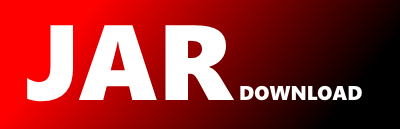
io.norberg.automatter.example.CollectionsFoobarBuilder Maven / Gradle / Ivy
package io.norberg.automatter.example;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
final class CollectionsFoobarBuilder {
private List oxen;
private List cows;
private List foo;
private Map ages;
public CollectionsFoobarBuilder() {
}
private CollectionsFoobarBuilder(CollectionExample.CollectionsFoobar v) {
List extends String> _oxen = v.oxen();
this.oxen = (_oxen == null) ? null : new ArrayList(_oxen);
List extends String> _cows = v.cows();
this.cows = (_cows == null) ? null : new ArrayList(_cows);
List extends Integer> _foo = v.foo();
this.foo = (_foo == null) ? null : new ArrayList(_foo);
Map extends String, ? extends Integer> _ages = v.ages();
this.ages = (_ages == null) ? null : new HashMap(_ages);
}
private CollectionsFoobarBuilder(CollectionsFoobarBuilder v) {
this.oxen = (v.oxen == null) ? null : new ArrayList(v.oxen);
this.cows = (v.cows == null) ? null : new ArrayList(v.cows);
this.foo = (v.foo == null) ? null : new ArrayList(v.foo);
this.ages = (v.ages == null) ? null : new HashMap(v.ages);
}
public List oxen() {
if (this.oxen == null) {
this.oxen = new ArrayList();
}
return oxen;
}
public CollectionsFoobarBuilder oxen(List extends String> oxen) {
return oxen((Collection extends String>) oxen);
}
public CollectionsFoobarBuilder oxen(Collection extends String> oxen) {
if (oxen == null) {
throw new NullPointerException("oxen");
}
for (String item : oxen) {
if (item == null) {
throw new NullPointerException("oxen: null item");
}
}
this.oxen = new ArrayList(oxen);
return this;
}
public CollectionsFoobarBuilder oxen(Iterable extends String> oxen) {
if (oxen == null) {
throw new NullPointerException("oxen");
}
if (oxen instanceof Collection) {
return oxen((Collection extends String>) oxen);
}
return oxen(oxen.iterator());
}
public CollectionsFoobarBuilder oxen(Iterator extends String> oxen) {
if (oxen == null) {
throw new NullPointerException("oxen");
}
this.oxen = new ArrayList();
while (oxen.hasNext()) {
String item = oxen.next();
if (item == null) {
throw new NullPointerException("oxen: null item");
}
this.oxen.add(item);
}
return this;
}
@SafeVarargs
public final CollectionsFoobarBuilder oxen(String... oxen) {
if (oxen == null) {
throw new NullPointerException("oxen");
}
return oxen(Arrays.asList(oxen));
}
public CollectionsFoobarBuilder addOx(String ox) {
if (ox == null) {
throw new NullPointerException("ox");
}
if (this.oxen == null) {
this.oxen = new ArrayList();
}
oxen.add(ox);
return this;
}
public List cows() {
if (this.cows == null) {
this.cows = new ArrayList();
}
return cows;
}
public CollectionsFoobarBuilder cows(List extends String> cows) {
return cows((Collection extends String>) cows);
}
public CollectionsFoobarBuilder cows(Collection extends String> cows) {
if (cows == null) {
throw new NullPointerException("cows");
}
for (String item : cows) {
if (item == null) {
throw new NullPointerException("cows: null item");
}
}
this.cows = new ArrayList(cows);
return this;
}
public CollectionsFoobarBuilder cows(Iterable extends String> cows) {
if (cows == null) {
throw new NullPointerException("cows");
}
if (cows instanceof Collection) {
return cows((Collection extends String>) cows);
}
return cows(cows.iterator());
}
public CollectionsFoobarBuilder cows(Iterator extends String> cows) {
if (cows == null) {
throw new NullPointerException("cows");
}
this.cows = new ArrayList();
while (cows.hasNext()) {
String item = cows.next();
if (item == null) {
throw new NullPointerException("cows: null item");
}
this.cows.add(item);
}
return this;
}
@SafeVarargs
public final CollectionsFoobarBuilder cows(String... cows) {
if (cows == null) {
throw new NullPointerException("cows");
}
return cows(Arrays.asList(cows));
}
public CollectionsFoobarBuilder addCow(String cow) {
if (cow == null) {
throw new NullPointerException("cow");
}
if (this.cows == null) {
this.cows = new ArrayList();
}
cows.add(cow);
return this;
}
public List foo() {
if (this.foo == null) {
this.foo = new ArrayList();
}
return foo;
}
public CollectionsFoobarBuilder foo(List extends Integer> foo) {
return foo((Collection extends Integer>) foo);
}
public CollectionsFoobarBuilder foo(Collection extends Integer> foo) {
if (foo == null) {
throw new NullPointerException("foo");
}
for (Integer item : foo) {
if (item == null) {
throw new NullPointerException("foo: null item");
}
}
this.foo = new ArrayList(foo);
return this;
}
public CollectionsFoobarBuilder foo(Iterable extends Integer> foo) {
if (foo == null) {
throw new NullPointerException("foo");
}
if (foo instanceof Collection) {
return foo((Collection extends Integer>) foo);
}
return foo(foo.iterator());
}
public CollectionsFoobarBuilder foo(Iterator extends Integer> foo) {
if (foo == null) {
throw new NullPointerException("foo");
}
this.foo = new ArrayList();
while (foo.hasNext()) {
Integer item = foo.next();
if (item == null) {
throw new NullPointerException("foo: null item");
}
this.foo.add(item);
}
return this;
}
@SafeVarargs
public final CollectionsFoobarBuilder foo(Integer... foo) {
if (foo == null) {
throw new NullPointerException("foo");
}
return foo(Arrays.asList(foo));
}
public Map ages() {
if (this.ages == null) {
this.ages = new HashMap();
}
return ages;
}
public CollectionsFoobarBuilder ages(Map extends String, ? extends Integer> ages) {
if (ages == null) {
throw new NullPointerException("ages");
}
for (Map.Entry extends String, ? extends Integer> entry : ages.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("ages: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("ages: null value");
}
}
this.ages = new HashMap(ages);
return this;
}
public CollectionsFoobarBuilder ages(String k1, Integer v1) {
if (k1 == null) {
throw new NullPointerException("ages: k1");
}
if (v1 == null) {
throw new NullPointerException("ages: v1");
}
ages = new HashMap();
ages.put(k1, v1);
return this;
}
public CollectionsFoobarBuilder ages(String k1, Integer v1, String k2, Integer v2) {
ages(k1, v1);
if (k2 == null) {
throw new NullPointerException("ages: k2");
}
if (v2 == null) {
throw new NullPointerException("ages: v2");
}
ages.put(k2, v2);
return this;
}
public CollectionsFoobarBuilder ages(String k1, Integer v1, String k2, Integer v2, String k3, Integer v3) {
ages(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("ages: k3");
}
if (v3 == null) {
throw new NullPointerException("ages: v3");
}
ages.put(k3, v3);
return this;
}
public CollectionsFoobarBuilder ages(String k1, Integer v1, String k2, Integer v2, String k3, Integer v3, String k4, Integer v4) {
ages(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("ages: k4");
}
if (v4 == null) {
throw new NullPointerException("ages: v4");
}
ages.put(k4, v4);
return this;
}
public CollectionsFoobarBuilder ages(String k1, Integer v1, String k2, Integer v2, String k3, Integer v3, String k4, Integer v4, String k5, Integer v5) {
ages(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("ages: k5");
}
if (v5 == null) {
throw new NullPointerException("ages: v5");
}
ages.put(k5, v5);
return this;
}
public CollectionsFoobarBuilder putAge(String key, Integer value) {
if (key == null) {
throw new NullPointerException("age: key");
}
if (value == null) {
throw new NullPointerException("age: value");
}
if (this.ages == null) {
this.ages = new HashMap();
}
ages.put(key, value);
return this;
}
public CollectionExample.CollectionsFoobar build() {
List _oxen = (oxen != null) ? Collections.unmodifiableList(new ArrayList(oxen)) : Collections.emptyList();
List _cows = (cows != null) ? Collections.unmodifiableList(new ArrayList(cows)) : Collections.emptyList();
List _foo = (foo != null) ? Collections.unmodifiableList(new ArrayList(foo)) : Collections.emptyList();
Map _ages = (ages != null) ? Collections.unmodifiableMap(new HashMap(ages)) : Collections.emptyMap();
return new Value(_oxen, _cows, _foo, _ages);
}
public static CollectionsFoobarBuilder from(CollectionExample.CollectionsFoobar v) {
return new CollectionsFoobarBuilder(v);
}
public static CollectionsFoobarBuilder from(CollectionsFoobarBuilder v) {
return new CollectionsFoobarBuilder(v);
}
private static final class Value implements CollectionExample.CollectionsFoobar {
private final List oxen;
private final List cows;
private final List foo;
private final Map ages;
private Value(@AutoMatter.Field("oxen") List oxen, @AutoMatter.Field("cows") List cows, @AutoMatter.Field("foo") List foo, @AutoMatter.Field("ages") Map ages) {
this.oxen = (oxen != null) ? oxen : Collections.emptyList();
this.cows = (cows != null) ? cows : Collections.emptyList();
this.foo = (foo != null) ? foo : Collections.emptyList();
this.ages = (ages != null) ? ages : Collections.emptyMap();
}
@AutoMatter.Field
@Override
public List oxen() {
return oxen;
}
@AutoMatter.Field
@Override
public List cows() {
return cows;
}
@AutoMatter.Field
@Override
public List foo() {
return foo;
}
@AutoMatter.Field
@Override
public Map ages() {
return ages;
}
public CollectionsFoobarBuilder builder() {
return new CollectionsFoobarBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof CollectionExample.CollectionsFoobar)) {
return false;
}
final CollectionExample.CollectionsFoobar that = (CollectionExample.CollectionsFoobar) o;
if (oxen != null ? !oxen.equals(that.oxen()) : that.oxen() != null) {
return false;
}
if (cows != null ? !cows.equals(that.cows()) : that.cows() != null) {
return false;
}
if (foo != null ? !foo.equals(that.foo()) : that.foo() != null) {
return false;
}
if (ages != null ? !ages.equals(that.ages()) : that.ages() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (oxen != null ? oxen.hashCode() : 0);
result = 31 * result + (cows != null ? cows.hashCode() : 0);
result = 31 * result + (foo != null ? foo.hashCode() : 0);
result = 31 * result + (ages != null ? ages.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "CollectionExample.CollectionsFoobar{" +
"oxen=" + oxen +
", cows=" + cows +
", foo=" + foo +
", ages=" + ages +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy