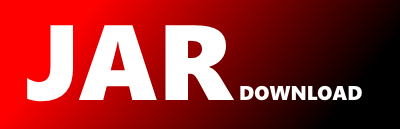
io.nosqlbench.engine.api.activityconfig.rawyaml.StatementsOwner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of engine-api Show documentation
Show all versions of engine-api Show documentation
The engine API for nosqlbench;
Provides the interfaces needed to build internal modules for the
nosqlbench core engine
/*
*
* Copyright 2016 jshook
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* /
*/
package io.nosqlbench.engine.api.activityconfig.rawyaml;
import io.nosqlbench.nb.api.errors.BasicError;
import java.util.*;
public class StatementsOwner extends RawStmtFields {
private final static List stmtsFieldNames = List.of("op","ops","operation","statement","statements");
private List rawStmtDefs = new ArrayList<>();
public StatementsOwner() {
}
public List getRawStmtDefs() {
return rawStmtDefs;
}
public void setRawStmtDefs(List rawStmtDefs) {
this.rawStmtDefs = rawStmtDefs;
}
public void setFieldsByReflection(Map propsmap) {
HashSet found = new HashSet<>();
for (String fname : stmtsFieldNames) {
if (propsmap.containsKey(fname)) {
found.add(fname);
}
}
if (found.size()>1) {
throw new BasicError("You used " + found + " as an op name, but only one of these is allowed.");
}
if (found.size()==1) {
Object stmtsFieldValue = propsmap.remove(found.iterator().next());
setStatementsFieldByType(stmtsFieldValue);
}
super.setFieldsByReflection(propsmap);
}
@SuppressWarnings("unchecked")
public void setStatementsFieldByType(Object object) {
// TODO: Re-plumb this code path with clarity around structural traversal
// LinkedHashMap> namedStatementMaps = new LinkedHashMap<>();
// if (object instanceof List) {
// List> entries = (ArrayList)object;
// for (Object entry : entries) {
//
// }
// }
//
if (object instanceof List) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy