDTO {
public static final String JSON_PROPERTY_MAX_TRANSACTIONS_PER_AGGREGATE = "maxTransactionsPerAggregate";
private String maxTransactionsPerAggregate;
public static final String JSON_PROPERTY_MAX_COSIGNATURES_PER_AGGREGATE = "maxCosignaturesPerAggregate";
private String maxCosignaturesPerAggregate;
public static final String JSON_PROPERTY_ENABLE_STRICT_COSIGNATURE_CHECK = "enableStrictCosignatureCheck";
private Boolean enableStrictCosignatureCheck;
public static final String JSON_PROPERTY_ENABLE_BONDED_AGGREGATE_SUPPORT = "enableBondedAggregateSupport";
private Boolean enableBondedAggregateSupport;
public static final String JSON_PROPERTY_MAX_BONDED_TRANSACTION_LIFETIME = "maxBondedTransactionLifetime";
private String maxBondedTransactionLifetime;
public AggregateNetworkPropertiesDTO maxTransactionsPerAggregate(String maxTransactionsPerAggregate) {
this.maxTransactionsPerAggregate = maxTransactionsPerAggregate;
return this;
}
/**
* Maximum number of transactions per aggregate.
* @return maxTransactionsPerAggregate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1'000", value = "Maximum number of transactions per aggregate.")
@JsonProperty(JSON_PROPERTY_MAX_TRANSACTIONS_PER_AGGREGATE)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxTransactionsPerAggregate() {
return maxTransactionsPerAggregate;
}
public void setMaxTransactionsPerAggregate(String maxTransactionsPerAggregate) {
this.maxTransactionsPerAggregate = maxTransactionsPerAggregate;
}
public AggregateNetworkPropertiesDTO maxCosignaturesPerAggregate(String maxCosignaturesPerAggregate) {
this.maxCosignaturesPerAggregate = maxCosignaturesPerAggregate;
return this;
}
/**
* Maximum number of cosignatures per aggregate.
* @return maxCosignaturesPerAggregate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "15", value = "Maximum number of cosignatures per aggregate.")
@JsonProperty(JSON_PROPERTY_MAX_COSIGNATURES_PER_AGGREGATE)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxCosignaturesPerAggregate() {
return maxCosignaturesPerAggregate;
}
public void setMaxCosignaturesPerAggregate(String maxCosignaturesPerAggregate) {
this.maxCosignaturesPerAggregate = maxCosignaturesPerAggregate;
}
public AggregateNetworkPropertiesDTO enableStrictCosignatureCheck(Boolean enableStrictCosignatureCheck) {
this.enableStrictCosignatureCheck = enableStrictCosignatureCheck;
return this;
}
/**
* Set to true if cosignatures must exactly match component signers. Set to false if cosignatures should be validated externally.
* @return enableStrictCosignatureCheck
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Set to true if cosignatures must exactly match component signers. Set to false if cosignatures should be validated externally.")
@JsonProperty(JSON_PROPERTY_ENABLE_STRICT_COSIGNATURE_CHECK)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getEnableStrictCosignatureCheck() {
return enableStrictCosignatureCheck;
}
public void setEnableStrictCosignatureCheck(Boolean enableStrictCosignatureCheck) {
this.enableStrictCosignatureCheck = enableStrictCosignatureCheck;
}
public AggregateNetworkPropertiesDTO enableBondedAggregateSupport(Boolean enableBondedAggregateSupport) {
this.enableBondedAggregateSupport = enableBondedAggregateSupport;
return this;
}
/**
* Set to true if bonded aggregates should be allowed. Set to false if bonded aggregates should be rejected.
* @return enableBondedAggregateSupport
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "true", value = "Set to true if bonded aggregates should be allowed. Set to false if bonded aggregates should be rejected.")
@JsonProperty(JSON_PROPERTY_ENABLE_BONDED_AGGREGATE_SUPPORT)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getEnableBondedAggregateSupport() {
return enableBondedAggregateSupport;
}
public void setEnableBondedAggregateSupport(Boolean enableBondedAggregateSupport) {
this.enableBondedAggregateSupport = enableBondedAggregateSupport;
}
public AggregateNetworkPropertiesDTO maxBondedTransactionLifetime(String maxBondedTransactionLifetime) {
this.maxBondedTransactionLifetime = maxBondedTransactionLifetime;
return this;
}
/**
* Maximum lifetime a bonded transaction can have before it expires.
* @return maxBondedTransactionLifetime
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "48h", value = "Maximum lifetime a bonded transaction can have before it expires.")
@JsonProperty(JSON_PROPERTY_MAX_BONDED_TRANSACTION_LIFETIME)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxBondedTransactionLifetime() {
return maxBondedTransactionLifetime;
}
public void setMaxBondedTransactionLifetime(String maxBondedTransactionLifetime) {
this.maxBondedTransactionLifetime = maxBondedTransactionLifetime;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AggregateNetworkPropertiesDTO aggregateNetworkPropertiesDTO = (AggregateNetworkPropertiesDTO) o;
return Objects.equals(this.maxTransactionsPerAggregate, aggregateNetworkPropertiesDTO.maxTransactionsPerAggregate) &&
Objects.equals(this.maxCosignaturesPerAggregate, aggregateNetworkPropertiesDTO.maxCosignaturesPerAggregate) &&
Objects.equals(this.enableStrictCosignatureCheck, aggregateNetworkPropertiesDTO.enableStrictCosignatureCheck) &&
Objects.equals(this.enableBondedAggregateSupport, aggregateNetworkPropertiesDTO.enableBondedAggregateSupport) &&
Objects.equals(this.maxBondedTransactionLifetime, aggregateNetworkPropertiesDTO.maxBondedTransactionLifetime);
}
@Override
public int hashCode() {
return Objects.hash(maxTransactionsPerAggregate, maxCosignaturesPerAggregate, enableStrictCosignatureCheck, enableBondedAggregateSupport, maxBondedTransactionLifetime);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AggregateNetworkPropertiesDTO {\n");
sb.append(" maxTransactionsPerAggregate: ").append(toIndentedString(maxTransactionsPerAggregate)).append("\n");
sb.append(" maxCosignaturesPerAggregate: ").append(toIndentedString(maxCosignaturesPerAggregate)).append("\n");
sb.append(" enableStrictCosignatureCheck: ").append(toIndentedString(enableStrictCosignatureCheck)).append("\n");
sb.append(" enableBondedAggregateSupport: ").append(toIndentedString(enableBondedAggregateSupport)).append("\n");
sb.append(" maxBondedTransactionLifetime: ").append(toIndentedString(maxBondedTransactionLifetime)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy