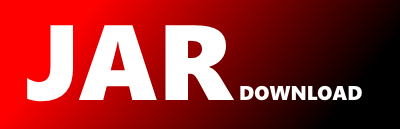
io.nem.symbol.sdk.infrastructure.vertx.MetadataRepositoryVertxImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of symbol-sdk-vertx-client Show documentation
Show all versions of symbol-sdk-vertx-client Show documentation
symbol-sdk-vertx-client lib for Sybol SDK Java
/*
* Copyright 2020 NEM
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.nem.symbol.sdk.infrastructure.vertx;
import io.nem.symbol.core.utils.ConvertUtils;
import io.nem.symbol.core.utils.MapperUtils;
import io.nem.symbol.sdk.api.MetadataRepository;
import io.nem.symbol.sdk.api.QueryParams;
import io.nem.symbol.sdk.model.account.Address;
import io.nem.symbol.sdk.model.metadata.Metadata;
import io.nem.symbol.sdk.model.metadata.MetadataEntry;
import io.nem.symbol.sdk.model.metadata.MetadataType;
import io.nem.symbol.sdk.model.mosaic.MosaicId;
import io.nem.symbol.sdk.model.namespace.NamespaceId;
import io.nem.symbol.sdk.openapi.vertx.api.MetadataRoutesApi;
import io.nem.symbol.sdk.openapi.vertx.api.MetadataRoutesApiImpl;
import io.nem.symbol.sdk.openapi.vertx.invoker.ApiClient;
import io.nem.symbol.sdk.openapi.vertx.model.MetadataDTO;
import io.nem.symbol.sdk.openapi.vertx.model.MetadataEntriesDTO;
import io.nem.symbol.sdk.openapi.vertx.model.MetadataEntryDTO;
import io.reactivex.Observable;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import java.math.BigInteger;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
/**
* Implementation of {@link MetadataRepository}
*/
public class MetadataRepositoryVertxImpl extends AbstractRepositoryVertxImpl implements
MetadataRepository {
private final MetadataRoutesApi client;
public MetadataRepositoryVertxImpl(ApiClient apiClient) {
super(apiClient);
client = new MetadataRoutesApiImpl(apiClient);
}
@Override
public Observable> getAccountMetadata(Address targetAddress,
Optional queryParams) {
Consumer>> callback = handler -> getClient()
.getAccountMetadata(targetAddress.plain(), getPageSize(queryParams),
getOrder(queryParams), getId(queryParams),
handler);
return handleList(callback);
}
@Override
public Observable> getAccountMetadataByKey(Address targetAddress,
BigInteger key) {
Consumer>> callback = handler -> getClient()
.getAccountMetadataByKey(targetAddress.plain(), toHex(key), handler);
return handleList(callback);
}
@Override
public Observable> getMosaicMetadata(MosaicId targetMosaicId,
Optional queryParams) {
Consumer>> callback = handler -> getClient()
.getMosaicMetadata(targetMosaicId.getIdAsHex(), getPageSize(queryParams),
getId(queryParams),
getOrder(queryParams),
handler);
return handleList(callback);
}
@Override
public Observable> getMosaicMetadataByKey(MosaicId targetMosaicId,
BigInteger key) {
Consumer>> callback = handler -> getClient()
.getMosaicMetadataByKey(targetMosaicId.getIdAsHex(), toHex(key), handler);
return handleList(callback);
}
@Override
public Observable getAccountMetadataByKeyAndSender(Address targetAddress,
BigInteger key, Address sourceAddress) {
Consumer>> callback = handler -> getClient()
.getAccountMetadataByKeyAndSender(targetAddress.plain(), toHex(key), sourceAddress.plain(),
handler);
return handleOne(callback);
}
@Override
public Observable getMosaicMetadataByKeyAndSender(MosaicId targetMosaicId,
BigInteger key, Address sourceAddress) {
Consumer>> callback = handler -> getClient()
.getMosaicMetadataByKeyAndSender(targetMosaicId.getIdAsHex(), toHex(key),
sourceAddress.plain(), handler);
return handleOne(callback);
}
@Override
public Observable> getNamespaceMetadata(NamespaceId targetNamespaceId,
Optional queryParams) {
Consumer>> callback = handler -> getClient()
.getNamespaceMetadata(targetNamespaceId.getIdAsHex(), getPageSize(queryParams),
getId(queryParams),
getOrder(queryParams),
handler);
return handleList(callback);
}
@Override
public Observable> getNamespaceMetadataByKey(NamespaceId targetNamespaceId,
BigInteger key) {
Consumer>> callback = handler -> getClient()
.getNamespaceMetadataByKey(targetNamespaceId.getIdAsHex(), toHex(key), handler);
return handleList(callback);
}
@Override
public Observable getNamespaceMetadataByKeyAndSender(NamespaceId targetNamespaceId,
BigInteger key, Address sourceAddress) {
Consumer>> callback = handler -> getClient()
.getNamespaceMetadataByKeyAndSender(targetNamespaceId.getIdAsHex(), toHex(key),
sourceAddress.plain(), handler);
return handleOne(callback);
}
public MetadataRoutesApi getClient() {
return client;
}
/**
* It handles an async call result of a list of {@link MetadataEntriesDTO} converting it into a {@link Observable}
* list of {@link Metadata}.
*
* @param callback the callback
* @return the {@link Observable} list of {@link Metadata}.
*/
private Observable> handleList(
Consumer>> callback) {
return exceptionHandling(
call(callback).map(MetadataEntriesDTO::getMetadataEntries).flatMapIterable(item -> item)
.map(this::toMetadata).toList()
.toObservable());
}
/**
* It handles an async call result of a {@link MetadataEntriesDTO} converting it into a {@link Observable} of {@link
* Metadata}.
*
* @param callback the callback
* @return the {@link Observable} of {@link Metadata}.
*/
private Observable handleOne(
Consumer>> callback) {
return exceptionHandling(call(callback)
.map(this::toMetadata));
}
/**
* It converts the {@link MetadataDTO} into a model {@link Metadata}.
*
* @param dto the {@link MetadataDTO}
* @return the {@link Metadata}
*/
private Metadata toMetadata(MetadataDTO dto) {
MetadataEntryDTO entryDto = dto.getMetadataEntry();
MetadataEntry metadataEntry = new MetadataEntry(entryDto.getCompositeHash(),
MapperUtils.toAddress(entryDto.getSourceAddress()),
MapperUtils.toAddress(entryDto.getTargetAddress()),
new BigInteger(entryDto.getScopedMetadataKey(), 16),
MetadataType.rawValueOf(entryDto.getMetadataType().getValue()),
ConvertUtils.fromHexToString(entryDto.getValue()),
Optional.ofNullable(Objects.toString(entryDto.getTargetId(), null)));
return new Metadata(dto.getId(), metadataEntry);
}
protected String toHex(BigInteger key) {
return ConvertUtils.toSize16Hex(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy