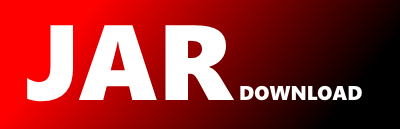
nextflow.util.Trie.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nextflow Show documentation
Show all versions of nextflow Show documentation
A DSL modelled around the UNIX pipe concept, that simplifies writing parallel and scalable pipelines in a portable manner
/*
* Copyright 2013-2024, Seqera Labs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nextflow.util
import groovy.transform.CompileStatic
/**
* Implements a basic Trie data structure
*
* @link http://en.wikipedia.org/wiki/Trie
*
* @author Paolo Di Tommaso
*/
@CompileStatic
class Trie {
private T node
private List> children
T getNode() { node }
List> getChildren() { children }
Trie( T obj ) {
this.node = obj
}
protected Trie addNode( T node ) {
if( children == null ) {
final result = new Trie(node)
children = new LinkedList>()
children.add(result)
return result
}
def result = children?.find { trie -> trie.node == node }
if( !result ) {
result = new Trie(node)
children.add(result)
return result
}
return result
}
Trie addPath( List nodes ) {
if(!nodes)
return null
def node = addNode(nodes.head())
node.addPath(nodes.tail())
return node
}
Trie addPath( T... nodes ) {
addPath( nodes as List )
}
Trie getChild( T name ) {
children?.find { trie -> trie.node == name }
}
List longest() {
longestImpl new LinkedList()
}
private List longestImpl(List result) {
result << node
if( children?.size() == 1 ) {
children.get(0).longestImpl(result)
}
return result
}
List> traverse(T stop=null) {
def result = new ArrayList>()
traverse0(new LinkedList(), result, stop)
return result
}
private void traverse0(List current, List> result, T stop) {
current.add(node)
if( !children || children.any { it.node==stop } ) {
result.add(current)
return
}
for( Trie t : children ) {
t.traverse0(new ArrayList(current), result, stop)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy