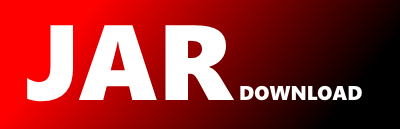
io.nem.symbol.sdk.openapi.jersey2.model.NetworkPropertiesDTO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-openapi-jersey2-client Show documentation
Show all versions of symbol-openapi-jersey2-client Show documentation
symbol-openapi-jersey2-client Generated Open API client for symbol-sdk-java
/*
* Catapult REST Endpoints
* OpenAPI Specification of catapult-rest 2.3.0
*
* The version of the OpenAPI document: 0.11.2
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.nem.symbol.sdk.openapi.jersey2.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.nem.symbol.sdk.openapi.jersey2.model.NodeIdentityEqualityStrategy;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* Network related configuration properties.
*/
@ApiModel(description = "Network related configuration properties.")
@JsonPropertyOrder({
NetworkPropertiesDTO.JSON_PROPERTY_IDENTIFIER,
NetworkPropertiesDTO.JSON_PROPERTY_NODE_EQUALITY_STRATEGY,
NetworkPropertiesDTO.JSON_PROPERTY_NEMESIS_SIGNER_PUBLIC_KEY,
NetworkPropertiesDTO.JSON_PROPERTY_GENERATION_HASH_SEED,
NetworkPropertiesDTO.JSON_PROPERTY_EPOCH_ADJUSTMENT
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2021-02-02T19:39:53.661Z[UTC]")
public class NetworkPropertiesDTO {
public static final String JSON_PROPERTY_IDENTIFIER = "identifier";
private String identifier;
public static final String JSON_PROPERTY_NODE_EQUALITY_STRATEGY = "nodeEqualityStrategy";
private NodeIdentityEqualityStrategy nodeEqualityStrategy;
public static final String JSON_PROPERTY_NEMESIS_SIGNER_PUBLIC_KEY = "nemesisSignerPublicKey";
private String nemesisSignerPublicKey;
public static final String JSON_PROPERTY_GENERATION_HASH_SEED = "generationHashSeed";
private String generationHashSeed;
public static final String JSON_PROPERTY_EPOCH_ADJUSTMENT = "epochAdjustment";
private String epochAdjustment;
public NetworkPropertiesDTO identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* Network identifier.
* @return identifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "mijin-test", value = "Network identifier.")
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public NetworkPropertiesDTO nodeEqualityStrategy(NodeIdentityEqualityStrategy nodeEqualityStrategy) {
this.nodeEqualityStrategy = nodeEqualityStrategy;
return this;
}
/**
* Get nodeEqualityStrategy
* @return nodeEqualityStrategy
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NODE_EQUALITY_STRATEGY)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public NodeIdentityEqualityStrategy getNodeEqualityStrategy() {
return nodeEqualityStrategy;
}
public void setNodeEqualityStrategy(NodeIdentityEqualityStrategy nodeEqualityStrategy) {
this.nodeEqualityStrategy = nodeEqualityStrategy;
}
public NetworkPropertiesDTO nemesisSignerPublicKey(String nemesisSignerPublicKey) {
this.nemesisSignerPublicKey = nemesisSignerPublicKey;
return this;
}
/**
* Public key.
* @return nemesisSignerPublicKey
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "AC1A6E1D8DE5B17D2C6B1293F1CAD3829EEACF38D09311BB3C8E5A880092DE26", value = "Public key.")
@JsonProperty(JSON_PROPERTY_NEMESIS_SIGNER_PUBLIC_KEY)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getNemesisSignerPublicKey() {
return nemesisSignerPublicKey;
}
public void setNemesisSignerPublicKey(String nemesisSignerPublicKey) {
this.nemesisSignerPublicKey = nemesisSignerPublicKey;
}
public NetworkPropertiesDTO generationHashSeed(String generationHashSeed) {
this.generationHashSeed = generationHashSeed;
return this;
}
/**
* Get generationHashSeed
* @return generationHashSeed
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "C8FC3FB54FDDFBCE0E8C71224990124E4EEC5AD5D30E592EDFA9524669A23810", value = "")
@JsonProperty(JSON_PROPERTY_GENERATION_HASH_SEED)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getGenerationHashSeed() {
return generationHashSeed;
}
public void setGenerationHashSeed(String generationHashSeed) {
this.generationHashSeed = generationHashSeed;
}
public NetworkPropertiesDTO epochAdjustment(String epochAdjustment) {
this.epochAdjustment = epochAdjustment;
return this;
}
/**
* Nemesis epoch time adjustment.
* @return epochAdjustment
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1573430400s", value = "Nemesis epoch time adjustment.")
@JsonProperty(JSON_PROPERTY_EPOCH_ADJUSTMENT)
// @JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getEpochAdjustment() {
return epochAdjustment;
}
public void setEpochAdjustment(String epochAdjustment) {
this.epochAdjustment = epochAdjustment;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NetworkPropertiesDTO networkPropertiesDTO = (NetworkPropertiesDTO) o;
return Objects.equals(this.identifier, networkPropertiesDTO.identifier) &&
Objects.equals(this.nodeEqualityStrategy, networkPropertiesDTO.nodeEqualityStrategy) &&
Objects.equals(this.nemesisSignerPublicKey, networkPropertiesDTO.nemesisSignerPublicKey) &&
Objects.equals(this.generationHashSeed, networkPropertiesDTO.generationHashSeed) &&
Objects.equals(this.epochAdjustment, networkPropertiesDTO.epochAdjustment);
}
@Override
public int hashCode() {
return Objects.hash(identifier, nodeEqualityStrategy, nemesisSignerPublicKey, generationHashSeed, epochAdjustment);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class NetworkPropertiesDTO {\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" nodeEqualityStrategy: ").append(toIndentedString(nodeEqualityStrategy)).append("\n");
sb.append(" nemesisSignerPublicKey: ").append(toIndentedString(nemesisSignerPublicKey)).append("\n");
sb.append(" generationHashSeed: ").append(toIndentedString(generationHashSeed)).append("\n");
sb.append(" epochAdjustment: ").append(toIndentedString(epochAdjustment)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy