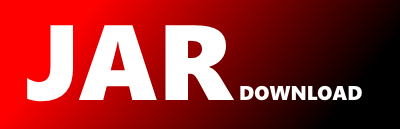
io.nosqlbench.engine.api.activityconfig.yaml.StmtsBlock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of engine-api Show documentation
Show all versions of engine-api Show documentation
The engine API for nosqlbench;
Provides the interfaces needed to build internal modules for the
nosqlbench core engine
/*
*
* Copyright 2016 jshook
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* /
*/
package io.nosqlbench.engine.api.activityconfig.yaml;
import io.nosqlbench.engine.api.activityconfig.MultiMapLookup;
import io.nosqlbench.engine.api.activityconfig.rawyaml.RawStmtDef;
import io.nosqlbench.engine.api.activityconfig.rawyaml.RawStmtsBlock;
import io.nosqlbench.engine.api.util.Tagged;
import org.jetbrains.annotations.NotNull;
import java.util.*;
public class StmtsBlock implements Tagged, Iterable {
private final RawStmtsBlock rawStmtsBlock;
private final StmtsDoc rawStmtsDoc;
private final int blockIdx;
public StmtsBlock(RawStmtsBlock rawStmtsBlock, StmtsDoc rawStmtsDoc, int blockIdx) {
this.rawStmtsBlock = rawStmtsBlock;
this.rawStmtsDoc = rawStmtsDoc;
this.blockIdx = blockIdx;
}
public List getOps() {
List rawOpTemplates = new ArrayList<>();
List statements = rawStmtsBlock.getRawStmtDefs();
for (int i = 0; i < statements.size(); i++) {
rawOpTemplates.add(
new OpDef(this, statements.get(i))
);
}
return rawOpTemplates;
}
public String getName() {
StringBuilder sb = new StringBuilder();
if (!rawStmtsDoc.getName().isEmpty()) {
sb.append(rawStmtsDoc.getName()).append("--");
}
if (!rawStmtsBlock.getName().isEmpty()) {
sb.append(rawStmtsBlock.getName());
} else {
sb.append("block").append(blockIdx);
}
return sb.toString();
}
public Map getTags() {
return new MultiMapLookup<>(rawStmtsBlock.getTags(), rawStmtsDoc.getTags());
}
public Map getParams() {
return new MultiMapLookup<>(rawStmtsBlock.getParams(), rawStmtsDoc.getParams());
}
public Map getParamsAsText() {
MultiMapLookup
© 2015 - 2024 Weber Informatics LLC | Privacy Policy