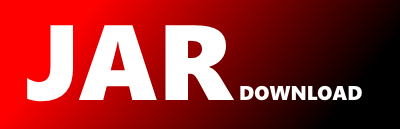
com.datastax.driver.core.exceptions.WriteTimeoutException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
/*
* Copyright DataStax, Inc.
*
* This software can be used solely with DataStax Enterprise. Please consult the license at
* http://www.datastax.com/terms/datastax-dse-driver-license-terms
*/
package com.datastax.driver.core.exceptions;
import com.datastax.driver.core.ConsistencyLevel;
import com.datastax.driver.core.EndPoint;
import com.datastax.driver.core.WriteType;
/** A Cassandra timeout during a write query. */
public class WriteTimeoutException extends QueryConsistencyException {
private static final long serialVersionUID = 0;
private final WriteType writeType;
/**
* This constructor should only be used internally by the driver when decoding error responses.
*/
public WriteTimeoutException(
ConsistencyLevel consistency, WriteType writeType, int received, int required) {
this(null, consistency, writeType, received, required);
}
public WriteTimeoutException(
EndPoint endPoint,
ConsistencyLevel consistency,
WriteType writeType,
int received,
int required) {
super(
endPoint,
String.format(
"Cassandra timeout during %s write query at consistency %s "
+ "(%d replica were required but only %d acknowledged the write)",
writeType, consistency, required, received),
consistency,
received,
required);
this.writeType = writeType;
}
private WriteTimeoutException(
EndPoint endPoint,
String msg,
Throwable cause,
ConsistencyLevel consistency,
WriteType writeType,
int received,
int required) {
super(endPoint, msg, cause, consistency, received, required);
this.writeType = writeType;
}
/**
* The type of the write for which a timeout was raised.
*
* @return the type of the write for which a timeout was raised.
*/
public WriteType getWriteType() {
return writeType;
}
@Override
public WriteTimeoutException copy() {
return new WriteTimeoutException(
getEndPoint(),
getMessage(),
this,
getConsistencyLevel(),
getWriteType(),
getReceivedAcknowledgements(),
getRequiredAcknowledgements());
}
/**
* Create a copy of this exception with a nicer stack trace, and including the coordinator address
* that caused this exception to be raised.
*
* This method is mainly intended for internal use by the driver and exists mainly because:
*
*
* - the original exception was decoded from a response frame and at that time, the
* coordinator address was not available; and
*
- the newly-created exception will refer to the current thread in its stack trace, which
* generally yields a more user-friendly stack trace that the original one.
*
*
* @param address The full address of the host that caused this exception to be thrown.
* @return a copy/clone of this exception, but with the given host address instead of the original
* one.
*/
public WriteTimeoutException copy(EndPoint address) {
return new WriteTimeoutException(
address,
getMessage(),
this,
getConsistencyLevel(),
getWriteType(),
getReceivedAcknowledgements(),
getRequiredAcknowledgements());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy