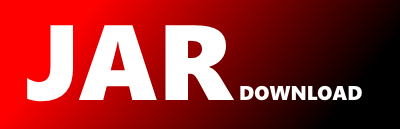
io.nosqlbench.engine.api.activityapi.planning.Sequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of engine-api Show documentation
Show all versions of engine-api Show documentation
The engine API for nosqlbench;
Provides the interfaces needed to build internal modules for the
nosqlbench core engine
package io.nosqlbench.engine.api.activityapi.planning;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Sequence implements OpSequence {
private final SequencerType type;
private final List elems;
private final int[] seq;
Sequence(SequencerType type, List elems, int[] seq) {
this.type = type;
this.elems = elems;
this.seq = seq;
}
@Override
public T get(long selector) {
int index = (int) (selector % seq.length);
index = seq[index];
return elems.get(index);
}
@Override
public List getOps() {
return elems;
}
@Override
public int[] getSequence() {
return seq;
}
public SequencerType getSequencerType() {
return type;
}
@Override
public Sequence transform(Function func) {
return new Sequence(type, elems.stream().map(func).collect(Collectors.toList()), seq);
}
@Override
public String toString() {
return Arrays.toString(this.seq);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy