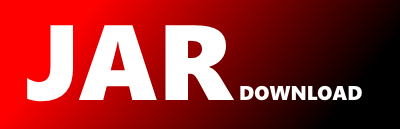
com.oracle.truffle.regex.tregex.nodes.input.InputReadNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
// CheckStyle: start generated
package com.oracle.truffle.regex.tregex.nodes.input;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.regex.runtime.nodes.ToCharNode;
import com.oracle.truffle.regex.runtime.nodes.ToCharNodeGen;
import java.util.concurrent.locks.Lock;
@GeneratedBy(InputReadNode.class)
@SuppressWarnings("unused")
public final class InputReadNodeGen extends InputReadNode {
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@CompilationFinal private volatile int state_;
@CompilationFinal private volatile int exclude_;
@Child private BoxedCharArray0Data boxedCharArray0_cache;
@Child private ToCharNode boxedCharArray1_toCharNode_;
private InputReadNodeGen() {
}
@ExplodeLoop
@Override
public int execute(Object arg0Value, int arg1Value) {
int state = state_;
if (state != 0 /* is-active doBytes(byte[], int) || doString(String, int) || doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) || doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
if ((state & 0b1) != 0 /* is-active doBytes(byte[], int) */ && arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
return InputReadNode.doBytes(arg0Value_, arg1Value);
}
if ((state & 0b10) != 0 /* is-active doString(String, int) */ && arg0Value instanceof String) {
String arg0Value_ = (String) arg0Value;
return InputReadNode.doString(arg0Value_, arg1Value);
}
if ((state & 0b1100) != 0 /* is-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) || doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
if ((state & 0b100) != 0 /* is-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
BoxedCharArray0Data s3_ = this.boxedCharArray0_cache;
while (s3_ != null) {
if ((s3_.inputs_.accepts(arg0Value)) && (s3_.inputs_.hasArrayElements(arg0Value))) {
return InputReadNode.doBoxedCharArray(arg0Value, arg1Value, s3_.inputs_, s3_.toCharNode_);
}
s3_ = s3_.next_;
}
}
if ((state & 0b1000) != 0 /* is-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary boxedCharArray1_inputs__ = (INTEROP_LIBRARY_.getUncached());
if ((boxedCharArray1_inputs__.hasArrayElements(arg0Value))) {
return this.boxedCharArray1Boundary(state, arg0Value, arg1Value);
}
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private int boxedCharArray1Boundary(int state, Object arg0Value, int arg1Value) {
{
InteropLibrary boxedCharArray1_inputs__ = (INTEROP_LIBRARY_.getUncached());
return InputReadNode.doBoxedCharArray(arg0Value, arg1Value, boxedCharArray1_inputs__, this.boxedCharArray1_toCharNode_);
}
}
private int executeAndSpecialize(Object arg0Value, int arg1Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int exclude = exclude_;
try {
if (arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
this.state_ = state = state | 0b1 /* add-active doBytes(byte[], int) */;
lock.unlock();
hasLock = false;
return InputReadNode.doBytes(arg0Value_, arg1Value);
}
if (arg0Value instanceof String) {
String arg0Value_ = (String) arg0Value;
this.state_ = state = state | 0b10 /* add-active doString(String, int) */;
lock.unlock();
hasLock = false;
return InputReadNode.doString(arg0Value_, arg1Value);
}
if ((exclude) == 0 /* is-not-excluded doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
int count3_ = 0;
BoxedCharArray0Data s3_ = this.boxedCharArray0_cache;
if ((state & 0b100) != 0 /* is-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */) {
while (s3_ != null) {
if ((s3_.inputs_.accepts(arg0Value)) && (s3_.inputs_.hasArrayElements(arg0Value))) {
break;
}
s3_ = s3_.next_;
count3_++;
}
}
if (s3_ == null) {
{
InteropLibrary inputs__ = super.insert((INTEROP_LIBRARY_.create(arg0Value)));
// assert (s3_.inputs_.accepts(arg0Value));
if ((inputs__.hasArrayElements(arg0Value)) && count3_ < (2)) {
s3_ = super.insert(new BoxedCharArray0Data(boxedCharArray0_cache));
s3_.inputs_ = s3_.insertAccessor(inputs__);
s3_.toCharNode_ = s3_.insertAccessor((ToCharNode.create()));
this.boxedCharArray0_cache = s3_;
this.state_ = state = state | 0b100 /* add-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */;
}
}
}
if (s3_ != null) {
lock.unlock();
hasLock = false;
return InputReadNode.doBoxedCharArray(arg0Value, arg1Value, s3_.inputs_, s3_.toCharNode_);
}
}
{
InteropLibrary boxedCharArray1_inputs__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
boxedCharArray1_inputs__ = (INTEROP_LIBRARY_.getUncached());
if ((boxedCharArray1_inputs__.hasArrayElements(arg0Value))) {
this.boxedCharArray1_toCharNode_ = super.insert((ToCharNode.create()));
this.exclude_ = exclude = exclude | 0b1 /* add-excluded doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */;
this.boxedCharArray0_cache = null;
state = state & 0xfffffffb /* remove-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */;
this.state_ = state = state | 0b1000 /* add-active doBoxedCharArray(Object, int, InteropLibrary, ToCharNode) */;
lock.unlock();
hasLock = false;
return InputReadNode.doBoxedCharArray(arg0Value, arg1Value, boxedCharArray1_inputs__, this.boxedCharArray1_toCharNode_);
}
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
BoxedCharArray0Data s3_ = this.boxedCharArray0_cache;
if ((s3_ == null || s3_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
public static InputReadNode create() {
return new InputReadNodeGen();
}
public static InputReadNode getUncached() {
return InputReadNodeGen.UNCACHED;
}
@GeneratedBy(InputReadNode.class)
private static final class BoxedCharArray0Data extends Node {
@Child BoxedCharArray0Data next_;
@Child InteropLibrary inputs_;
@Child ToCharNode toCharNode_;
BoxedCharArray0Data(BoxedCharArray0Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
T insertAccessor(T node) {
return super.insert(node);
}
}
@GeneratedBy(InputReadNode.class)
private static final class Uncached extends InputReadNode {
@TruffleBoundary
@Override
public int execute(Object arg0Value, int arg1Value) {
if (arg0Value instanceof byte[]) {
byte[] arg0Value_ = (byte[]) arg0Value;
return InputReadNode.doBytes(arg0Value_, arg1Value);
}
if (arg0Value instanceof String) {
String arg0Value_ = (String) arg0Value;
return InputReadNode.doString(arg0Value_, arg1Value);
}
if (((INTEROP_LIBRARY_.getUncached(arg0Value)).hasArrayElements(arg0Value))) {
return InputReadNode.doBoxedCharArray(arg0Value, arg1Value, (INTEROP_LIBRARY_.getUncached(arg0Value)), (ToCharNodeGen.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy