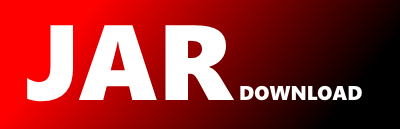
io.netty.incubator.codec.http3.QpackStaticTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of netty-incubator-codec-http3 Show documentation
Show all versions of netty-incubator-codec-http3 Show documentation
Netty is an asynchronous event-driven network application framework for
rapid development of maintainable high performance protocol servers and
clients.
/*
* Copyright 2020 The Netty Project
*
* The Netty Project licenses this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.netty.incubator.codec.http3;
import io.netty.handler.codec.UnsupportedValueConverter;
import io.netty.util.AsciiString;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
final class QpackStaticTable {
static final int NOT_FOUND = -1;
/**
* Special mask used to disambiguate exact pair index from
* name only index and avoid executing lookup twice. Supposed
* to be used internally. The value should be large enough
* not to override bits from static table index (current size
* of the table is 99 elements).
*/
static final int MASK_NAME_REF = 1 << 10;
/**
* > STATIC_INDEX_BY_NAME = createMap(length);
private static QpackHeaderField newEmptyHeaderField(String name) {
return new QpackHeaderField(AsciiString.cached(name), AsciiString.EMPTY_STRING);
}
private static QpackHeaderField newHeaderField(String name, String value) {
return new QpackHeaderField(AsciiString.cached(name), AsciiString.cached(value));
}
/**
* Return the header field at the given index value.
* Note that QPACK uses 0-based indexing when HPACK is using 1-based.
*/
static QpackHeaderField getField(int index) {
return STATIC_TABLE.get(index);
}
/**
* Returns the lowest index value for the given header field name in the static
* table. Returns -1 if the header field name is not in the static table.
*/
static int getIndex(CharSequence name) {
List index = STATIC_INDEX_BY_NAME.get(name);
if (index == null) {
return NOT_FOUND;
}
return index.get(0);
}
/**
* Returns:
* a) the index value for the given header field in the static table (when found);
* b) the index value for a given name with a single bit masked (no exact match);
* c) -1 if name was not found in the static table.
*/
static int findFieldIndex(CharSequence name, CharSequence value) {
final List nameIndex = STATIC_INDEX_BY_NAME.get(name);
// Early return if name not found in the table.
if (nameIndex == null) {
return NOT_FOUND;
}
// If name was found, check all subsequence elements of the table for exact match.
for (int index: nameIndex) {
QpackHeaderField field = STATIC_TABLE.get(index);
if (QpackUtil.equalsVariableTime(value, field.value)) {
return index;
}
}
// No exact match was found but we still can reference the name.
return nameIndex.get(0) | MASK_NAME_REF;
}
/**
* Creates a map CharSequenceMap header name to index value to allow quick lookup.
*/
@SuppressWarnings("unchecked")
private static CharSequenceMap> createMap(int length) {
CharSequenceMap> mapping =
new CharSequenceMap>(true, UnsupportedValueConverter.>instance(), length);
for (int index = 0; index < length; index++) {
final QpackHeaderField field = getField(index);
final List cursor = mapping.get(field.name);
if (cursor == null) {
final List holder = new ArrayList<>(16);
holder.add(index);
mapping.set(field.name, holder);
} else {
cursor.add(index);
}
}
return mapping;
}
private QpackStaticTable() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy