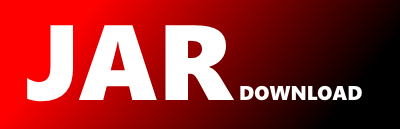
nextflow.config.Manifest.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nextflow Show documentation
Show all versions of nextflow Show documentation
A DSL modelled around the UNIX pipe concept, that simplifies writing parallel and scalable pipelines in a portable manner
/*
* Copyright 2013-2024, Seqera Labs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nextflow.config
import java.util.stream.Collectors
import groovy.transform.CompileStatic
import groovy.transform.EqualsAndHashCode
import groovy.util.logging.Slf4j
import nextflow.exception.AbortOperationException
import static nextflow.Const.DEFAULT_MAIN_FILE_NAME
/**
* Models the nextflow config manifest settings
*
* @author Paolo Di Tommaso
*/
@Slf4j
@CompileStatic
class Manifest {
private Map target
Manifest() { target = Collections.emptyMap() }
Manifest(Map object) {
assert object != null
this.target = new HashMap(object.size())
final validFields = this.metaClass.properties.collect { it.name }.findAll { it!='class' }
object.each { key, value ->
if( validFields.contains(key) )
target.put(key, value)
else
log.warn("Invalid config manifest attribute `$key`")
}
}
String getHomePage() {
target.homePage
}
String getDefaultBranch() {
target.defaultBranch
}
String getDescription() {
target.description
}
String getAuthor() {
target.author
}
List getContributors() {
if( !target.contributors )
return Collections.emptyList()
try {
final contributors = target.contributors as List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy