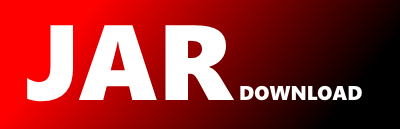
org.apache.maven.plugin.registry.PluginRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
/*
=================== DO NOT EDIT THIS FILE ====================
Generated by Modello 1.0.1 on 2009-08-06 15:14:41,
any modifications will be overwritten.
==============================================================
*/
package org.apache.maven.plugin.registry;
/**
* Root element of the plugin registry file.
*
* @version $Revision$ $Date$
*/
public class PluginRegistry
extends TrackableBase
implements java.io.Serializable
{
//--------------------------/
//- Class/Member Variables -/
//--------------------------/
/**
*
* Specifies how often to check for plugin updates.
* Valid values are: never, always, interval:XXX.
* For the interval specification, XXX denotes a
* terse interval specification, such as 4h.
* Where h=hours, m=minutes, d=days, w=weeks. The
* interval period should be specified in descending
* order of granularity, like this: '[n]w [n]d [n]h
* [n]m'. Any omitted level of granularity will be
* assumed to be a zero value.
*
*/
private String updateInterval = "never";
/**
* Specifies whether the user should be prompted to update
* plugins.
*/
private String autoUpdate;
/**
* Whether to resolve plugin versions using LATEST metadata.
*/
private String checkLatest;
/**
* Field plugins.
*/
private java.util.List plugins;
/**
* Field modelEncoding.
*/
private String modelEncoding = "UTF-8";
//-----------/
//- Methods -/
//-----------/
/**
* Method addPlugin.
*
* @param plugin
*/
public void addPlugin( Plugin plugin )
{
if ( !(plugin instanceof Plugin) )
{
throw new ClassCastException( "PluginRegistry.addPlugins(plugin) parameter must be instanceof " + Plugin.class.getName() );
}
getPlugins().add( plugin );
} //-- void addPlugin( Plugin )
/**
* Get specifies whether the user should be prompted to update
* plugins.
*
* @return String
*/
public String getAutoUpdate()
{
return this.autoUpdate;
} //-- String getAutoUpdate()
/**
* Get whether to resolve plugin versions using LATEST
* metadata.
*
* @return String
*/
public String getCheckLatest()
{
return this.checkLatest;
} //-- String getCheckLatest()
/**
* Method getModelEncoding.
*
* @return the current encoding used when reading/writing this
* model
*/
public String getModelEncoding()
{
return modelEncoding;
} //-- String getModelEncoding()
/**
* Method getPlugins.
*
* @return List
*/
public java.util.List getPlugins()
{
if ( this.plugins == null )
{
this.plugins = new java.util.ArrayList();
}
return this.plugins;
} //-- java.util.List getPlugins()
/**
* Get specifies how often to check for plugin updates. Valid
* values are: never, always, interval:XXX.
* For the interval specification, XXX denotes a
* terse interval specification, such as 4h.
* Where h=hours, m=minutes, d=days, w=weeks. The
* interval period should be specified in descending
* order of granularity, like this: '[n]w [n]d [n]h
* [n]m'. Any omitted level of granularity will be
* assumed to be a zero value.
*
* @return String
*/
public String getUpdateInterval()
{
return this.updateInterval;
} //-- String getUpdateInterval()
/**
* Method removePlugin.
*
* @param plugin
*/
public void removePlugin( Plugin plugin )
{
if ( !(plugin instanceof Plugin) )
{
throw new ClassCastException( "PluginRegistry.removePlugins(plugin) parameter must be instanceof " + Plugin.class.getName() );
}
getPlugins().remove( plugin );
} //-- void removePlugin( Plugin )
/**
* Set specifies whether the user should be prompted to update
* plugins.
*
* @param autoUpdate
*/
public void setAutoUpdate( String autoUpdate )
{
this.autoUpdate = autoUpdate;
} //-- void setAutoUpdate( String )
/**
* Set whether to resolve plugin versions using LATEST
* metadata.
*
* @param checkLatest
*/
public void setCheckLatest( String checkLatest )
{
this.checkLatest = checkLatest;
} //-- void setCheckLatest( String )
/**
* Set an encoding used for reading/writing the model.
*
* @param modelEncoding
*/
public void setModelEncoding( String modelEncoding )
{
this.modelEncoding = modelEncoding;
} //-- void setModelEncoding( String )
/**
* Set specified plugin update policy information.
*
* @param plugins
*/
public void setPlugins( java.util.List plugins )
{
this.plugins = plugins;
} //-- void setPlugins( java.util.List )
/**
* Set specifies how often to check for plugin updates. Valid
* values are: never, always, interval:XXX.
* For the interval specification, XXX denotes a
* terse interval specification, such as 4h.
* Where h=hours, m=minutes, d=days, w=weeks. The
* interval period should be specified in descending
* order of granularity, like this: '[n]w [n]d [n]h
* [n]m'. Any omitted level of granularity will be
* assumed to be a zero value.
*
* @param updateInterval
*/
public void setUpdateInterval( String updateInterval )
{
this.updateInterval = updateInterval;
} //-- void setUpdateInterval( String )
private java.util.Map pluginsByKey;
public java.util.Map getPluginsByKey()
{
if ( pluginsByKey == null )
{
pluginsByKey = new java.util.HashMap();
for ( java.util.Iterator it = getPlugins().iterator(); it.hasNext(); )
{
Plugin plugin = (Plugin) it.next();
pluginsByKey.put( plugin.getKey(), plugin );
}
}
return pluginsByKey;
}
public void flushPluginsByKey()
{
this.pluginsByKey = null;
}
private RuntimeInfo runtimeInfo;
public void setRuntimeInfo( RuntimeInfo runtimeInfo )
{
this.runtimeInfo = runtimeInfo;
}
public RuntimeInfo getRuntimeInfo()
{
return runtimeInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy