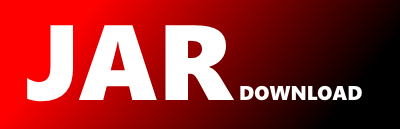
oshi.hardware.HWPartition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
/**
* MIT License
*
* Copyright (c) 2010 - 2020 The OSHI Project Contributors: https://github.com/oshi/oshi/graphs/contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package oshi.hardware;
import oshi.annotation.concurrent.Immutable;
import oshi.util.FormatUtil;
/**
* A region on a hard disk or other secondary storage, so that an operating
* system can manage information in each region separately. A partition appears
* in the operating system as a distinct "logical" disk that uses part of the
* actual disk.
*/
@Immutable
public class HWPartition {
private final String identification;
private final String name;
private final String type;
private final String uuid;
private final long size;
private final int major;
private final int minor;
private final String mountPoint;
/**
* Creates a new HWPartition
*
* @param identification
* The unique partition id
* @param name
* Friendly name of the partition
* @param type
* Type or description of the partition
* @param uuid
* UUID
* @param size
* Size in bytes
* @param major
* Device ID (Major)
* @param minor
* Device ID (Minor)
* @param mountPoint
* Where the partition is mounted
*/
public HWPartition(String identification, String name, String type, String uuid, long size, int major, int minor,
String mountPoint) {
this.identification = identification;
this.name = name;
this.type = type;
this.uuid = uuid;
this.size = size;
this.major = major;
this.minor = minor;
this.mountPoint = mountPoint;
}
/**
*
* Getter for the field identification
.
*
*
* @return Returns the identification.
*/
public String getIdentification() {
return this.identification;
}
/**
*
* Getter for the field name
.
*
*
* @return Returns the name.
*/
public String getName() {
return this.name;
}
/**
*
* Getter for the field type
.
*
*
* @return Returns the type.
*/
public String getType() {
return this.type;
}
/**
*
* Getter for the field uuid
.
*
*
* @return Returns the uuid.
*/
public String getUuid() {
return this.uuid;
}
/**
*
* Getter for the field size
.
*
*
* @return Returns the size in bytes.
*/
public long getSize() {
return this.size;
}
/**
*
* Getter for the field major
.
*
*
* @return Returns the major device ID.
*/
public int getMajor() {
return this.major;
}
/**
*
* Getter for the field minor
.
*
*
* @return Returns the minor device ID.
*/
public int getMinor() {
return this.minor;
}
/**
*
* Getter for the field mountPoint
.
*
*
* @return Returns the mount point.
*/
public String getMountPoint() {
return this.mountPoint;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(getIdentification()).append(": ");
sb.append(getName()).append(" ");
sb.append("(").append(getType()).append(") ");
sb.append("Maj:Min=").append(getMajor()).append(":").append(getMinor()).append(", ");
sb.append("size: ").append(FormatUtil.formatBytesDecimal(getSize()));
sb.append(getMountPoint().isEmpty() ? "" : " @ " + getMountPoint());
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy