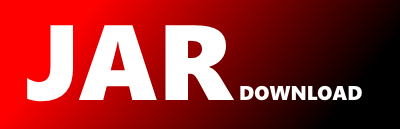
nextflow.io.SkipLinesInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nxf-commons Show documentation
Show all versions of nxf-commons Show documentation
A DSL modelled around the UNIX pipe concept, that simplifies writing parallel and scalable pipelines in a portable manner
/*
* Copyright (c) 2013-2017, Centre for Genomic Regulation (CRG).
* Copyright (c) 2013-2017, Paolo Di Tommaso and the respective authors.
*
* This file is part of 'Nextflow'.
*
* Nextflow is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Nextflow is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Nextflow. If not, see .
*/
package nextflow.io;
import java.io.IOException;
import java.io.InputStream;
/**
* An input stream that skips the first `n` lines in a text stream
*
* @author Paolo Di Tommaso
*/
public class SkipLinesInputStream extends InputStream {
private static final int LF = '\n';
private static final int CR = '\r';
private InputStream target;
private int skip;
private int buffer=-1;
private int count;
/**
* Creates a FilterInputStream
* by assigning the argument in
* to the field this.in
so as
* to remember it for later use.
*
* @param inputStream the underlying input stream, or null
if
* this instance is to be created without an underlying stream.
*/
public SkipLinesInputStream(InputStream inputStream, int skip) {
this.target = inputStream;
this.skip = skip;
}
public long skip(long n) throws IOException {
throw new UnsupportedOperationException("Skip operation is not supported by " + this.getClass().getName());
}
@Override
public int read() throws IOException {
while( count < skip ) {
int ch = read0();
if( ch == -1 )
return -1;
}
return read0();
}
/**
* A line is considered to be terminated by any one
* of a line feed ('\n'), a carriage return ('\r'), or a carriage return
* followed immediately by a linefeed.
*
*/
private int read0() throws IOException {
if( buffer != -1 ) {
int result = buffer;
buffer=-1;
return result;
}
int result = target.read();
if( result == LF ) {
count++;
}
if( result == CR ) {
count++;
int next = target.read();
if( next != LF )
buffer = next;
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy