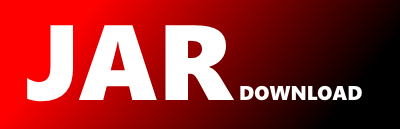
io.nosqlbench.nb.api.content.NBPathsAPI Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nb-api Show documentation
Show all versions of nb-api Show documentation
The top level API module for NoSQLBench. This module should have no internal
module dependencies other than the mvn-default module.
All modules within NoSQLBench can safely depend on this module with circular
dependencies. This module provides cross-cutting code infrastracture, such as
path utilities and ways of describing services used between modules.
It is also the transitive aggregation point for system-wide library dependencies
for logging and testing or similar needs.
package io.nosqlbench.nb.api.content;
import java.nio.file.Path;
import java.util.List;
import java.util.Optional;
public interface NBPathsAPI {
interface Facets extends
GetSource, GetPrefix, GetName, GetExtension, DoSearch {
}
interface GetSource {
/**
* Only provide content from the class path and the local filesystem.
*
* @return this builder
*/
GetPrefix localContent();
/**
* Only return content from remote URLs. If the user is providing non-URL content
* in this context, it is an error. Throw an error in that case.
*
* @return this builder
*/
GetPrefix remoteContent();
/**
* Only return content from the runtime classpath, internal resources that are bundled,
* and do not return content on the file system.
*
* @return this builder
*/
GetPrefix internalContent();
/**
* Only return content from the filesystem, but not remote URLs nor internal bundled resources.
*
* @return this builder
*/
GetPrefix fileContent();
/**
* Return content from everywhere, from remote URls, or from the file system and then the internal
* bundled content if not found in the file system first.
*
* @return this builder
*/
GetPrefix allContent();
}
interface GetPrefix extends GetName {
/**
* Each of the prefix paths will be searched if the resource is not found with the exact
* path given. To be specific, if you want to search within a location based on wildcards,
* you must provide a prefix that provides a boundary for the search.
*
* @param prefixPaths A list of paths to include in the search
* @return this builder
*/
GetPrefix prefix(String... prefixPaths);
}
interface GetName extends GetExtension {
/**
* Provide the names of the resources to be resolved. More than one resource may be provided.
* If no name is provided, then a wildcard search is assumed.
*
* @param name The name of the resource to load
* @return this builder
*/
GetExtension name(String... name);
/**
* Provide a combined prefix, name and suffix in a combined form. For each search template provided,
* the value is sliced up into the three components (prefix, name, extension) and added as if they
* were specified separately using the following rules:
*
* - Any suffix like
{@code .name}
is stripped off as the extension.
* - Any literal (non-pattern) path parts are taken as the prefix.
* - The remainder is taken as the name.
*
*
* Examples:
*
* - {@code my/prefix/path/..?/name.txt} yields:
*
* - prefix: {@code my/prefix/path/}
* - name: {@code ..?/name}
* - extension: {@code .txt}
*
*
* - {@code .*.yaml} yeilds:
*
* - prefix: {@code ./}
* - name: {@code .*}
* - extension: {@code .yaml}
*
*
*
*
* @param name
* @return
*/
DoSearch search(String... name);
}
interface GetExtension extends DoSearch {
/**
* provide a list of optional file extensions which should be considered. If the content is
* not found under the provided name, then each of the extension is tried in order.
* Any provided names are combined with the extensions to create an expanded list of
* paths to search for. if extensions are provided without a name, then wildcards are created
* with the extensions as suffix patterns.
*
* @param extensions The extension names to try
* @return this builder
*/
DoSearch extension(String... extensions);
}
interface DoSearch {
/**
* Return the result of resolving the resource.
*
* @return an optional {@code Content>} element.
*/
Optional> first();
/**
* Return the result of resolving each of the resource names given. This has the same semantics
* of {@link #first()}, except that it returns a result pair-wise for each name given.
*
* @return A list of optional {@code Content>} elements.
*/
List>> resolveEach();
/**
* Provide a list of all matching content that was matched by the search qualifiers
*
* @return a list of content
*/
List> list();
/**
* Return a list of paths which are comprised of the relative part
* once the provided base has been removed from the front. This is done
* per content item within the direct filesystem the path belongs to.
*
* @param base The root path elements to remove
* @return Relative paths
*/
List relativeTo(String... base);
/**
* Find exactly one source of content under the search parameters given.
* It is an error if you find none, or more than one.
*
* @return An optional content element.
*/
Content> one();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy