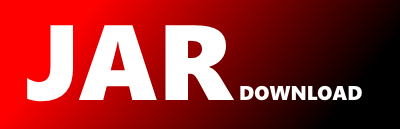
io.nosqlbench.nb.api.content.URIResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nb-api Show documentation
Show all versions of nb-api Show documentation
The top level API module for NoSQLBench. This module should have no internal
module dependencies other than the mvn-default module.
All modules within NoSQLBench can safely depend on this module with circular
dependencies. This module provides cross-cutting code infrastracture, such as
path utilities and ways of describing services used between modules.
It is also the transitive aggregation point for system-wide library dependencies
for logging and testing or similar needs.
package io.nosqlbench.nb.api.content;
import io.nosqlbench.nb.api.errors.BasicError;
import org.apache.commons.math3.FieldElement;
import java.net.URI;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
/**
* This is a stateful search object for resources like Paths or URLs.
* It provides the abilitiy to look for URIs in any form, with simple
* pluggable search back-ends, in some preferential order.
*/
public class URIResolver implements ContentResolver {
private List loaders = new ArrayList<>();
private static List EVERYWHERE = List.of(
ResolverForURL.INSTANCE,
ResolverForFilesystem.INSTANCE,
ResolverForClasspath.INSTANCE
);
private List extensions;
private List extraPaths;
public URIResolver() {
}
/**
* Include resources from all known places, including remote URLs,
* the local default filesystem, and the classpath, which includes
* the jars that hold the current runtime application.
* @return this URISearch
*/
public URIResolver all() {
loaders = EVERYWHERE;
return this;
}
/**
* Includ resources in the default filesystem
* @return this URISearch
*/
public URIResolver inFS() {
loaders.add(ResolverForFilesystem.INSTANCE);
return this;
}
/**
* Include resources in remote URLs
* @return this URISearch
*/
public URIResolver inURLs() {
loaders.add(ResolverForURL.INSTANCE);
return this;
}
/**
* Include resources within the classpath.
* @return this URISearch
*/
public URIResolver inCP() {
loaders.add(ResolverForClasspath.INSTANCE);
return this;
}
public List> resolve(String uri) {
return resolve(URI.create(uri));
}
@Override
public List resolveDirectory(URI uri) {
List dirs = new ArrayList<>();
for (ContentResolver loader : loaders) {
dirs.addAll(loader.resolveDirectory(uri));
}
return dirs;
}
public List> resolve(URI uri) {
List> resolved = new ArrayList<>();
for (ContentResolver loader : loaders) {
List> contents = loader.resolve(uri);
resolved.addAll(contents);
}
return resolved;
}
public List> resolveAll(String uri) {
return resolveAll(URI.create(uri));
}
public List> resolveAll(URI uri) {
List> allFound = new ArrayList<>();
for (ContentResolver loader : loaders) {
allFound.addAll(loader.resolve(uri));
}
return allFound;
}
public URIResolver extension(String extension) {
this.extensions = this.extensions==null ? new ArrayList<>() : this.extensions;
this.extensions.add(extension);
return this;
}
public URIResolver extraPaths(String extraPath) {
this.extraPaths = this.extraPaths==null ? new ArrayList<>() : this.extraPaths;
this.extraPaths.add(Path.of(extraPath));
return this;
}
public Content> resolveOne(String candidatePath) {
List> contents = resolveAll(candidatePath);
if (contents.size()==1) {
return contents.get(0);
}
if (contents.size()==0) {
return null;
}
throw new BasicError("Error while loading content '" + candidatePath +"', only one is allowed, but " + contents.size() + " were found");
}
public String toString() {
return "[resolver]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy