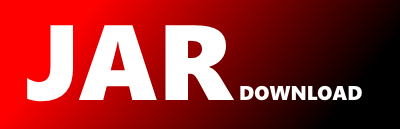
com.datastax.driver.core.exceptions.ReadFailureException Maven / Gradle / Ivy
Show all versions of driver-cql-shaded Show documentation
/*
* Copyright DataStax, Inc.
*
* This software can be used solely with DataStax Enterprise. Please consult the license at
* http://www.datastax.com/terms/datastax-dse-driver-license-terms
*/
package com.datastax.driver.core.exceptions;
import com.datastax.driver.core.ConsistencyLevel;
import com.datastax.driver.core.EndPoint;
import java.net.InetAddress;
import java.util.Collections;
import java.util.Map;
/**
* A non-timeout error during a read query.
*
* This happens when some of the replicas that were contacted by the coordinator replied with an
* error.
*/
@SuppressWarnings("serial")
public class ReadFailureException extends QueryConsistencyException {
private final int failed;
private final boolean dataPresent;
private final Map failuresMap;
/**
* This constructor should only be used internally by the driver when decoding error responses.
*/
public ReadFailureException(
ConsistencyLevel consistency,
int received,
int required,
int failed,
Map failuresMap,
boolean dataPresent) {
this(null, consistency, received, required, failed, failuresMap, dataPresent);
}
/** @deprecated Legacy constructor for backward compatibility. */
@Deprecated
public ReadFailureException(
ConsistencyLevel consistency, int received, int required, int failed, boolean dataPresent) {
this(
null,
consistency,
received,
required,
failed,
Collections.emptyMap(),
dataPresent);
}
public ReadFailureException(
EndPoint endPoint,
ConsistencyLevel consistency,
int received,
int required,
int failed,
Map failuresMap,
boolean dataPresent) {
super(
endPoint,
String.format(
"Cassandra failure during read query at consistency %s "
+ "(%d responses were required but only %d replica responded, %d failed)",
consistency, required, received, failed),
consistency,
received,
required);
this.failed = failed;
this.failuresMap = failuresMap;
this.dataPresent = dataPresent;
}
/** @deprecated Legacy constructor for backward compatibility. */
@Deprecated
public ReadFailureException(
EndPoint endPoint,
ConsistencyLevel consistency,
int received,
int required,
int failed,
boolean dataPresent) {
this(
endPoint,
consistency,
received,
required,
failed,
Collections.emptyMap(),
dataPresent);
}
private ReadFailureException(
EndPoint endPoint,
String msg,
Throwable cause,
ConsistencyLevel consistency,
int received,
int required,
int failed,
Map failuresMap,
boolean dataPresent) {
super(endPoint, msg, cause, consistency, received, required);
this.failed = failed;
this.failuresMap = failuresMap;
this.dataPresent = dataPresent;
}
/**
* Returns the number of replicas that experienced a failure while executing the request.
*
* @return the number of failures.
*/
public int getFailures() {
return failed;
}
/**
* Returns the a failure reason code for each node that failed.
*
* At the time of writing, the existing reason codes are:
*
*
* - {@code 0x0000}: the error does not have a specific code assigned yet, or the cause is
* unknown.
*
- {@code 0x0001}: The read operation scanned too many tombstones (as defined by {@code
* tombstone_failure_threshold} in {@code cassandra.yaml}, causing a {@code
* TombstoneOverwhelmingException}.
*
*
* (please refer to the Cassandra documentation for your version for the most up-to-date list of
* errors)
*
* This feature is available for protocol v5 or above only. With lower protocol versions, the
* map will always be empty.
*
* @return a map of IP addresses to failure codes.
*/
public Map getFailuresMap() {
return failuresMap;
}
/**
* Whether the actual data was amongst the received replica responses.
*
* During reads, Cassandra doesn't request data from every replica to minimize internal network
* traffic. Instead, some replicas are only asked for a checksum of the data. A read timeout may
* occurred even if enough replicas have responded to fulfill the consistency level if only
* checksum responses have been received. This method allows to detect that case.
*
* @return whether the data was amongst the received replica responses.
*/
public boolean wasDataRetrieved() {
return dataPresent;
}
@Override
public ReadFailureException copy() {
return new ReadFailureException(
getEndPoint(),
getMessage(),
this,
getConsistencyLevel(),
getReceivedAcknowledgements(),
getRequiredAcknowledgements(),
getFailures(),
getFailuresMap(),
wasDataRetrieved());
}
public ReadFailureException copy(EndPoint endPoint) {
return new ReadFailureException(
endPoint,
getMessage(),
this,
getConsistencyLevel(),
getReceivedAcknowledgements(),
getRequiredAcknowledgements(),
failed,
getFailuresMap(),
dataPresent);
}
}