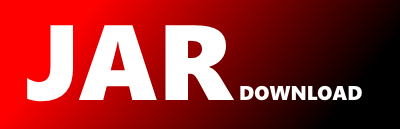
nextflow.util.LockManager.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nextflow Show documentation
Show all versions of nextflow Show documentation
A DSL modelled around the UNIX pipe concept, that simplifies writing parallel and scalable pipelines in a portable manner
/*
* Copyright 2013-2024, Seqera Labs
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nextflow.util
import java.util.concurrent.ConcurrentHashMap
import java.util.concurrent.locks.Lock
import java.util.concurrent.locks.ReentrantLock
import java.util.function.Function
import groovy.transform.CompileStatic
/**
* A lock manager that allows the acquire of a lock on a unique key object
*
* @author Paolo Di Tommaso
*/
@CompileStatic
class LockManager {
private int MAX_SIZE = 200
/**
* Maintain a pool of lock handler to reduce garbage collection
*/
private List pool = new ArrayList<>(MAX_SIZE)
/**
* Associate a lock handle for each key
*/
private ConcurrentHashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy