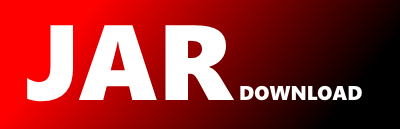
io.nem.symbol.sdk.openapi.jersey2.model.NamespaceNetworkPropertiesDTO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-openapi-jersey2-client Show documentation
Show all versions of symbol-openapi-jersey2-client Show documentation
symbol-openapi-jersey2-client Generated Open API client for symbol-sdk-java
/*
* Catapult REST Endpoints
* OpenAPI Specification of catapult-rest 2.3.0
*
* The version of the OpenAPI document: 0.11.2
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.nem.symbol.sdk.openapi.jersey2.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* NamespaceNetworkPropertiesDTO
*/
@JsonPropertyOrder({
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_MAX_NAME_SIZE,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_MAX_CHILD_NAMESPACES,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_MAX_NAMESPACE_DEPTH,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_MIN_NAMESPACE_DURATION,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_MAX_NAMESPACE_DURATION,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_NAMESPACE_GRACE_PERIOD_DURATION,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_RESERVED_ROOT_NAMESPACE_NAMES,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_NAMESPACE_RENTAL_FEE_SINK_ADDRESS,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_ROOT_NAMESPACE_RENTAL_FEE_PER_BLOCK,
NamespaceNetworkPropertiesDTO.JSON_PROPERTY_CHILD_NAMESPACE_RENTAL_FEE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2021-02-02T19:39:53.661Z[UTC]")
public class NamespaceNetworkPropertiesDTO {
public static final String JSON_PROPERTY_MAX_NAME_SIZE = "maxNameSize";
private String maxNameSize;
public static final String JSON_PROPERTY_MAX_CHILD_NAMESPACES = "maxChildNamespaces";
private String maxChildNamespaces;
public static final String JSON_PROPERTY_MAX_NAMESPACE_DEPTH = "maxNamespaceDepth";
private String maxNamespaceDepth;
public static final String JSON_PROPERTY_MIN_NAMESPACE_DURATION = "minNamespaceDuration";
private String minNamespaceDuration;
public static final String JSON_PROPERTY_MAX_NAMESPACE_DURATION = "maxNamespaceDuration";
private String maxNamespaceDuration;
public static final String JSON_PROPERTY_NAMESPACE_GRACE_PERIOD_DURATION = "namespaceGracePeriodDuration";
private String namespaceGracePeriodDuration;
public static final String JSON_PROPERTY_RESERVED_ROOT_NAMESPACE_NAMES = "reservedRootNamespaceNames";
private String reservedRootNamespaceNames;
public static final String JSON_PROPERTY_NAMESPACE_RENTAL_FEE_SINK_ADDRESS = "namespaceRentalFeeSinkAddress";
private String namespaceRentalFeeSinkAddress;
public static final String JSON_PROPERTY_ROOT_NAMESPACE_RENTAL_FEE_PER_BLOCK = "rootNamespaceRentalFeePerBlock";
private String rootNamespaceRentalFeePerBlock;
public static final String JSON_PROPERTY_CHILD_NAMESPACE_RENTAL_FEE = "childNamespaceRentalFee";
private String childNamespaceRentalFee;
public NamespaceNetworkPropertiesDTO maxNameSize(String maxNameSize) {
this.maxNameSize = maxNameSize;
return this;
}
/**
* Maximum namespace name size.
* @return maxNameSize
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "64", value = "Maximum namespace name size.")
@JsonProperty(JSON_PROPERTY_MAX_NAME_SIZE)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxNameSize() {
return maxNameSize;
}
public void setMaxNameSize(String maxNameSize) {
this.maxNameSize = maxNameSize;
}
public NamespaceNetworkPropertiesDTO maxChildNamespaces(String maxChildNamespaces) {
this.maxChildNamespaces = maxChildNamespaces;
return this;
}
/**
* Maximum number of children for a root namespace.
* @return maxChildNamespaces
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "500", value = "Maximum number of children for a root namespace.")
@JsonProperty(JSON_PROPERTY_MAX_CHILD_NAMESPACES)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxChildNamespaces() {
return maxChildNamespaces;
}
public void setMaxChildNamespaces(String maxChildNamespaces) {
this.maxChildNamespaces = maxChildNamespaces;
}
public NamespaceNetworkPropertiesDTO maxNamespaceDepth(String maxNamespaceDepth) {
this.maxNamespaceDepth = maxNamespaceDepth;
return this;
}
/**
* Maximum namespace depth.
* @return maxNamespaceDepth
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "3", value = "Maximum namespace depth.")
@JsonProperty(JSON_PROPERTY_MAX_NAMESPACE_DEPTH)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxNamespaceDepth() {
return maxNamespaceDepth;
}
public void setMaxNamespaceDepth(String maxNamespaceDepth) {
this.maxNamespaceDepth = maxNamespaceDepth;
}
public NamespaceNetworkPropertiesDTO minNamespaceDuration(String minNamespaceDuration) {
this.minNamespaceDuration = minNamespaceDuration;
return this;
}
/**
* Minimum namespace duration.
* @return minNamespaceDuration
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1m", value = "Minimum namespace duration.")
@JsonProperty(JSON_PROPERTY_MIN_NAMESPACE_DURATION)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMinNamespaceDuration() {
return minNamespaceDuration;
}
public void setMinNamespaceDuration(String minNamespaceDuration) {
this.minNamespaceDuration = minNamespaceDuration;
}
public NamespaceNetworkPropertiesDTO maxNamespaceDuration(String maxNamespaceDuration) {
this.maxNamespaceDuration = maxNamespaceDuration;
return this;
}
/**
* Maximum namespace duration.
* @return maxNamespaceDuration
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "365d", value = "Maximum namespace duration.")
@JsonProperty(JSON_PROPERTY_MAX_NAMESPACE_DURATION)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMaxNamespaceDuration() {
return maxNamespaceDuration;
}
public void setMaxNamespaceDuration(String maxNamespaceDuration) {
this.maxNamespaceDuration = maxNamespaceDuration;
}
public NamespaceNetworkPropertiesDTO namespaceGracePeriodDuration(String namespaceGracePeriodDuration) {
this.namespaceGracePeriodDuration = namespaceGracePeriodDuration;
return this;
}
/**
* Grace period during which time only the previous owner can renew an expired namespace.
* @return namespaceGracePeriodDuration
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2m", value = "Grace period during which time only the previous owner can renew an expired namespace.")
@JsonProperty(JSON_PROPERTY_NAMESPACE_GRACE_PERIOD_DURATION)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNamespaceGracePeriodDuration() {
return namespaceGracePeriodDuration;
}
public void setNamespaceGracePeriodDuration(String namespaceGracePeriodDuration) {
this.namespaceGracePeriodDuration = namespaceGracePeriodDuration;
}
public NamespaceNetworkPropertiesDTO reservedRootNamespaceNames(String reservedRootNamespaceNames) {
this.reservedRootNamespaceNames = reservedRootNamespaceNames;
return this;
}
/**
* Reserved root namespaces that cannot be claimed.
* @return reservedRootNamespaceNames
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "xem, nem, user, account, org, com, biz, net, edu, mil, gov, info", value = "Reserved root namespaces that cannot be claimed.")
@JsonProperty(JSON_PROPERTY_RESERVED_ROOT_NAMESPACE_NAMES)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReservedRootNamespaceNames() {
return reservedRootNamespaceNames;
}
public void setReservedRootNamespaceNames(String reservedRootNamespaceNames) {
this.reservedRootNamespaceNames = reservedRootNamespaceNames;
}
public NamespaceNetworkPropertiesDTO namespaceRentalFeeSinkAddress(String namespaceRentalFeeSinkAddress) {
this.namespaceRentalFeeSinkAddress = namespaceRentalFeeSinkAddress;
return this;
}
/**
* Address encoded using a 32-character set.
* @return namespaceRentalFeeSinkAddress
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "SAAA244WMCB2JXGNQTQHQOS45TGBFF4V2MJBVOQ", value = "Address encoded using a 32-character set.")
@JsonProperty(JSON_PROPERTY_NAMESPACE_RENTAL_FEE_SINK_ADDRESS)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNamespaceRentalFeeSinkAddress() {
return namespaceRentalFeeSinkAddress;
}
public void setNamespaceRentalFeeSinkAddress(String namespaceRentalFeeSinkAddress) {
this.namespaceRentalFeeSinkAddress = namespaceRentalFeeSinkAddress;
}
public NamespaceNetworkPropertiesDTO rootNamespaceRentalFeePerBlock(String rootNamespaceRentalFeePerBlock) {
this.rootNamespaceRentalFeePerBlock = rootNamespaceRentalFeePerBlock;
return this;
}
/**
* Root namespace rental fee per block.
* @return rootNamespaceRentalFeePerBlock
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1", value = "Root namespace rental fee per block.")
@JsonProperty(JSON_PROPERTY_ROOT_NAMESPACE_RENTAL_FEE_PER_BLOCK)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getRootNamespaceRentalFeePerBlock() {
return rootNamespaceRentalFeePerBlock;
}
public void setRootNamespaceRentalFeePerBlock(String rootNamespaceRentalFeePerBlock) {
this.rootNamespaceRentalFeePerBlock = rootNamespaceRentalFeePerBlock;
}
public NamespaceNetworkPropertiesDTO childNamespaceRentalFee(String childNamespaceRentalFee) {
this.childNamespaceRentalFee = childNamespaceRentalFee;
return this;
}
/**
* Child namespace rental fee.
* @return childNamespaceRentalFee
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "100", value = "Child namespace rental fee.")
@JsonProperty(JSON_PROPERTY_CHILD_NAMESPACE_RENTAL_FEE)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getChildNamespaceRentalFee() {
return childNamespaceRentalFee;
}
public void setChildNamespaceRentalFee(String childNamespaceRentalFee) {
this.childNamespaceRentalFee = childNamespaceRentalFee;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NamespaceNetworkPropertiesDTO namespaceNetworkPropertiesDTO = (NamespaceNetworkPropertiesDTO) o;
return Objects.equals(this.maxNameSize, namespaceNetworkPropertiesDTO.maxNameSize) &&
Objects.equals(this.maxChildNamespaces, namespaceNetworkPropertiesDTO.maxChildNamespaces) &&
Objects.equals(this.maxNamespaceDepth, namespaceNetworkPropertiesDTO.maxNamespaceDepth) &&
Objects.equals(this.minNamespaceDuration, namespaceNetworkPropertiesDTO.minNamespaceDuration) &&
Objects.equals(this.maxNamespaceDuration, namespaceNetworkPropertiesDTO.maxNamespaceDuration) &&
Objects.equals(this.namespaceGracePeriodDuration, namespaceNetworkPropertiesDTO.namespaceGracePeriodDuration) &&
Objects.equals(this.reservedRootNamespaceNames, namespaceNetworkPropertiesDTO.reservedRootNamespaceNames) &&
Objects.equals(this.namespaceRentalFeeSinkAddress, namespaceNetworkPropertiesDTO.namespaceRentalFeeSinkAddress) &&
Objects.equals(this.rootNamespaceRentalFeePerBlock, namespaceNetworkPropertiesDTO.rootNamespaceRentalFeePerBlock) &&
Objects.equals(this.childNamespaceRentalFee, namespaceNetworkPropertiesDTO.childNamespaceRentalFee);
}
@Override
public int hashCode() {
return Objects.hash(maxNameSize, maxChildNamespaces, maxNamespaceDepth, minNamespaceDuration, maxNamespaceDuration, namespaceGracePeriodDuration, reservedRootNamespaceNames, namespaceRentalFeeSinkAddress, rootNamespaceRentalFeePerBlock, childNamespaceRentalFee);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class NamespaceNetworkPropertiesDTO {\n");
sb.append(" maxNameSize: ").append(toIndentedString(maxNameSize)).append("\n");
sb.append(" maxChildNamespaces: ").append(toIndentedString(maxChildNamespaces)).append("\n");
sb.append(" maxNamespaceDepth: ").append(toIndentedString(maxNamespaceDepth)).append("\n");
sb.append(" minNamespaceDuration: ").append(toIndentedString(minNamespaceDuration)).append("\n");
sb.append(" maxNamespaceDuration: ").append(toIndentedString(maxNamespaceDuration)).append("\n");
sb.append(" namespaceGracePeriodDuration: ").append(toIndentedString(namespaceGracePeriodDuration)).append("\n");
sb.append(" reservedRootNamespaceNames: ").append(toIndentedString(reservedRootNamespaceNames)).append("\n");
sb.append(" namespaceRentalFeeSinkAddress: ").append(toIndentedString(namespaceRentalFeeSinkAddress)).append("\n");
sb.append(" rootNamespaceRentalFeePerBlock: ").append(toIndentedString(rootNamespaceRentalFeePerBlock)).append("\n");
sb.append(" childNamespaceRentalFee: ").append(toIndentedString(childNamespaceRentalFee)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy