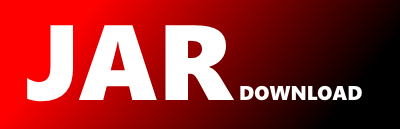
org.jboss.netty.channel.ChannelEvent Maven / Gradle / Ivy
Show all versions of netty Show documentation
/*
* Copyright 2012 The Netty Project
*
* The Netty Project licenses this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package org.jboss.netty.channel;
import org.jboss.netty.buffer.ChannelBuffer;
import org.jboss.netty.channel.socket.ServerSocketChannel;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.net.SocketAddress;
/**
* An I/O event or I/O request associated with a {@link Channel}.
*
* A {@link ChannelEvent} is handled by a series of {@link ChannelHandler}s in
* a {@link ChannelPipeline}.
*
*
Upstream events and downstream events, and their interpretation
*
* Every event is either an upstream event or a downstream event.
* If an event flows forward from the first handler to the last handler in a
* {@link ChannelPipeline}, we call it an upstream event and say "an
* event goes upstream." If an event flows backward from the last
* handler to the first handler in a {@link ChannelPipeline}, we call it a
* downstream event and say "an event goes downstream."
* (Please refer to the diagram in {@link ChannelPipeline} for more explanation.)
*
* When your server receives a message from a client, the event associated with
* the received message is an upstream event. When your server sends a message
* or reply to the client, the event associated with the write request is a
* downstream event. The same rule applies for the client side. If your client
* sent a request to the server, it means your client triggered a downstream
* event. If your client received a response from the server, it means
* your client will be notified with an upstream event. Upstream events are
* often the result of inbound operations such as {@link InputStream#read(byte[])},
* and downstream events are the request for outbound operations such as
* {@link OutputStream#write(byte[])}, {@link Socket#connect(SocketAddress)},
* and {@link Socket#close()}.
*
*
Upstream events
*
*
*
* Event name Event type and condition Meaning
*
*
* {@code "messageReceived"}
* {@link MessageEvent}
* a message object (e.g. {@link ChannelBuffer}) was received from a remote peer
*
*
* {@code "exceptionCaught"}
* {@link ExceptionEvent}
* an exception was raised by an I/O thread or a {@link ChannelHandler}
*
*
* {@code "channelOpen"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#OPEN OPEN}, value = {@code true})
* a {@link Channel} is open, but not bound nor connected
* Be aware that this event is fired from within the I/O thread. You should never
* execute any heavy operation in there as it will block the dispatching to other workers!
*
*
* {@code "channelClosed"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#OPEN OPEN}, value = {@code false})
* a {@link Channel} was closed and all its related resources were released
*
*
* {@code "channelBound"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#BOUND BOUND}, value = {@link SocketAddress})
* a {@link Channel} is open and bound to a local address, but not connected.
* Be aware that this event is fired from within the I/O thread. You should never
* execute any heavy operation in there as it will block the dispatching to other workers!
*
*
* {@code "channelUnbound"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#BOUND BOUND}, value = {@code null})
* a {@link Channel} was unbound from the current local address
*
*
* {@code "channelConnected"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#CONNECTED CONNECTED}, value =
* {@link SocketAddress})
* a {@link Channel} is open, bound to a local address, and connected to a remote address
* Be aware that this event is fired from within the I/O thread. You should never
* execute any heavy operation in there as it will block the dispatching to other workers!
*
*
* {@code "writeComplete"}
* {@link WriteCompletionEvent}
* something has been written to a remote peer
*
*
* {@code "channelDisconnected"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#CONNECTED CONNECTED}, value = {@code null})
* a {@link Channel} was disconnected from its remote peer
*
*
* {@code "channelInterestChanged"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#INTEREST_OPS INTEREST_OPS}, no value)
* a {@link Channel}'s {@link Channel#getInterestOps() interestOps} was changed
*
*
*
* These two additional event types are used only for a parent channel which
* can have a child channel (e.g. {@link ServerSocketChannel}).
*
*
*
* Event name Event type and condition Meaning
*
*
* {@code "childChannelOpen"}
* {@link ChildChannelStateEvent}
({@code childChannel.isOpen() = true})
* a child {@link Channel} was open (e.g. a server channel accepted a connection.)
*
*
* {@code "childChannelClosed"}
* {@link ChildChannelStateEvent}
({@code childChannel.isOpen() = false})
* a child {@link Channel} was closed (e.g. the accepted connection was closed.)
*
*
*
* Downstream events
*
*
*
* Event name Event type and condition Meaning
*
*
* {@code "write"}
* {@link MessageEvent} Send a message to the {@link Channel}.
*
*
* {@code "bind"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#BOUND BOUND}, value = {@link SocketAddress})
* Bind the {@link Channel} to the specified local address.
*
*
* {@code "unbind"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#BOUND BOUND}, value = {@code null})
* Unbind the {@link Channel} from the current local address.
*
*
* {@code "connect"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#CONNECTED CONNECTED}, value =
* {@link SocketAddress})
* Connect the {@link Channel} to the specified remote address.
*
*
* {@code "disconnect"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#CONNECTED CONNECTED}, value = {@code null})
* Disconnect the {@link Channel} from the current remote address.
*
*
* {@code "close"}
* {@link ChannelStateEvent}
(state = {@link ChannelState#OPEN OPEN}, value = {@code false})
* Close the {@link Channel}.
*
*
*
* Other event types and conditions which were not addressed here will be
* ignored and discarded. Please note that there's no {@code "open"} in the
* table. It is because a {@link Channel} is always open when it is created
* by a {@link ChannelFactory}.
*
*
Additional resources worth reading
*
* Please refer to the {@link ChannelHandler} and {@link ChannelPipeline}
* documentation to find out how an event flows in a pipeline and how to handle
* the event in your application.
*
* @apiviz.landmark
* @apiviz.composedOf org.jboss.netty.channel.ChannelFuture
*/
public interface ChannelEvent {
/**
* Returns the {@link Channel} which is associated with this event.
*/
Channel getChannel();
/**
* Returns the {@link ChannelFuture} which is associated with this event.
* If this event is an upstream event, this method will always return a
* {@link SucceededChannelFuture} because the event has occurred already.
* If this event is a downstream event (i.e. I/O request), the returned
* future will be notified when the I/O request succeeds or fails.
*/
ChannelFuture getFuture();
}