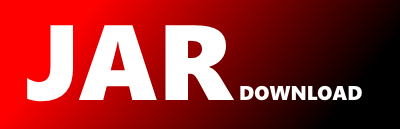
io.netty.incubator.codec.http3.Http3UnidirectionalStreamInboundHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netty-incubator-codec-http3 Show documentation
Show all versions of netty-incubator-codec-http3 Show documentation
Netty is an asynchronous event-driven network application framework for
rapid development of maintainable high performance protocol servers and
clients.
/*
* Copyright 2020 The Netty Project
*
* The Netty Project licenses this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.netty.incubator.codec.http3;
import io.netty.buffer.ByteBuf;
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandler;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
import io.netty.handler.codec.ByteToMessageDecoder;
import io.netty.incubator.codec.http3.Http3FrameCodec.Http3FrameCodecFactory;
import io.netty.util.AttributeKey;
import io.netty.util.ReferenceCountUtil;
import java.util.List;
import java.util.function.LongFunction;
import java.util.function.Supplier;
import static io.netty.incubator.codec.http3.Http3CodecUtils.HTTP3_CONTROL_STREAM_TYPE;
import static io.netty.incubator.codec.http3.Http3CodecUtils.HTTP3_PUSH_STREAM_TYPE;
import static io.netty.incubator.codec.http3.Http3CodecUtils.HTTP3_QPACK_DECODER_STREAM_TYPE;
import static io.netty.incubator.codec.http3.Http3CodecUtils.HTTP3_QPACK_ENCODER_STREAM_TYPE;
import static io.netty.incubator.codec.http3.Http3RequestStreamCodecState.NO_STATE;
/**
* {@link ByteToMessageDecoder} which helps to detect the type of unidirectional stream.
*/
abstract class Http3UnidirectionalStreamInboundHandler extends ByteToMessageDecoder {
private static final AttributeKey REMOTE_CONTROL_STREAM = AttributeKey.valueOf("H3_REMOTE_CONTROL_STREAM");
private static final AttributeKey REMOTE_QPACK_DECODER_STREAM =
AttributeKey.valueOf("H3_REMOTE_QPACK_DECODER_STREAM");
private static final AttributeKey REMOTE_QPACK_ENCODER_STREAM =
AttributeKey.valueOf("H3_REMOTE_QPACK_ENCODER_STREAM");
final Http3FrameCodecFactory codecFactory;
final Http3ControlStreamInboundHandler localControlStreamHandler;
final Http3ControlStreamOutboundHandler remoteControlStreamHandler;
final Supplier qpackEncoderHandlerFactory;
final Supplier qpackDecoderHandlerFactory;
final LongFunction unknownStreamHandlerFactory;
Http3UnidirectionalStreamInboundHandler(Http3FrameCodecFactory codecFactory,
Http3ControlStreamInboundHandler localControlStreamHandler,
Http3ControlStreamOutboundHandler remoteControlStreamHandler,
LongFunction unknownStreamHandlerFactory,
Supplier qpackEncoderHandlerFactory,
Supplier qpackDecoderHandlerFactory) {
this.codecFactory = codecFactory;
this.localControlStreamHandler = localControlStreamHandler;
this.remoteControlStreamHandler = remoteControlStreamHandler;
this.qpackEncoderHandlerFactory = qpackEncoderHandlerFactory;
this.qpackDecoderHandlerFactory = qpackDecoderHandlerFactory;
if (unknownStreamHandlerFactory == null) {
// If the user did not specify an own factory just drop all bytes on the floor.
unknownStreamHandlerFactory = type -> ReleaseHandler.INSTANCE;
}
this.unknownStreamHandlerFactory = unknownStreamHandlerFactory;
}
@Override
protected void decode(ChannelHandlerContext ctx, ByteBuf in, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy