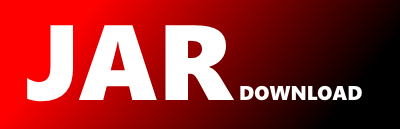
io.neow3j.protocol.Service Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
neow3j: Java/Kotlin/Android Development Toolkit for the Neo Blockchain
package io.neow3j.protocol;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.neow3j.protocol.core.Request;
import io.neow3j.protocol.core.Response;
import io.neow3j.protocol.notifications.Notification;
import io.neow3j.utils.Async;
import io.reactivex.Observable;
import java.io.IOException;
import java.io.InputStream;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutorService;
/**
* Base service implementation.
*/
public abstract class Service implements Neow3jService {
protected final ObjectMapper objectMapper;
protected ExecutorService asyncExecutorService;
/**
* Create a Service.
*
* @param executorService an external ExecutorService where {@link Request} calls should run.
* @param includeRawResponses option to include or not raw responses on the {@link Response} object.
*/
public Service(ExecutorService executorService, boolean includeRawResponses) {
objectMapper = ObjectMapperFactory.getObjectMapper(includeRawResponses);
asyncExecutorService = executorService;
}
/**
* Create a Service.
*
* @param includeRawResponses option to include or not raw responses on the {@link Response} object.
*/
public Service(boolean includeRawResponses) {
objectMapper = ObjectMapperFactory.getObjectMapper(includeRawResponses);
}
protected abstract InputStream performIO(String payload) throws IOException;
@Override
public T send(
Request request, Class responseType) throws IOException {
String payload = objectMapper.writeValueAsString(request);
try (InputStream result = performIO(payload)) {
if (result != null) {
return objectMapper.readValue(result, responseType);
} else {
return null;
}
}
}
@Override
public CompletableFuture sendAsync(
Request jsonRpc20Request, Class responseType) {
return Async.run(() ->
send(jsonRpc20Request, responseType), asyncExecutorService);
}
@Override
public > Observable subscribe(
Request request, String unsubscribeMethod, Class responseType) {
throw new UnsupportedOperationException(
String.format("Service %s does not support subscriptions", this.getClass()
.getSimpleName()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy