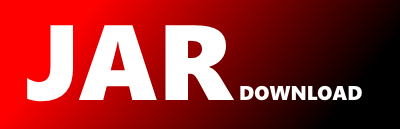
io.nosqlbench.nb.api.markdown.aggregator.ParsedFrontMatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
package io.nosqlbench.nb.api.markdown.aggregator;
import io.nosqlbench.nb.api.markdown.types.DocScope;
import io.nosqlbench.nb.api.markdown.types.FrontMatterInfo;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.security.InvalidParameterException;
import java.util.*;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
public class ParsedFrontMatter implements FrontMatterInfo {
private final static Logger logger = LogManager.getLogger(ParsedFrontMatter.class);
private final Map> data;
public ParsedFrontMatter(Map> data) {
this.data = data;
}
@Override
public String getTitle() {
List titles = data.get(FrontMatterInfo.TITLE);
if (titles==null) {
return "";
}
if (titles.size()!=1) {
throw new InvalidParameterException(FrontMatterInfo.TITLE + " can only contain a single value.");
}
return titles.get(0);
}
@Override
public int getWeight() {
List weights = data.get(FrontMatterInfo.WEIGHT);
if (weights==null) {
return 0;
}
if (weights.size()!=1) {
throw new InvalidParameterException(FrontMatterInfo.WEIGHT + " can only contain a single value.");
}
return Integer.parseInt(weights.get(0));
}
@Override
public Set getTopics() {
List topics = data.get(FrontMatterInfo.TOPICS);
Set topicSet = new HashSet<>();
if (topics!=null) {
for (String topic : topics) {
Collections.addAll(topicSet, topic.split(", *"));
}
// topicSet.addAll(topics);
}
return topicSet;
}
@Override
public List getIncluded() {
List included = data.get(FrontMatterInfo.INCLUDED);
List includedList = new ArrayList<>();
if (included!=null) {
for (String s : included) {
Collections.addAll(includedList, s.split(", *"));
}
}
return includedList;
}
@Override
public List getAggregations() {
if (!data.containsKey(FrontMatterInfo.AGGREGATE)) {
return List.of();
}
List patterns = new ArrayList<>();
for (String aggName : data.get(FrontMatterInfo.AGGREGATE)) {
Collections.addAll(patterns,aggName.split(", *"));
}
return patterns.stream().map(Pattern::compile).collect(Collectors.toList());
}
@Override
public Set getDocScopes() {
if (!data.containsKey(FrontMatterInfo.SCOPES)) {
return Set.of(DocScope.NONE);
}
List scopeNames = new ArrayList<>();
for (String scopeName : data.get(FrontMatterInfo.SCOPES)) {
Collections.addAll(scopeNames,scopeName.split(", *"));
}
return scopeNames.stream().map(DocScope::valueOf).collect(Collectors.toSet());
}
public List getDiagnostics() {
List warnings = new ArrayList<>();
for (String propname : data.keySet()) {
if (!FrontMatterInfo.FrontMatterKeyWords.contains(propname)) {
warnings.add("unrecognized frontm atter property " + propname);
}
}
return warnings;
}
public void setTopics(Set newTopics) {
// TODO: allow functional version of this
// this.data.put(FrontMatterInfo.TOPICS,newTopics);
}
public ParsedFrontMatter withTopics(List assigning) {
HashMap> newmap = new HashMap<>();
newmap.putAll(this.data);
newmap.put(FrontMatterInfo.TOPICS,assigning);
return new ParsedFrontMatter(newmap);
}
public ParsedFrontMatter withIncluded(List included) {
HashMap> newmap = new HashMap<>();
newmap.putAll(this.data);
newmap.put(FrontMatterInfo.INCLUDED,included);
return new ParsedFrontMatter(newmap);
}
@Override
public String toString() {
return "ParsedFrontMatter{" +
"data=" + data +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ParsedFrontMatter that = (ParsedFrontMatter) o;
return Objects.equals(data, that.data);
}
@Override
public int hashCode() {
return Objects.hash(data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy