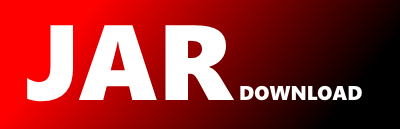
io.neow3j.protocol.core.Request Maven / Gradle / Ivy
package io.neow3j.protocol.core;
import io.neow3j.protocol.Neow3jService;
import rx.Observable;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicLong;
public class Request {
private static AtomicLong nextId = new AtomicLong(0);
private String jsonrpc = "2.0";
private String method;
private List params;
private long id;
private Neow3jService neow3jService;
// Unfortunately require an instance of the type too, see
// http://stackoverflow.com/a/3437930/3211687
private Class responseType;
public Request() {
}
public Request(String method, List params,
Neow3jService neow3jService, Class type) {
this.method = method;
this.params = params;
this.id = nextId.getAndIncrement();
this.neow3jService = neow3jService;
this.responseType = type;
}
public String getJsonrpc() {
return jsonrpc;
}
public void setJsonrpc(String jsonrpc) {
this.jsonrpc = jsonrpc;
}
public String getMethod() {
return method;
}
public void setMethod(String method) {
this.method = method;
}
public List getParams() {
return params;
}
public void setParams(List params) {
this.params = params;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public T send() throws IOException {
return neow3jService.send(this, responseType);
}
public CompletableFuture sendAsync() {
return neow3jService.sendAsync(this, responseType);
}
public Observable observable() {
return new RemoteCall<>(this::send).observable();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy