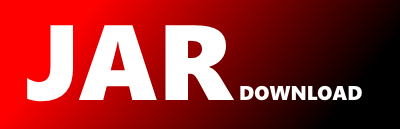
io.norberg.automatter.example.FoobarBuilder Maven / Gradle / Ivy
package io.norberg.automatter.example;
import io.norberg.automatter.AutoMatter;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class FoobarBuilder {
private String foo;
private int bar;
public FoobarBuilder() {
}
private FoobarBuilder(Foobar v) {
this.foo = v.foo();
this.bar = v.bar();
}
private FoobarBuilder(FoobarBuilder v) {
this.foo = v.foo;
this.bar = v.bar;
}
public String foo() {
return foo;
}
public FoobarBuilder foo(String foo) {
if (foo == null) {
throw new NullPointerException("foo");
}
this.foo = foo;
return this;
}
public int bar() {
return bar;
}
public FoobarBuilder bar(int bar) {
this.bar = bar;
return this;
}
public FoobarBuilder builder() {
return new FoobarBuilder(this);
}
public Foobar build() {
return new Value(foo, bar);
}
public static FoobarBuilder from(Foobar v) {
return new FoobarBuilder(v);
}
public static FoobarBuilder from(FoobarBuilder v) {
return new FoobarBuilder(v);
}
private static final class Value implements Foobar {
private final String foo;
private final int bar;
private Value(@AutoMatter.Field("foo") String foo, @AutoMatter.Field("bar") int bar) {
if (foo == null) {
throw new NullPointerException("foo");
}
this.foo = foo;
this.bar = bar;
}
@AutoMatter.Field
@Override
public String foo() {
return foo;
}
@AutoMatter.Field
@Override
public int bar() {
return bar;
}
@Override
public FoobarBuilder builder() {
return new FoobarBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Foobar)) {
return false;
}
final Foobar that = (Foobar) o;
if (foo != null ? !foo.equals(that.foo()) : that.foo() != null) {
return false;
}
if (bar != that.bar()) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (foo != null ? foo.hashCode() : 0);
result = 31 * result + bar;
return result;
}
@Override
public String toString() {
return "Foobar{" +
"foo=" + foo +
", bar=" + bar +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy