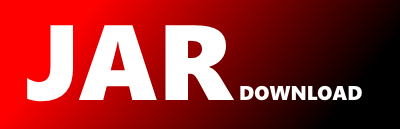
io.nosqlbench.engine.api.activityconfig.yaml.OpDef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of engine-api Show documentation
Show all versions of engine-api Show documentation
The engine API for nosqlbench;
Provides the interfaces needed to build internal modules for the
nosqlbench core engine
/*
*
* Copyright 2016 jshook
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* /
*/
package io.nosqlbench.engine.api.activityconfig.yaml;
import io.nosqlbench.engine.api.activityconfig.MultiMapLookup;
import io.nosqlbench.engine.api.activityconfig.rawyaml.RawStmtDef;
import io.nosqlbench.nb.api.errors.BasicError;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
public class OpDef extends OpTemplate {
private final static Logger logger = LogManager.getLogger(OpDef.class);
private final RawStmtDef rawStmtDef;
private final StmtsBlock block;
private final LinkedHashMap params;
private final LinkedHashMap bindings;
private final LinkedHashMap tags;
public OpDef(StmtsBlock block, RawStmtDef rawStmtDef) {
this.block = block;
this.rawStmtDef = rawStmtDef;
this.params = composeParams();
this.bindings = composeBindings();
this.tags = composeTags();
}
@Override
public String getName() {
return block.getName() + "--" + rawStmtDef.getName();
}
@Override
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy