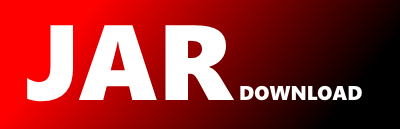
io.norberg.rut.benchmark.RoutingBenchmark Maven / Gradle / Ivy
package io.norberg.rut.benchmark;
import org.openjdk.jmh.annotations.Benchmark;
import org.openjdk.jmh.annotations.Scope;
import org.openjdk.jmh.annotations.Setup;
import org.openjdk.jmh.annotations.State;
import org.openjdk.jmh.runner.Runner;
import org.openjdk.jmh.runner.RunnerException;
import org.openjdk.jmh.runner.options.Options;
import org.openjdk.jmh.runner.options.OptionsBuilder;
import java.util.regex.Pattern;
import io.norberg.rut.Router;
@State(Scope.Thread)
public class RoutingBenchmark {
private static final String[] PATHS = {
"/users/",
"/users/",
"/users//profile",
"/users//blogs/",
"/users//blogs//posts/",
"/users//blogs//posts/",
"/users//blogs/",
"/blogs/",
"/blogs/",
};
private static final String PATH = "/users/foo-user/blogs/bar-blog/posts/baz-post";
private static final Router ROUTER;
private static final Router.Result RESULT;
static {
final Router.Builder builder = Router.builder();
for (final String path : PATHS) {
builder.route("GET", path, path);
}
ROUTER = builder.build();
RESULT = ROUTER.result();
}
private static final Pattern[] PATTERNS;
static {
PATTERNS = new Pattern[PATHS.length];
for (int i = 0; i < PATHS.length; i++) {
final String path = PATHS[i];
final String regex = path.replaceAll("<.+?>", "[^/]*");
PATTERNS[i] = Pattern.compile(regex);
}
}
private String path;
private Pattern[] uriPatterns;
@Setup
public void setup() {
path = PATH;
uriPatterns = PATTERNS;
}
@Benchmark
public Pattern regexRouting() throws InterruptedException {
for (final Pattern pattern : uriPatterns) {
if (pattern.matcher(path).matches()) {
return pattern;
}
}
throw new AssertionError();
}
@Benchmark
public String radixTreeRouting() {
ROUTER.route("GET", path, RESULT);
final String target = RESULT.target();
if (target == null) {
throw new AssertionError();
}
return target;
}
public static void main(final String... args) throws RunnerException {
Options opt = new OptionsBuilder()
.include(".*" + RoutingBenchmark.class.getSimpleName() + ".*")
.warmupIterations(5)
.measurementIterations(20)
.forks(5)
.build();
new Runner(opt).run();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy