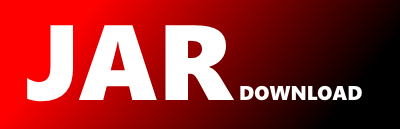
io.nosqlbench.adapter.diag.DiagOpDispenser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adapter-diag Show documentation
Show all versions of adapter-diag Show documentation
A nosqlbench ActivityType (AT) driver module;
Provides a diagnostic activity that logs input at some interval
/*
* Copyright (c) 2022 nosqlbench
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.nosqlbench.adapter.diag;
import io.nosqlbench.adapter.diag.optasks.DiagTask;
import io.nosqlbench.engine.api.activityapi.ratelimits.RateLimiter;
import io.nosqlbench.engine.api.activityimpl.BaseOpDispenser;
import io.nosqlbench.engine.api.activityimpl.uniform.DriverAdapter;
import io.nosqlbench.engine.api.templating.ParsedOp;
import io.nosqlbench.nb.annotations.ServiceSelector;
import io.nosqlbench.api.config.standard.NBConfigModel;
import io.nosqlbench.api.config.standard.NBConfiguration;
import io.nosqlbench.api.config.standard.NBReconfigurable;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.*;
import java.util.function.LongFunction;
public class DiagOpDispenser extends BaseOpDispenser implements NBReconfigurable {
private final static Logger logger = LogManager.getLogger(DiagOpDispenser.class);
private OpFunc opFunc;
private RateLimiter diagRateLimiter;
private LongFunction spaceF;
private OpFunc opFuncs;
public DiagOpDispenser(DriverAdapter adapter, ParsedOp op) {
super(adapter,op);
this.opFunc = resolveOpFunc(op);
}
private OpFunc resolveOpFunc(ParsedOp op) {
List tasks = new ArrayList<>();
Set tasknames = op.getDefinedNames();
/**
* Dynamically load diag tasks and add them to the in-memory template used by the op dispenser
*/
for (String taskname : tasknames) {
// Get the value of the keyed task name, but throw an error if it is not static or not a map
// Get the op config by name, if provided in string or map form, and
// produce a normalized map form which contains the type field. If
// the type isn't contained in the parsed form, inject the name as short-hand
// Also, inject the name into the map
Map taskcfg = op.parseStaticCmdMap(taskname, "type");
taskcfg.computeIfAbsent("name",l -> taskname);
taskcfg.computeIfAbsent("type",l -> taskname);
String optype = taskcfg.remove("type").toString();
// Dynamically load the named task instance, based on the op field key AKA the taskname
// and ensure that exactly one is found or throw an error
DiagTask task = ServiceSelector.of(optype, ServiceLoader.load(DiagTask.class)).getOne();
// Load the configuration model of the dynamically loaded task for type-safe configuration
NBConfigModel cfgmodel = task.getConfigModel();
// Apply the raw configuration data to the configuration model, which
// both validates the provided configuration fields and
// yields a usable configuration that should apply to the loaded task without error or ambiguity
NBConfiguration taskconfig = cfgmodel.apply(taskcfg);
// Apply the validated configuration to the loaded task
task.applyConfig(taskconfig);
// Store the task into the diag op's list of things to do when it runs
tasks.add(task);
}
this.opFunc = new OpFunc(tasks);
return opFunc;
}
@Override
public void applyReconfig(NBConfiguration recfg) {
opFunc.applyReconfig(recfg);
}
@Override
public NBConfigModel getReconfigModel() {
return opFunc.getReconfigModel();
}
private final static class OpFunc implements LongFunction, NBReconfigurable {
private final List tasks;
public OpFunc(List tasks) {
this.tasks = tasks;
}
@Override
public DiagOp apply(long value) {
return new DiagOp(tasks);
}
@Override
public void applyReconfig(NBConfiguration recfg) {
NBReconfigurable.applyMatching(recfg,tasks);
}
@Override
public NBConfigModel getReconfigModel() {
return NBReconfigurable.collectModels(DiagTask.class, tasks);
}
}
@Override
public DiagOp apply(long value) {
return opFunc.apply(value);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy