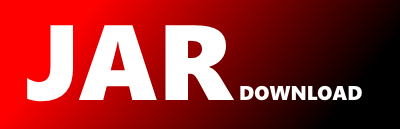
io.nosqlbench.nb.api.config.standard.Param Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nb-api Show documentation
Show all versions of nb-api Show documentation
The top level API module for NoSQLBench. This module should have no internal
module dependencies other than the mvn-default module.
All modules within NoSQLBench can safely depend on this module with circular
dependencies. This module provides cross-cutting code infrastracture, such as
path utilities and ways of describing services used between modules.
It is also the transitive aggregation point for system-wide library dependencies
for logging and testing or similar needs.
package io.nosqlbench.nb.api.config.standard;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* A configuration element describes a single configurable parameter.
*
* @param The type of value which can be stored in this named configuration
* parameter in in actual configuration data.
*/
public class Param {
private final List names;
public final Class extends T> type;
private String description;
private final T defaultValue;
public boolean required;
private Pattern regex;
public Param(
List names,
Class extends T> type,
String description,
boolean required,
T defaultValue
) {
this.names = names;
this.type = type;
this.description = description;
this.required = required;
this.defaultValue = defaultValue;
}
/**
* Declare an optional String parameter with the given name.
*
* @param name the name of the parameter
*/
public static Param optional(String name) {
return optional(List.of(name), String.class);
}
/**
* Declare an optional String parameter specified by any of the names. They act as synonyms.
* When users provide more than one of these in configuration data, it is considered an error.
*
* @param names one or more names that the parameter can be specified with.
*/
public static Param optional(List names) {
return optional(names, String.class);
}
/**
* Declare an optional parameter specified by any of the names which must be assignable to
* (returnable as) the specified type.
* When users provide more than one of these in configuration data, it is considered an error.
*
* @param names one or more names that the parameter can be specified with.
* @param type The type of value that the provided configuration value must be returnable as (assignable to)
* @param Generic type for inference.
*/
public static Param optional(List names, Class type) {
return new Param(names, type, null, false, null);
}
/**
* Declare an optional parameter specified by any of the names which must be assignable to
* (returnable as) the specified type.
* When users provide more than one of these in configuration data, it is considered an error.
*
* @param names one or more names that the parameter can be specified with.
* @param type The type of value that the provided configuration value must be returnable as (assignable to)
* @param description A description of what this parameter is
* @param Generic type for inference.
*/
public static Param optional(List names, Class type, String description) {
return new Param(names, type, description, false, null);
}
/**
* Declare an optional parameter for the given name which must be assignable to
* (returnable as) the specified type.
* When users provide more than one of these in configuration data, it is considered an error.
*
* @param name the name of the parameter
* @param type The type of value that the provided configuration value must be returnable as (assignable to)
* @param Generic type for inference.
*/
public static Param optional(String name, Class type) {
return new Param(List.of(name), type, null, false, null);
}
/**
* Declare an optional parameter for the given name which must be assignable to
* (returnable as) the specified type.
* When users provide more than one of these in configuration data, it is considered an error.
*
* @param name the name of the parameter
* @param type The type of value that the provided configuration value must be returnable as (assignable to)
* @param description A description of what this parameter is
* @param Generic type for inference.
*/
public static Param optional(String name, Class type, String description) {
return new Param(List.of(name), type, description, false, null);
}
/**
* Parameters which are given a default value are automatically marked as required, as the default
* value allows them to be accessed as such.
*
* @param name
* @param defaultValue
* @param
* @return
*/
public static Param defaultTo(String name, V defaultValue) {
return new Param(List.of(name), (Class) defaultValue.getClass(), null, true, defaultValue);
}
/**
* Parameters which are given a default value are automatically marked as required, as the default
* value allows them to be accessed as such.
*
* @param name
* @param defaultValue
* @param
* @return
*/
public static Param defaultTo(String name, V defaultValue, String description) {
return new Param(List.of(name), (Class) defaultValue.getClass(), description, true, defaultValue);
}
/**
* Parameters which are given a default value are automatically marked as required, as the default
* value allows them to be accessed as such.
*
* @param names
* @param defaultValue
* @param
* @return
*/
public static Param defaultTo(List names, V defaultValue) {
return new Param(names, (Class) defaultValue.getClass(), null, true, defaultValue);
}
public static Param required(String name, Class type) {
return new Param(List.of(name), type, null, true, null);
}
public static Param required(List names, Class type) {
return new Param(names, type, null, true, null);
}
@Override
public String toString() {
return "Element{" +
"names='" + names.toString() + '\'' +
", type=" + type +
", description='" + description + '\'' +
", required=" + required +
", defaultValue = " + defaultValue +
'}';
}
public List getNames() {
return names;
}
public Class> getType() {
return type;
}
public String getDescription() {
return description;
}
public boolean isRequired() {
return required;
}
public Param> setRequired(boolean required) {
this.required = required;
return this;
}
public T getDefaultValue() {
return defaultValue;
}
public Param setDescription(String description) {
this.description = description;
return this;
}
public Param setRegex(Pattern regex) {
this.regex = regex;
return this;
}
public Param setRegex(String pattern) {
this.regex = Pattern.compile(pattern);
return this;
}
public Pattern getRegex() {
return regex;
}
public CheckResult validate(Object value) {
if (value == null) {
if (isRequired()) {
return CheckResult.INVALID(this, null, "Value is null but " + this.getNames() + " is required");
} else {
return CheckResult.VALID(this, null, "Value is null, but " + this.getNames() + " is not required");
}
}
if (!this.getType().isAssignableFrom(value.getClass())) {
return CheckResult.INVALID(this, value, "Can't assign " + value.getClass().getSimpleName() + " to " + "" +
this.getType().getSimpleName());
}
if (getRegex() != null) {
if (value instanceof CharSequence) {
Matcher matcher = getRegex().matcher(value.toString());
if (!matcher.matches()) {
return CheckResult.INVALID(this, value,
"Could not match required pattern (" + getRegex().toString() +
") with value '" + value + "' for field '" + getNames() + "'");
}
}
}
return CheckResult.VALID(this, value, "All validators passed for field '" + getNames() + "'");
}
public final static class CheckResult {
public final Param element;
public final Object value;
public final String message;
private final boolean isValid;
private CheckResult(Param e, Object value, String message, boolean isValid) {
this.element = e;
this.value = value;
this.message = message;
this.isValid = isValid;
}
public static CheckResult VALID(Param element, Object value, String message) {
return new CheckResult<>(element, value, message, true);
}
public static CheckResult VALID(Param element, Object value) {
return new CheckResult<>(element, value, "", true);
}
public static CheckResult INVALID(Param element, Object value, String message) {
return new CheckResult<>(element, value, message, false);
}
public boolean isValid() {
return isValid;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy