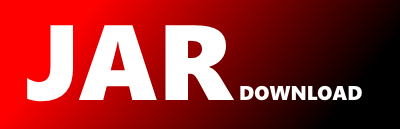
com.oracle.truffle.polyglot.PolyglotProxyGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
// CheckStyle: start generated
package com.oracle.truffle.polyglot;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.TruffleLanguage;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.TruffleLanguage.ContextReference;
import com.oracle.truffle.api.TruffleLanguage.LanguageReference;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.ArityException;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.InvalidArrayIndexException;
import com.oracle.truffle.api.interop.UnknownIdentifierException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.interop.UnsupportedTypeException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.utilities.TriState;
import com.oracle.truffle.polyglot.HostLanguage.HostContext;
import com.oracle.truffle.polyglot.PolyglotProxy.IsIdenticalOrUndefined;
import java.time.Duration;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.ZoneId;
import java.util.concurrent.locks.Lock;
@GeneratedBy(PolyglotProxy.class)
@SuppressWarnings("unused")
final class PolyglotProxyGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
static {
LibraryExport.register(PolyglotProxy.class, new InteropLibraryExports());
}
private PolyglotProxyGen() {
}
@GeneratedBy(PolyglotProxy.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, PolyglotProxy.class, false);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof PolyglotProxy;
InteropLibrary uncached = new Uncached();
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof PolyglotProxy;
return new Cached();
}
@GeneratedBy(PolyglotProxy.class)
private static final class Cached extends InteropLibrary {
@CompilationFinal private volatile int state_;
@Child private InteropLibrary executables;
@CompilationFinal private ContextReference hostLanguageContextReference_;
@CompilationFinal private LanguageReference hostLanguageReference_;
Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof PolyglotProxy) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof PolyglotProxy;
}
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b11) != 0 /* is-active doHostObject(PolyglotProxy, PolyglotProxy) || doOther(PolyglotProxy, Object) */) {
if ((state & 0b1) != 0 /* is-active doHostObject(PolyglotProxy, PolyglotProxy) */ && arg1Value instanceof PolyglotProxy) {
PolyglotProxy arg1Value_ = (PolyglotProxy) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
if ((state & 0b10) != 0 /* is-active doOther(PolyglotProxy, Object) */) {
if (isIdenticalOrUndefinedFallbackGuard_(state, arg0Value, arg1Value)) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isIdenticalOrUndefinedAndSpecialize(arg0Value, arg1Value);
}
private TriState isIdenticalOrUndefinedAndSpecialize(PolyglotProxy arg0Value, Object arg1Value) {
int state = state_;
if (arg1Value instanceof PolyglotProxy) {
PolyglotProxy arg1Value_ = (PolyglotProxy) arg1Value;
this.state_ = state = state | 0b1 /* add-active doHostObject(PolyglotProxy, PolyglotProxy) */;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
this.state_ = state = state | 0b10 /* add-active doOther(PolyglotProxy, Object) */;
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state = state_;
if ((state & 0b11) == 0b0) {
return NodeCost.UNINITIALIZED;
} else if (((state & 0b11) & ((state & 0b11) - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
@Override
public boolean isInstantiable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isInstantiable();
}
@Override
public Object instantiate(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b100) != 0 /* is-active instantiate(PolyglotProxy, Object[], InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary instantiateNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference instantiateNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage instantiateNode__language__ = hostLanguageReference__.get();
return arg0Value.instantiate(arg1Value, instantiateNode__library__, instantiateNode__context__, instantiateNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return instantiateNode_AndSpecialize(arg0Value, arg1Value);
}
private Object instantiateNode_AndSpecialize(PolyglotProxy arg0Value, Object[] arg1Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage instantiateNode__language__ = null;
ContextReference instantiateNode__context__ = null;
InteropLibrary instantiateNode__library__ = null;
instantiateNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
instantiateNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
instantiateNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b100 /* add-active instantiate(PolyglotProxy, Object[], InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.instantiate(arg1Value, instantiateNode__library__, instantiateNode__context__, instantiateNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isExecutable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isExecutable();
}
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000) != 0 /* is-active execute(PolyglotProxy, Object[], InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary executeNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference executeNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage executeNode__language__ = hostLanguageReference__.get();
return arg0Value.execute(arg1Value, executeNode__library__, executeNode__context__, executeNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeNode_AndSpecialize(arg0Value, arg1Value);
}
private Object executeNode_AndSpecialize(PolyglotProxy arg0Value, Object[] arg1Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage executeNode__language__ = null;
ContextReference executeNode__context__ = null;
InteropLibrary executeNode__library__ = null;
executeNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
executeNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
executeNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b1000 /* add-active execute(PolyglotProxy, Object[], InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.execute(arg1Value, executeNode__library__, executeNode__context__, executeNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isPointer(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isPointer();
}
@Override
public long asPointer(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b10000) != 0 /* is-active asPointer(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asPointerNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asPointerNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asPointerNode__language__ = hostLanguageReference__.get();
return arg0Value.asPointer(asPointerNode__library__, asPointerNode__context__, asPointerNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asPointerNode_AndSpecialize(arg0Value);
}
private long asPointerNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asPointerNode__language__ = null;
ContextReference asPointerNode__context__ = null;
InteropLibrary asPointerNode__library__ = null;
asPointerNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asPointerNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asPointerNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b10000 /* add-active asPointer(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asPointer(asPointerNode__library__, asPointerNode__context__, asPointerNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean hasArrayElements(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).hasArrayElements();
}
@Override
public Object readArrayElement(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidArrayIndexException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b100000) != 0 /* is-active readArrayElement(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary readArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference readArrayElementNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage readArrayElementNode__language__ = hostLanguageReference__.get();
return arg0Value.readArrayElement(arg1Value, readArrayElementNode__library__, readArrayElementNode__context__, readArrayElementNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readArrayElementNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readArrayElementNode_AndSpecialize(PolyglotProxy arg0Value, long arg1Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage readArrayElementNode__language__ = null;
ContextReference readArrayElementNode__context__ = null;
InteropLibrary readArrayElementNode__library__ = null;
readArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
readArrayElementNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
readArrayElementNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b100000 /* add-active readArrayElement(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.readArrayElement(arg1Value, readArrayElementNode__library__, readArrayElementNode__context__, readArrayElementNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public void writeArrayElement(Object arg0Value_, long arg1Value, Object arg2Value) throws UnsupportedMessageException, UnsupportedTypeException, InvalidArrayIndexException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000) != 0 /* is-active writeArrayElement(PolyglotProxy, long, Object, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary writeArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference writeArrayElementNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage writeArrayElementNode__language__ = hostLanguageReference__.get();
arg0Value.writeArrayElement(arg1Value, arg2Value, writeArrayElementNode__library__, writeArrayElementNode__context__, writeArrayElementNode__language__);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
writeArrayElementNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void writeArrayElementNode_AndSpecialize(PolyglotProxy arg0Value, long arg1Value, Object arg2Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage writeArrayElementNode__language__ = null;
ContextReference writeArrayElementNode__context__ = null;
InteropLibrary writeArrayElementNode__library__ = null;
writeArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
writeArrayElementNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
writeArrayElementNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b1000000 /* add-active writeArrayElement(PolyglotProxy, long, Object, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
arg0Value.writeArrayElement(arg1Value, arg2Value, writeArrayElementNode__library__, writeArrayElementNode__context__, writeArrayElementNode__language__);
return;
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public void removeArrayElement(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidArrayIndexException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b10000000) != 0 /* is-active removeArrayElement(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary removeArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference removeArrayElementNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage removeArrayElementNode__language__ = hostLanguageReference__.get();
arg0Value.removeArrayElement(arg1Value, removeArrayElementNode__library__, removeArrayElementNode__context__, removeArrayElementNode__language__);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
removeArrayElementNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void removeArrayElementNode_AndSpecialize(PolyglotProxy arg0Value, long arg1Value) throws UnsupportedMessageException, InvalidArrayIndexException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage removeArrayElementNode__language__ = null;
ContextReference removeArrayElementNode__context__ = null;
InteropLibrary removeArrayElementNode__library__ = null;
removeArrayElementNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
removeArrayElementNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
removeArrayElementNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b10000000 /* add-active removeArrayElement(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
arg0Value.removeArrayElement(arg1Value, removeArrayElementNode__library__, removeArrayElementNode__context__, removeArrayElementNode__language__);
return;
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public long getArraySize(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b100000000) != 0 /* is-active getArraySize(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary getArraySizeNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference getArraySizeNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage getArraySizeNode__language__ = hostLanguageReference__.get();
return arg0Value.getArraySize(getArraySizeNode__library__, getArraySizeNode__context__, getArraySizeNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getArraySizeNode_AndSpecialize(arg0Value);
}
private long getArraySizeNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage getArraySizeNode__language__ = null;
ContextReference getArraySizeNode__context__ = null;
InteropLibrary getArraySizeNode__library__ = null;
getArraySizeNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
getArraySizeNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
getArraySizeNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b100000000 /* add-active getArraySize(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.getArraySize(getArraySizeNode__library__, getArraySizeNode__context__, getArraySizeNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isArrayElementReadable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000000) != 0 /* is-active isArrayElementExisting(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isArrayElementExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isArrayElementExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isArrayElementExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isArrayElementExisting(arg1Value, isArrayElementExistingNode__library__, isArrayElementExistingNode__context__, isArrayElementExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isArrayElementExistingNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isArrayElementExistingNode_AndSpecialize(PolyglotProxy arg0Value, long arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage isArrayElementExistingNode__language__ = null;
ContextReference isArrayElementExistingNode__context__ = null;
InteropLibrary isArrayElementExistingNode__library__ = null;
isArrayElementExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
isArrayElementExistingNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
isArrayElementExistingNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b1000000000 /* add-active isArrayElementExisting(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.isArrayElementExisting(arg1Value, isArrayElementExistingNode__library__, isArrayElementExistingNode__context__, isArrayElementExistingNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isArrayElementModifiable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000000) != 0 /* is-active isArrayElementExisting(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isArrayElementExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isArrayElementExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isArrayElementExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isArrayElementExisting(arg1Value, isArrayElementExistingNode__library__, isArrayElementExistingNode__context__, isArrayElementExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isArrayElementExistingNode_AndSpecialize(arg0Value, arg1Value);
}
@Override
public boolean isArrayElementRemovable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000000) != 0 /* is-active isArrayElementExisting(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isArrayElementExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isArrayElementExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isArrayElementExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isArrayElementExisting(arg1Value, isArrayElementExistingNode__library__, isArrayElementExistingNode__context__, isArrayElementExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isArrayElementExistingNode_AndSpecialize(arg0Value, arg1Value);
}
@Override
public boolean isArrayElementInsertable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b10000000000) != 0 /* is-active isArrayElementInsertable(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isArrayElementInsertableNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isArrayElementInsertableNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isArrayElementInsertableNode__language__ = hostLanguageReference__.get();
return arg0Value.isArrayElementInsertable(arg1Value, isArrayElementInsertableNode__library__, isArrayElementInsertableNode__context__, isArrayElementInsertableNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isArrayElementInsertableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isArrayElementInsertableNode_AndSpecialize(PolyglotProxy arg0Value, long arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage isArrayElementInsertableNode__language__ = null;
ContextReference isArrayElementInsertableNode__context__ = null;
InteropLibrary isArrayElementInsertableNode__library__ = null;
isArrayElementInsertableNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
isArrayElementInsertableNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
isArrayElementInsertableNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b10000000000 /* add-active isArrayElementInsertable(PolyglotProxy, long, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.isArrayElementInsertable(arg1Value, isArrayElementInsertableNode__library__, isArrayElementInsertableNode__context__, isArrayElementInsertableNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean hasMembers(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).hasMembers();
}
@Override
public Object getMembers(Object arg0Value_, boolean arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b100000000000) != 0 /* is-active getMembers(PolyglotProxy, boolean, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary getMembersNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference getMembersNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage getMembersNode__language__ = hostLanguageReference__.get();
return arg0Value.getMembers(arg1Value, getMembersNode__library__, getMembersNode__context__, getMembersNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getMembersNode_AndSpecialize(arg0Value, arg1Value);
}
private Object getMembersNode_AndSpecialize(PolyglotProxy arg0Value, boolean arg1Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage getMembersNode__language__ = null;
ContextReference getMembersNode__context__ = null;
InteropLibrary getMembersNode__library__ = null;
getMembersNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
getMembersNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
getMembersNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b100000000000 /* add-active getMembers(PolyglotProxy, boolean, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.getMembers(arg1Value, getMembersNode__library__, getMembersNode__context__, getMembersNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public Object readMember(Object arg0Value_, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000000000) != 0 /* is-active readMember(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary readMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference readMemberNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage readMemberNode__language__ = hostLanguageReference__.get();
return arg0Value.readMember(arg1Value, readMemberNode__library__, readMemberNode__context__, readMemberNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readMemberNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readMemberNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage readMemberNode__language__ = null;
ContextReference readMemberNode__context__ = null;
InteropLibrary readMemberNode__library__ = null;
readMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
readMemberNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
readMemberNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b1000000000000 /* add-active readMember(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.readMember(arg1Value, readMemberNode__library__, readMemberNode__context__, readMemberNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public void writeMember(Object arg0Value_, String arg1Value, Object arg2Value) throws UnsupportedMessageException, UnknownIdentifierException, UnsupportedTypeException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b10000000000000) != 0 /* is-active writeMember(PolyglotProxy, String, Object, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary writeMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference writeMemberNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage writeMemberNode__language__ = hostLanguageReference__.get();
arg0Value.writeMember(arg1Value, arg2Value, writeMemberNode__library__, writeMemberNode__context__, writeMemberNode__language__);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
writeMemberNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void writeMemberNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value, Object arg2Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage writeMemberNode__language__ = null;
ContextReference writeMemberNode__context__ = null;
InteropLibrary writeMemberNode__library__ = null;
writeMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
writeMemberNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
writeMemberNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b10000000000000 /* add-active writeMember(PolyglotProxy, String, Object, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
arg0Value.writeMember(arg1Value, arg2Value, writeMemberNode__library__, writeMemberNode__context__, writeMemberNode__language__);
return;
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public Object invokeMember(Object arg0Value_, String arg1Value, Object... arg2Value) throws UnsupportedMessageException, ArityException, UnknownIdentifierException, UnsupportedTypeException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b100000000000000) != 0 /* is-active invokeMember(PolyglotProxy, String, Object[], InteropLibrary, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary invokeMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference invokeMemberNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage invokeMemberNode__language__ = hostLanguageReference__.get();
return arg0Value.invokeMember(arg1Value, arg2Value, invokeMemberNode__library__, this.executables, invokeMemberNode__context__, invokeMemberNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return invokeMemberNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object invokeMemberNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value, Object[] arg2Value) throws UnsupportedMessageException, UnsupportedTypeException, ArityException, UnknownIdentifierException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage invokeMemberNode__language__ = null;
ContextReference invokeMemberNode__context__ = null;
InteropLibrary invokeMemberNode__library__ = null;
invokeMemberNode__library__ = (this);
if (this.executables == null) {
this.executables = super.insert((INTEROP_LIBRARY_.createDispatched(PolyglotProxy.LIMIT)));
}
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
invokeMemberNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
invokeMemberNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b100000000000000 /* add-active invokeMember(PolyglotProxy, String, Object[], InteropLibrary, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.invokeMember(arg1Value, arg2Value, invokeMemberNode__library__, this.executables, invokeMemberNode__context__, invokeMemberNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isMemberInvocable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0b1000000000000000) != 0 /* is-active isMemberInvocable(PolyglotProxy, String, InteropLibrary, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isMemberInvocableNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isMemberInvocableNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isMemberInvocableNode__language__ = hostLanguageReference__.get();
return arg0Value.isMemberInvocable(arg1Value, isMemberInvocableNode__library__, this.executables, isMemberInvocableNode__context__, isMemberInvocableNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isMemberInvocableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isMemberInvocableNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage isMemberInvocableNode__language__ = null;
ContextReference isMemberInvocableNode__context__ = null;
InteropLibrary isMemberInvocableNode__library__ = null;
isMemberInvocableNode__library__ = (this);
if (this.executables == null) {
this.executables = super.insert((INTEROP_LIBRARY_.createDispatched(PolyglotProxy.LIMIT)));
}
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
isMemberInvocableNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
isMemberInvocableNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0b1000000000000000 /* add-active isMemberInvocable(PolyglotProxy, String, InteropLibrary, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.isMemberInvocable(arg1Value, isMemberInvocableNode__library__, this.executables, isMemberInvocableNode__context__, isMemberInvocableNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public void removeMember(Object arg0Value_, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x10000) != 0 /* is-active removeMember(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary removeMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference removeMemberNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage removeMemberNode__language__ = hostLanguageReference__.get();
arg0Value.removeMember(arg1Value, removeMemberNode__library__, removeMemberNode__context__, removeMemberNode__language__);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
removeMemberNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void removeMemberNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage removeMemberNode__language__ = null;
ContextReference removeMemberNode__context__ = null;
InteropLibrary removeMemberNode__library__ = null;
removeMemberNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
removeMemberNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
removeMemberNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x10000 /* add-active removeMember(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
arg0Value.removeMember(arg1Value, removeMemberNode__library__, removeMemberNode__context__, removeMemberNode__language__);
return;
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isMemberReadable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x20000) != 0 /* is-active isMemberExisting(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isMemberExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isMemberExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isMemberExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isMemberExisting(arg1Value, isMemberExistingNode__library__, isMemberExistingNode__context__, isMemberExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isMemberExistingNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isMemberExistingNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage isMemberExistingNode__language__ = null;
ContextReference isMemberExistingNode__context__ = null;
InteropLibrary isMemberExistingNode__library__ = null;
isMemberExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
isMemberExistingNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
isMemberExistingNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x20000 /* add-active isMemberExisting(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.isMemberExisting(arg1Value, isMemberExistingNode__library__, isMemberExistingNode__context__, isMemberExistingNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isMemberModifiable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x20000) != 0 /* is-active isMemberExisting(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isMemberExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isMemberExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isMemberExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isMemberExisting(arg1Value, isMemberExistingNode__library__, isMemberExistingNode__context__, isMemberExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isMemberExistingNode_AndSpecialize(arg0Value, arg1Value);
}
@Override
public boolean isMemberRemovable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x20000) != 0 /* is-active isMemberExisting(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isMemberExistingNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isMemberExistingNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isMemberExistingNode__language__ = hostLanguageReference__.get();
return arg0Value.isMemberExisting(arg1Value, isMemberExistingNode__library__, isMemberExistingNode__context__, isMemberExistingNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isMemberExistingNode_AndSpecialize(arg0Value, arg1Value);
}
@Override
public boolean isMemberInsertable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x40000) != 0 /* is-active isMemberInsertable(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary isMemberInsertableNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference isMemberInsertableNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage isMemberInsertableNode__language__ = hostLanguageReference__.get();
return arg0Value.isMemberInsertable(arg1Value, isMemberInsertableNode__library__, isMemberInsertableNode__context__, isMemberInsertableNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isMemberInsertableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isMemberInsertableNode_AndSpecialize(PolyglotProxy arg0Value, String arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage isMemberInsertableNode__language__ = null;
ContextReference isMemberInsertableNode__context__ = null;
InteropLibrary isMemberInsertableNode__library__ = null;
isMemberInsertableNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
isMemberInsertableNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
isMemberInsertableNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x40000 /* add-active isMemberInsertable(PolyglotProxy, String, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.isMemberInsertable(arg1Value, isMemberInsertableNode__library__, isMemberInsertableNode__context__, isMemberInsertableNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isDate(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isDate();
}
@Override
public boolean isTime(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isTime();
}
@Override
public boolean isTimeZone(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isTimeZone();
}
@Override
public ZoneId asTimeZone(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x80000) != 0 /* is-active asTimeZone(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asTimeZoneNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asTimeZoneNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asTimeZoneNode__language__ = hostLanguageReference__.get();
return arg0Value.asTimeZone(asTimeZoneNode__library__, asTimeZoneNode__context__, asTimeZoneNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asTimeZoneNode_AndSpecialize(arg0Value);
}
private ZoneId asTimeZoneNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asTimeZoneNode__language__ = null;
ContextReference asTimeZoneNode__context__ = null;
InteropLibrary asTimeZoneNode__library__ = null;
asTimeZoneNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asTimeZoneNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asTimeZoneNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x80000 /* add-active asTimeZone(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asTimeZone(asTimeZoneNode__library__, asTimeZoneNode__context__, asTimeZoneNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public LocalDate asDate(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x100000) != 0 /* is-active asDate(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asDateNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asDateNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asDateNode__language__ = hostLanguageReference__.get();
return arg0Value.asDate(asDateNode__library__, asDateNode__context__, asDateNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asDateNode_AndSpecialize(arg0Value);
}
private LocalDate asDateNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asDateNode__language__ = null;
ContextReference asDateNode__context__ = null;
InteropLibrary asDateNode__library__ = null;
asDateNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asDateNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asDateNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x100000 /* add-active asDate(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asDate(asDateNode__library__, asDateNode__context__, asDateNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public LocalTime asTime(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x200000) != 0 /* is-active asTime(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asTimeNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asTimeNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asTimeNode__language__ = hostLanguageReference__.get();
return arg0Value.asTime(asTimeNode__library__, asTimeNode__context__, asTimeNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asTimeNode_AndSpecialize(arg0Value);
}
private LocalTime asTimeNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asTimeNode__language__ = null;
ContextReference asTimeNode__context__ = null;
InteropLibrary asTimeNode__library__ = null;
asTimeNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asTimeNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asTimeNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x200000 /* add-active asTime(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asTime(asTimeNode__library__, asTimeNode__context__, asTimeNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public Instant asInstant(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x400000) != 0 /* is-active asInstant(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asInstantNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asInstantNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asInstantNode__language__ = hostLanguageReference__.get();
return arg0Value.asInstant(asInstantNode__library__, asInstantNode__context__, asInstantNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asInstantNode_AndSpecialize(arg0Value);
}
private Instant asInstantNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asInstantNode__language__ = null;
ContextReference asInstantNode__context__ = null;
InteropLibrary asInstantNode__library__ = null;
asInstantNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asInstantNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asInstantNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x400000 /* add-active asInstant(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asInstant(asInstantNode__library__, asInstantNode__context__, asInstantNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean isDuration(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).isDuration();
}
@Override
public Duration asDuration(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x800000) != 0 /* is-active asDuration(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */) {
{
InteropLibrary asDurationNode__library__ = (this);
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference asDurationNode__context__ = hostLanguageContextReference__;
LanguageReference hostLanguageReference__ = this.hostLanguageReference_;
HostLanguage asDurationNode__language__ = hostLanguageReference__.get();
return arg0Value.asDuration(asDurationNode__library__, asDurationNode__context__, asDurationNode__language__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return asDurationNode_AndSpecialize(arg0Value);
}
private Duration asDurationNode_AndSpecialize(PolyglotProxy arg0Value) throws UnsupportedMessageException {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
HostLanguage asDurationNode__language__ = null;
ContextReference asDurationNode__context__ = null;
InteropLibrary asDurationNode__library__ = null;
asDurationNode__library__ = (this);
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
asDurationNode__context__ = hostLanguageContextReference__1;
LanguageReference hostLanguageReference__1 = this.hostLanguageReference_;
if (hostLanguageReference__1 == null) {
this.hostLanguageReference_ = hostLanguageReference__1 = super.lookupLanguageReference(HostLanguage.class);
}
asDurationNode__language__ = hostLanguageReference__1.get();
this.state_ = state = state | 0x800000 /* add-active asDuration(PolyglotProxy, InteropLibrary, ContextReference, HostLanguage) */;
lock.unlock();
hasLock = false;
return arg0Value.asDuration(asDurationNode__library__, asDurationNode__context__, asDurationNode__language__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean hasLanguage(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).hasLanguage();
}
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).getLanguage();
}
@Override
public Object toDisplayString(Object arg0Value_, boolean arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x1000000) != 0 /* is-active toDisplayString(PolyglotProxy, boolean, ContextReference) */) {
{
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference toDisplayStringNode__context__ = hostLanguageContextReference__;
return arg0Value.toDisplayString(arg1Value, toDisplayStringNode__context__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return toDisplayStringNode_AndSpecialize(arg0Value, arg1Value);
}
private Object toDisplayStringNode_AndSpecialize(PolyglotProxy arg0Value, boolean arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
ContextReference toDisplayStringNode__context__ = null;
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
toDisplayStringNode__context__ = hostLanguageContextReference__1;
this.state_ = state = state | 0x1000000 /* add-active toDisplayString(PolyglotProxy, boolean, ContextReference) */;
lock.unlock();
hasLock = false;
return arg0Value.toDisplayString(arg1Value, toDisplayStringNode__context__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public boolean hasMetaObject(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PolyglotProxy) receiver)).hasMetaObject();
}
@Override
public Object getMetaObject(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
int state = state_;
if ((state & 0x2000000) != 0 /* is-active getMetaObject(PolyglotProxy, ContextReference) */) {
{
ContextReference hostLanguageContextReference__ = this.hostLanguageContextReference_;
ContextReference getMetaObjectNode__context__ = hostLanguageContextReference__;
return arg0Value.getMetaObject(getMetaObjectNode__context__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getMetaObjectNode_AndSpecialize(arg0Value);
}
private Object getMetaObjectNode_AndSpecialize(PolyglotProxy arg0Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
try {
{
ContextReference getMetaObjectNode__context__ = null;
ContextReference hostLanguageContextReference__1 = this.hostLanguageContextReference_;
if (hostLanguageContextReference__1 == null) {
this.hostLanguageContextReference_ = hostLanguageContextReference__1 = super.lookupContextReference(HostLanguage.class);
}
getMetaObjectNode__context__ = hostLanguageContextReference__1;
this.state_ = state = state | 0x2000000 /* add-active getMetaObject(PolyglotProxy, ContextReference) */;
lock.unlock();
hasLock = false;
return arg0Value.getMetaObject(getMetaObjectNode__context__);
}
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return PolyglotProxy.identityHashCode((((PolyglotProxy) receiver)));
}
private static boolean isIdenticalOrUndefinedFallbackGuard_(int state, PolyglotProxy arg0Value, Object arg1Value) {
if (((state & 0b1)) == 0 /* is-not-active doHostObject(PolyglotProxy, PolyglotProxy) */ && arg1Value instanceof PolyglotProxy) {
return false;
}
return true;
}
}
@GeneratedBy(PolyglotProxy.class)
private static final class Uncached extends InteropLibrary {
private final ContextReference hostLanguageContextReference_ = lookupContextReference(HostLanguage.class);
private final LanguageReference hostLanguageReference_ = lookupLanguageReference(HostLanguage.class);
Uncached() {
}
@TruffleBoundary
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof PolyglotProxy) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof PolyglotProxy;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
if (arg1Value instanceof PolyglotProxy) {
PolyglotProxy arg1Value_ = (PolyglotProxy) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public boolean isInstantiable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isInstantiable();
}
@TruffleBoundary
@Override
public Object instantiate(Object arg0Value_, Object... arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.instantiate(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isExecutable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isExecutable();
}
@TruffleBoundary
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.execute(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isPointer(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isPointer();
}
@TruffleBoundary
@Override
public long asPointer(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asPointer((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean hasArrayElements(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .hasArrayElements();
}
@TruffleBoundary
@Override
public Object readArrayElement(Object arg0Value_, long arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.readArrayElement(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public void writeArrayElement(Object arg0Value_, long arg1Value, Object arg2Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
arg0Value.writeArrayElement(arg1Value, arg2Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
return;
}
@TruffleBoundary
@Override
public void removeArrayElement(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidArrayIndexException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
arg0Value.removeArrayElement(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
return;
}
@TruffleBoundary
@Override
public long getArraySize(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.getArraySize((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isArrayElementReadable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isArrayElementExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isArrayElementModifiable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isArrayElementExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isArrayElementRemovable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isArrayElementExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isArrayElementInsertable(Object arg0Value_, long arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isArrayElementInsertable(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean hasMembers(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .hasMembers();
}
@TruffleBoundary
@Override
public Object getMembers(Object arg0Value_, boolean arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.getMembers(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public Object readMember(Object arg0Value_, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.readMember(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public void writeMember(Object arg0Value_, String arg1Value, Object arg2Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
arg0Value.writeMember(arg1Value, arg2Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
return;
}
@TruffleBoundary
@Override
public Object invokeMember(Object arg0Value_, String arg1Value, Object... arg2Value) throws UnsupportedMessageException, UnsupportedTypeException, ArityException, UnknownIdentifierException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.invokeMember(arg1Value, arg2Value, (this), (INTEROP_LIBRARY_.getUncached()), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isMemberInvocable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isMemberInvocable(arg1Value, (this), (INTEROP_LIBRARY_.getUncached()), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public void removeMember(Object arg0Value_, String arg1Value) throws UnsupportedMessageException, UnknownIdentifierException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
arg0Value.removeMember(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
return;
}
@TruffleBoundary
@Override
public boolean isMemberReadable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isMemberExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isMemberModifiable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isMemberExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isMemberRemovable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isMemberExisting(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isMemberInsertable(Object arg0Value_, String arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.isMemberInsertable(arg1Value, (this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isDate(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isDate();
}
@TruffleBoundary
@Override
public boolean isTime(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isTime();
}
@TruffleBoundary
@Override
public boolean isTimeZone(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isTimeZone();
}
@TruffleBoundary
@Override
public ZoneId asTimeZone(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asTimeZone((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public LocalDate asDate(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asDate((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public LocalTime asTime(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asTime((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public Instant asInstant(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asInstant((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean isDuration(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .isDuration();
}
@TruffleBoundary
@Override
public Duration asDuration(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.asDuration((this), this.hostLanguageContextReference_, this.hostLanguageReference_.get());
}
@TruffleBoundary
@Override
public boolean hasLanguage(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .hasLanguage();
}
@TruffleBoundary
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .getLanguage();
}
@TruffleBoundary
@Override
public Object toDisplayString(Object arg0Value_, boolean arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.toDisplayString(arg1Value, this.hostLanguageContextReference_);
}
@TruffleBoundary
@Override
public boolean hasMetaObject(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PolyglotProxy) receiver) .hasMetaObject();
}
@TruffleBoundary
@Override
public Object getMetaObject(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PolyglotProxy arg0Value = ((PolyglotProxy) arg0Value_);
return arg0Value.getMetaObject(this.hostLanguageContextReference_);
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return PolyglotProxy.identityHashCode(((PolyglotProxy) receiver) );
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy