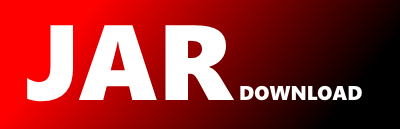
com.datastax.driver.core.schemabuilder.SchemaStatement Maven / Gradle / Ivy
Show all versions of driver-cql-shaded Show documentation
/*
* Copyright DataStax, Inc.
*
* This software can be used solely with DataStax Enterprise. Please consult the license at
* http://www.datastax.com/terms/datastax-dse-driver-license-terms
*/
package com.datastax.driver.core.schemabuilder;
import com.datastax.driver.core.CodecRegistry;
import com.datastax.driver.core.Metadata;
import com.datastax.driver.core.ProtocolVersion;
import com.datastax.driver.core.RegularStatement;
import com.datastax.driver.core.Statement;
import com.google.common.base.Strings;
import java.nio.ByteBuffer;
import java.util.Map;
/** A DDL statement generated by {@link SchemaBuilder}. */
public abstract class SchemaStatement extends RegularStatement {
static final String STATEMENT_START = "\n\t";
static final String COLUMN_FORMATTING = "\n\t\t";
private volatile String cache;
private String keyspace;
abstract String buildInternal();
@Override
public String getQueryString(CodecRegistry codecRegistry) {
if (cache == null) {
cache = buildInternal();
}
return cache;
}
@Override
public ByteBuffer[] getValues(ProtocolVersion protocolVersion, CodecRegistry codecRegistry) {
// DDL statements never have values
return new ByteBuffer[0];
}
@Override
public boolean hasValues(CodecRegistry codecRegistry) {
return false;
}
@Override
public Map getNamedValues(
ProtocolVersion protocolVersion, CodecRegistry codecRegistry) {
// DDL statements never have values
return null;
}
@Override
public boolean usesNamedValues() {
return false;
}
@Override
public String getKeyspace() {
return keyspace;
}
/**
* Sets the keyspace this query operates on.
*
* This method allows you to manually provide a keyspace for this query. It is used for the
* following:
*
*
* - To indicate to cassandra what keyspace the statement is applicable to (protocol V5+
* only). This is useful when the query does not provide an explicit keyspace and you want
* to override the session's keyspace.
*
- By {@link com.datastax.driver.core.policies.TokenAwarePolicy} to help identify which
* replicas are applicable to send this statement to.
*
*
* Do note that if the query does not use a fully qualified keyspace, then you do not need to set
* the keyspace through this method as the currently logged in keyspace will be used if it is set.
*
* Note: This expects the internal representation of the keyspace. In the typical case,
* the internal representation is equivalent to the CQL representation. However, if you are using
* a keyspace that requires the use of quotes in CQL (a quoted identifier), i.e.: "MyKS", this
* method will return the unquoted representation instead, i.e. MyKS.
*
* @param keyspace the name of the keyspace this query operates on.
* @return this {@code SchemaStatement} object.
* @see Statement#getKeyspace
*/
public SchemaStatement setKeyspace(String keyspace) {
this.keyspace = keyspace;
return this;
}
/**
* {@inheritDoc}
*
* @param protocolVersion unused by this implementation (the key is always null for schema
* statements).
* @param codecRegistry unused by this implementation (the key is always null for schema
* statements).
*/
@Override
public ByteBuffer getRoutingKey(ProtocolVersion protocolVersion, CodecRegistry codecRegistry) {
return null; // there is no token awareness for DDL statements
}
static void validateNotEmpty(String columnName, String label) {
if (Strings.isNullOrEmpty(columnName)) {
throw new IllegalArgumentException(label + " should not be null or blank");
}
}
static void validateNotNull(Object value, String label) {
if (value == null) {
throw new IllegalArgumentException(label + " should not be null");
}
}
static void validateNotKeyWord(String label, String message) {
if (Metadata.isReservedCqlKeyword(label)) {
throw new IllegalArgumentException(message);
}
}
static SchemaStatement fromQueryString(final String queryString) {
return new SchemaStatement() {
@Override
public String buildInternal() {
return queryString;
}
};
}
StatementStart asStatementStart() {
return new StatementStart() {
@Override
public String buildInternal() {
return SchemaStatement.this.buildInternal();
}
};
}
}