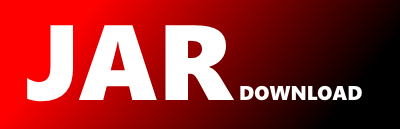
com.carrotsearch.hppc.FloatContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
package com.carrotsearch.hppc;
import java.util.Iterator;
import com.carrotsearch.hppc.cursors.FloatCursor;
import com.carrotsearch.hppc.predicates.FloatPredicate;
import com.carrotsearch.hppc.procedures.FloatProcedure;
/**
* A generic container holding float
s.
*/
@javax.annotation.Generated(
date = "2015-05-07T09:33:04+0200",
value = "KTypeContainer.java")
public interface FloatContainer extends Iterable {
/**
* Returns an iterator to a cursor traversing the collection. The order of
* traversal is not defined. More than one cursor may be active at a time. The
* behavior of iterators is undefined if structural changes are made to the
* underlying collection.
*
*
* The iterator is implemented as a cursor and it returns the same cursor
* instance on every call to {@link Iterator#next()} (to avoid boxing of
* primitive types). To read the current list's value (or index in the list)
* use the cursor's public fields. An example is shown below.
*
*
*
* for (FloatCursor<float> c : container) {
* System.out.println("index=" + c.index + " value=" + c.value);
* }
*
*/
public Iterator iterator();
/**
* Lookup a given element in the container. This operation has no speed
* guarantees (may be linear with respect to the size of this container).
*
* @return Returns true
if this container has an element equal to
* e
.
*/
public boolean contains(float e);
/**
* Return the current number of elements in this container. The time for
* calculating the container's size may take O(n)
time, although
* implementing classes should try to maintain the current size and return in
* constant time.
*/
public int size();
/**
* Shortcut for size() == 0
.
*/
public boolean isEmpty();
/**
* Copies all elements of this container to an array.
*
* The returned array is always a copy, regardless of the storage used by the
* container.
*/
public float [] toArray();
/* */
/**
* Applies a procedure
to all container elements. Returns the
* argument (any subclass of {@link FloatProcedure}. This lets the caller to
* call methods of the argument by chaining the call (even if the argument is
* an anonymous type) to retrieve computed values, for example (IntContainer):
*
*
* int count = container.forEach(new IntProcedure() {
* int count; // this is a field declaration in an anonymous class.
*
* public void apply(int value) {
* count++;
* }
* }).count;
*
*/
public T forEach(T procedure);
/**
* Applies a predicate
to container elements as long, as the
* predicate returns true
. The iteration is interrupted
* otherwise.
*/
public T forEach(T predicate);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy