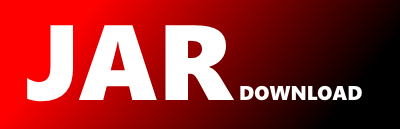
oshi.hardware.common.AbstractNetworkIF Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-cql-shaded Show documentation
Show all versions of driver-cql-shaded Show documentation
A Shaded CQL ActivityType driver for http://nosqlbench.io/
/**
* MIT License
*
* Copyright (c) 2010 - 2020 The OSHI Project Contributors: https://github.com/oshi/oshi/graphs/contributors
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package oshi.hardware.common;
import static oshi.util.Memoizer.memoize;
import java.net.InetAddress;
import java.net.InterfaceAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import java.util.function.Supplier;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import oshi.annotation.concurrent.ThreadSafe;
import oshi.hardware.NetworkIF;
import oshi.util.FileUtil;
import oshi.util.FormatUtil;
/**
* Network interfaces implementation.
*/
@ThreadSafe
public abstract class AbstractNetworkIF implements NetworkIF {
private static final Logger LOG = LoggerFactory.getLogger(AbstractNetworkIF.class);
private static final String OSHI_VM_MAC_ADDR_PROPERTIES = "oshi.vmmacaddr.properties";
private NetworkInterface networkInterface;
private int mtu;
private String mac;
private String[] ipv4;
private Short[] subnetMasks;
private String[] ipv6;
private Short[] prefixLengths;
private final Supplier vmMacAddrProps = memoize(AbstractNetworkIF::queryVmMacAddrProps);
/**
* Construct a {@link NetworkIF} object backed by the specified
* {@link NetworkInterface}.
*
* @param netint
* The core java {@link NetworkInterface} backing this object.
*/
protected AbstractNetworkIF(NetworkInterface netint) {
this.networkInterface = netint;
try {
// Set MTU
this.mtu = networkInterface.getMTU();
// Set MAC
byte[] hwmac = networkInterface.getHardwareAddress();
if (hwmac != null) {
List octets = new ArrayList<>(6);
for (byte b : hwmac) {
octets.add(String.format("%02x", b));
}
this.mac = String.join(":", octets);
} else {
this.mac = "Unknown";
}
// Set IP arrays
ArrayList ipv4list = new ArrayList<>();
ArrayList subnetMaskList = new ArrayList<>();
ArrayList ipv6list = new ArrayList<>();
ArrayList prefixLengthList = new ArrayList<>();
for (InterfaceAddress interfaceAddress : networkInterface.getInterfaceAddresses()) {
InetAddress address = interfaceAddress.getAddress();
if (address.getHostAddress().length() > 0) {
if (address.getHostAddress().contains(":")) {
ipv6list.add(address.getHostAddress().split("%")[0]);
prefixLengthList.add(interfaceAddress.getNetworkPrefixLength());
} else {
ipv4list.add(address.getHostAddress());
subnetMaskList.add(interfaceAddress.getNetworkPrefixLength());
}
}
}
this.ipv4 = ipv4list.toArray(new String[0]);
this.subnetMasks = subnetMaskList.toArray(new Short[0]);
this.ipv6 = ipv6list.toArray(new String[0]);
this.prefixLengths = prefixLengthList.toArray(new Short[0]);
} catch (SocketException e) {
LOG.error("Socket exception: {}", e.getMessage());
}
}
/**
* Returns network interfaces that are not Loopback, and have a hardware
* address.
*
* @return A list of network interfaces
*/
protected static List getNetworkInterfaces() {
List result = new ArrayList<>();
Enumeration interfaces = null;
try {
interfaces = NetworkInterface.getNetworkInterfaces(); // can return null
} catch (SocketException ex) {
LOG.error("Socket exception when retrieving interfaces: {}", ex.getMessage());
}
if (interfaces != null) {
while (interfaces.hasMoreElements()) {
NetworkInterface netint = interfaces.nextElement();
try {
if (!netint.isLoopback() && netint.getHardwareAddress() != null) {
result.add(netint);
}
} catch (SocketException ex) {
LOG.error("Socket exception when retrieving interface \"{}\": {}", netint.getName(),
ex.getMessage());
}
}
}
return result;
}
@Override
public NetworkInterface queryNetworkInterface() {
return this.networkInterface;
}
@Override
public String getName() {
return this.networkInterface.getName();
}
@Override
public String getDisplayName() {
return this.networkInterface.getDisplayName();
}
@Override
public int getMTU() {
return this.mtu;
}
@Override
public String getMacaddr() {
return this.mac;
}
@Override
public String[] getIPv4addr() {
return Arrays.copyOf(this.ipv4, this.ipv4.length);
}
@Override
public Short[] getSubnetMasks() {
return Arrays.copyOf(this.subnetMasks, this.subnetMasks.length);
}
@Override
public String[] getIPv6addr() {
return Arrays.copyOf(this.ipv6, this.ipv6.length);
}
@Override
public Short[] getPrefixLengths() {
return Arrays.copyOf(this.prefixLengths, this.prefixLengths.length);
}
@Override
public boolean isKnownVmMacAddr() {
String oui = getMacaddr().length() > 7 ? getMacaddr().substring(0, 8) : getMacaddr();
return this.vmMacAddrProps.get().containsKey(oui.toUpperCase());
}
@Override
public int getIfType() {
// default
return 0;
}
@Override
public int getNdisPhysicalMediumType() {
// default
return 0;
}
@Override
public boolean isConnectorPresent() {
// default
return false;
}
private static Properties queryVmMacAddrProps() {
return FileUtil.readPropertiesFromFilename(OSHI_VM_MAC_ADDR_PROPERTIES);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("Name: ").append(getName()).append(" ").append("(").append(getDisplayName()).append(")").append("\n");
sb.append(" MAC Address: ").append(getMacaddr()).append("\n");
sb.append(" MTU: ").append(getMTU()).append(", ").append("Speed: ").append(getSpeed()).append("\n");
String[] ipv4withmask = getIPv4addr();
if (this.ipv4.length == this.subnetMasks.length) {
for (int i = 0; i < this.subnetMasks.length; i++) {
ipv4withmask[i] += "/" + this.subnetMasks[i];
}
}
sb.append(" IPv4: ").append(Arrays.toString(ipv4withmask)).append("\n");
String[] ipv6withprefixlength = getIPv6addr();
if (this.ipv6.length == this.prefixLengths.length) {
for (int j = 0; j < this.prefixLengths.length; j++) {
ipv6withprefixlength[j] += "/" + this.prefixLengths[j];
}
}
sb.append(" IPv6: ").append(Arrays.toString(ipv6withprefixlength)).append("\n");
sb.append(" Traffic: received ").append(getPacketsRecv()).append(" packets/")
.append(FormatUtil.formatBytes(getBytesRecv())).append(" (" + getInErrors() + " err, ")
.append(getInDrops() + " drop);");
sb.append(" transmitted ").append(getPacketsSent()).append(" packets/")
.append(FormatUtil.formatBytes(getBytesSent())).append(" (" + getOutErrors() + " err, ")
.append(getCollisions() + " coll);");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy